\n\u003C/template>\n\n\u003Cscript setup>\nconst props = defineProps({\n name: {\n type: String,\n required: true,\n },\n strokeColor: {\n type: String,\n default: \"\",\n },\n fillColor: {\n type: String,\n default: \"\",\n },\n});\n\nconst getCustomized = (content) => {\n if (props.strokeColor) {\n content = content.replaceAll(\n /stroke=\"[^\"]*\"/g,\n `stroke=\"${props.strokeColor}\"`\n );\n }\n if (props.fillColor) {\n content = content.replaceAll(\n /fill=\"[^\"]*\"/g,\n `fill=\"${props.fillColor}\"`\n );\n }\n return content;\n};\n\u003C/script>\n\n```\n\nand here's how I use it\n``` vue\n\u003CKBIcon name=\"kb:burger\" strokeColor=\"red\" />\n\u003CKBIcon name=\"kb:burger\" strokeColor=\"blue\" />\n```\n\nEach KBIcon instance should render the icon with its respective strokeColor properties:\nThe first icon should have a red stroke.\nThe second icon should have a blue stroke.\n\nhowever, the actual behavior is both icons render with the same red strokeColor, indicating that the customize function result is being cached and reused. Any solution for this? I just need to set different colors for the same icon. I tried set style color but it also have the same cache result.\n\nhere's my svg I use (/assets/icons/burger.svg):\n``` javascript\n\u003Csvg width=\"24\" height=\"24\" viewBox=\"0 0 24 24\" fill=\"none\" xmlns=\"http://www.w3.org/2000/svg\">\n\u003Cpath d=\"M5 6.78137H19\" stroke=\"#9EA2AE\" stroke-width=\"1.5\" stroke-linecap=\"round\" stroke-linejoin=\"round\"/>\n\u003Cpath d=\"M5 11.6251H19\" stroke=\"#9EA2AE\" stroke-width=\"1.5\" stroke-linecap=\"round\" stroke-linejoin=\"round\"/>\n\u003Cpath d=\"M5 16.4687H19\" stroke=\"#9EA2AE\" stroke-width=\"1.5\" stroke-linecap=\"round\" stroke-linejoin=\"round\"/>\n\u003C/svg>\n```",[],332,"nuxt","icon","closed","local nuxt icon caching issue","2025-01-06T07:08:29Z","https://github.com/nuxt/icon/issues/332",0.44956288,{"labels":1993,"number":1994,"owner":1985,"repository":1985,"state":1987,"title":1995,"updated_at":1996,"url":1997,"score":1998},[],12025,"Error when attempting to use Tailwind CSS with Nuxt 3","2023-01-19T15:54:17Z","https://github.com/nuxt/nuxt/issues/12025",0.6975882,{"description":2000,"labels":2001,"number":2002,"owner":1985,"repository":1985,"state":1987,"title":2003,"updated_at":2004,"url":2005,"score":2006},"\r\nhttps://github.com/nuxt/nuxt/assets/47276871/8a502cef-7dbd-4dc6-bbd2-5327fde643a4\r\n\r\n\u003Cimg width=\"372\" alt=\"image\" src=\"https://github.com/nuxt/nuxt/assets/47276871/619327f3-f7d5-4559-880c-5834974f75d0\">\r\n\u003Cimg width=\"398\" alt=\"image\" src=\"https://github.com/nuxt/nuxt/assets/47276871/d7fc548a-d1ac-4077-9b81-0c0bd86f7e9b\">\r\n\r\nHere is a code base, hope can help me to see the problem https://github.com/Lucas-lululu/test-code.git",[],22718,"I have a doubt. When element-plus and tailwindcss exist in nuxt3 at the same time, the color of the element-plus component will appear in a post-rendering process. I don’t know which step is the problem.","2023-08-30T16:31:04Z","https://github.com/nuxt/nuxt/issues/22718",0.699377,{"description":2008,"labels":2009,"number":2025,"owner":1985,"repository":1985,"state":1987,"title":2026,"updated_at":2027,"url":2028,"score":2029},"I used nuxt 3 for a project, and then during the deployment, when I run the `npx nuxi generate`, none of the images in the asset folder would display as well as the script codes, though they worked fine locally. I tried the `npm run build` and this was quite better. Just that swiper runs the first slide and then that is it. the rest of the images wouldn't show. Fontawesome never works when I run the two commands. When I tried deploying to Netlify, I get the first results. When i switched to vercel, when it runs the `npm run build` command, I get the first result; as in no images in the assets show, the codes in the script tags doesn't work. I tried to change the command to `npx nuxi generate`and i get the same results. In the browser, `Loading module from “https://aklowadrumz-ilx4-jm01tvghs-aklowa-drumzs-projects.vercel.app/aklowadrumz/assets/CAUEt-de.js” was blocked because of a disallowed MIME type (“text/html”)`.\r\n\r\nHere is the configuration that I did in the nuxt.config.ts\r\n\r\n`import { Static } from \"vue\";\r\n\r\n// https://nuxt.com/docs/api/configuration/nuxt-config\r\nexport default defineNuxtConfig({\r\n devtools: { enabled: process.env.NODE_ENV !== 'production' },\r\n ssr: true,\r\n target: Static,\r\n nitro: {\r\n preset: 'vercel',\r\n prerender: {\r\n routes: ['/', '/blog/history', '/blog/history', '/blog/dance', '/blog/acrobatics']\r\n }\r\n },\r\n app: {\r\n baseURL: '/aklowadrumz/', // baseURL: '/\u003Crepository>/'\r\n buildAssetsDir: 'assets',\r\n head: {\r\n title: 'Aklowa Drum Band',\r\n meta: [\r\n { name: 'viewport', content: 'width=device-width, initial-scale=1' }\r\n ],\r\n script: [{ src: 'https://identity.netlify.com/v1/netlify-identity-widget.js', defer: true }],\r\n }\r\n},\r\nbuild: {\r\n assetsDir: 'assets',\r\ntranspile: ['@fortawesome/vue-fontawesome'],\r\nchunkSizeWarningLimit: 100000,\r\ncssMinify: {\r\n preset: 'default',\r\n},\r\n},\r\npostcss: {\r\n plugins: {\r\n tailwindcss: {},\r\n autoprefixer: {},\r\n },\r\n},\r\npublicRuntimeConfig: {\r\n static: {\r\n publicPath: \"/\" // or a path relative to your domain (e.g., \"/my-app/\")\r\n }\r\n},\r\n// publicPath: process.env.npm_lifecycle_event === 'generate' ? '/pwa/' : '/_nuxt/',\r\n\r\noptimization: {\r\n splitChunks: {\r\n name: true,\r\n },\r\n runtimeChunk: true,\r\n},\r\n\r\nsplitChunks: {\r\n layouts: true,\r\n pages: true,\r\n commons: true,\r\n},\r\n\r\n modules: [\r\n '@hypernym/nuxt-gsap',\r\n '@nuxtjs/tailwindcss',\r\n 'nuxt-purgecss',\r\n '@nuxtjs/eslint-module',\r\n 'nuxt-swiper',\r\n \"@nuxt/image\"\r\n ],\r\n tailwindcss: {\r\n cssPath: ['~/assets/css/tailwind.css', { injectPosition: \"first\" }],\r\n configPath: 'tailwind.config',\r\n exposeConfig: {\r\n level: 2\r\n },\r\n config: {},\r\n viewer: true,\r\n },\r\n css: [\r\n '@fortawesome/fontawesome-svg-core/styles.css',\r\n '~/assets/css/tailwind.css'\r\n ],\r\n})`\r\n\r\nand here is the package.json\r\n`{\r\n \"name\": \"nuxt-app\",\r\n \"private\": true,\r\n \"type\": \"module\",\r\n \"scripts\": {\r\n \"build\": \"nuxt build\",\r\n \"dev\": \"nuxt dev\",\r\n \"generate\": \"nuxt generate\",\r\n \"preview\": \"nuxt preview\",\r\n \"postinstall\": \"nuxt prepare\"\r\n },\r\n \"dependencies\": {\r\n \"@fortawesome/fontawesome-svg-core\": \"^6.5.2\",\r\n \"@fortawesome/free-brands-svg-icons\": \"^6.5.2\",\r\n \"@fortawesome/free-solid-svg-icons\": \"^6.5.2\",\r\n \"@fortawesome/vue-fontawesome\": \"^3.0.6\",\r\n \"@hypernym/nuxt-gsap\": \"^2.4.2\",\r\n \"@nuxt/image\": \"^1.5.0\",\r\n \"@nuxtjs/pwa\": \"^3.3.5\",\r\n \"@tailwindcss/typography\": \"^0.5.12\",\r\n \"gh-pages\": \"^6.1.1\",\r\n \"gsap\": \"^3.12.5\",\r\n \"nuxt\": \"^3.11.1\",\r\n \"nuxt-swiper\": \"^1.2.2\",\r\n \"swiper\": \"^11.1.0\",\r\n \"vue\": \"^3.4.21\",\r\n \"vue-router\": \"^4.3.0\"\r\n },\r\n \"devDependencies\": {\r\n \"@nuxtjs/eslint-module\": \"^4.1.0\",\r\n \"@nuxtjs/tailwindcss\": \"^6.11.4\",\r\n \"autoprefixer\": \"^10.4.19\",\r\n \"eslint\": \"^8.57.0\",\r\n \"eslint-plugin-vue\": \"^9.24.0\",\r\n \"nuxt-purgecss\": \"^2.0.0\",\r\n \"postcss\": \"^8.4.38\",\r\n \"sass\": \"^1.72.0\",\r\n \"tailwindcss\": \"^3.4.3\"\r\n }\r\n}\r\n`\r\n",[2010,2013,2016,2019,2022],{"name":2011,"color":2012},"3.x","29bc7f",{"name":2014,"color":2015},"pending triage","E99695",{"name":2017,"color":2018},"nitro","bfd4f2",{"name":2020,"color":2021},"needs reproduction","FBCA04",{"name":2023,"color":2024},"closed-by-bot","ededed",26673,"Deployment problems with Nuxt 3 - Tailwind - Netlify or vercel","2024-05-15T01:50:09Z","https://github.com/nuxt/nuxt/issues/26673",0.70065254,{"description":2031,"labels":2032,"number":2036,"owner":1985,"repository":1985,"state":1987,"title":2037,"updated_at":2038,"url":2039,"score":2040},"### Environment\n\n- Operating System: `Darwin`\r\n- Node Version: `v20.11.1`\r\n- Nuxt Version: `3.11.2`\r\n- CLI Version: `3.11.1`\r\n- Nitro Version: `2.9.6`\r\n- Package Manager: `yarn@4.2.2`\r\n- Builder: `-`\r\n- User Config: `devtools`, `debug`, `hooks`, `modules`, `plugins`, `nitro`, `runtimeConfig`, `dayjs`, `postcss`, `app`, `css`, `apollo`, `carousel`, `tour`, `vue`, `routeRules`\r\n- Runtime Modules: `@nuxtjs/tailwindcss@6.12.0`, `@nuxtjs/device@3.1.1`, `@nuxt/image@1.4.0`, `@pinia/nuxt@0.5.1`, `@pinia-plugin-persistedstate/nuxt@1.2.0`, `dayjs-nuxt@2.1.9`, `vue3-carousel-nuxt@1.1.1`, `@nuxtjs/apollo@5.0.0-alpha.14`, `nuxt-tour@0.0.9`, `@nuxtjs/i18n@8.3.1`\r\n- Build Modules: `-`\r\n\n\n### Reproduction\n\nCannot reproduce the issue either on new repo or stackblitz\r\n\r\nUsing \r\n\r\n \"nuxt\": \"^3.11.2\",\r\n \"@nuxtjs/tailwindcss\": \"^6.12.0\",\n\n### Describe the bug\n\nIn the below image in nuxt 3 bg-transparent is added at last but it is overridden by bg-yocket-neutral-100\r\n\r\n\u003Cimg width=\"647\" alt=\"Screenshot 2024-05-28 at 5 46 06 PM\" src=\"https://github.com/nuxt/nuxt/assets/45727492/081685a9-7c97-4f4e-8449-e3f0bfbc0719\">\r\n\r\nIn nuxt 2 the behaviour was opposite\r\n\u003Cimg width=\"1115\" alt=\"Screenshot 2024-05-28 at 5 52 22 PM\" src=\"https://github.com/nuxt/nuxt/assets/45727492/f4d6d5f5-d323-4312-bb37-46a4fb52f776\">\r\n\r\n\r\nThis same thing is happening throughout the project\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2033,2034,2035],{"name":2011,"color":2012},{"name":2014,"color":2015},{"name":2020,"color":2021},27385,"Tailwindcss added at last is not applied","2024-05-30T05:43:05Z","https://github.com/nuxt/nuxt/issues/27385",0.70086807,{"description":2042,"labels":2043,"number":2049,"owner":1985,"repository":1985,"state":1987,"title":2050,"updated_at":2051,"url":2052,"score":2053},"### Environment\n\nnode: v16.15.1\r\nnuxt: ^3.2.0\r\ntailwindcss: ^6.2.0\n\n### Reproduction\n\nhttps://stackblitz.com/edit/github-ky7itr\n\n### Describe the bug\n\nHello team,I want to use the style of tailwindcss: https://tailwindcss.com/docs/gradient-color-stops\r\n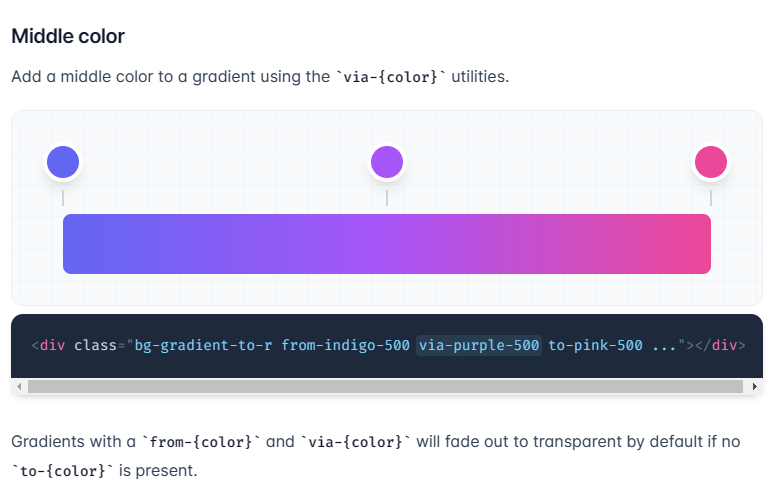\r\nso I wrote this:\r\n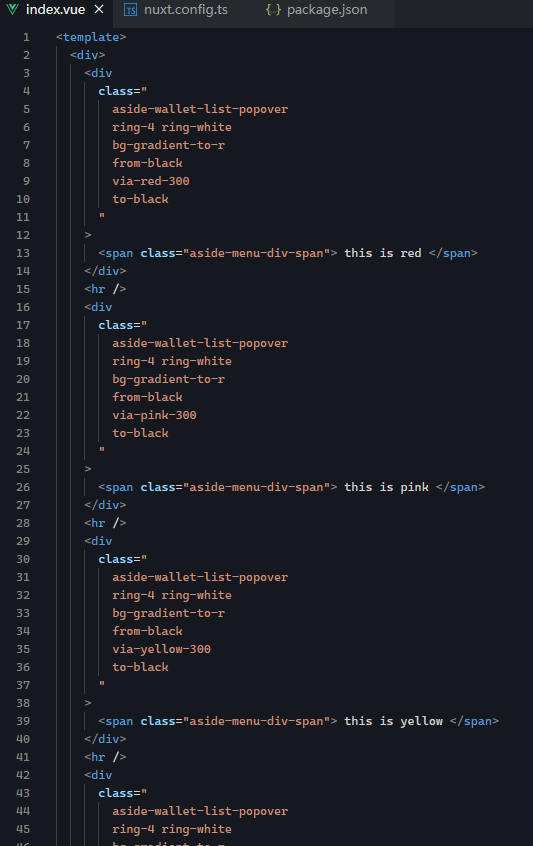\r\nresult:\r\n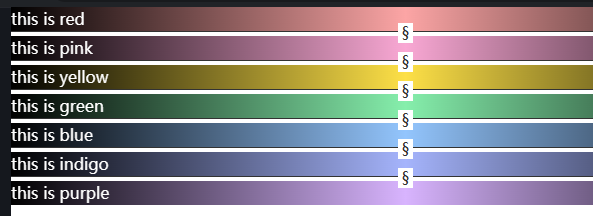\r\nIt's right,now I use dynamic class:\r\n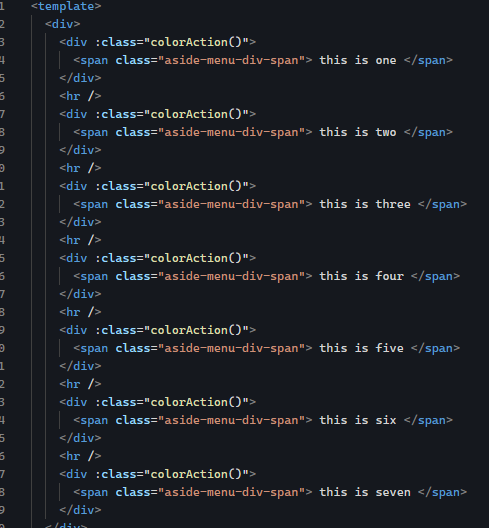\r\nreuslt:\r\n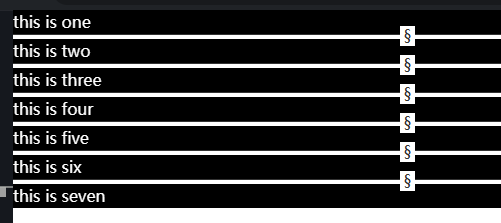\r\n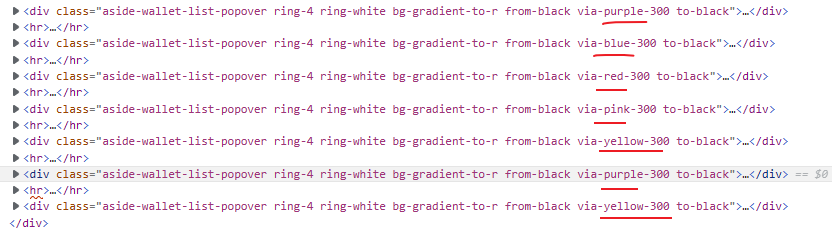\r\nIt can be seen from the console that the style is indeed written into the class,so what did I wrong?If you can see right style,please \r\nrestart the project,thanks.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2044,2045,2046],{"name":2011,"color":2012},{"name":2014,"color":2015},{"name":2047,"color":2048},"⛔️ can be closed","484893",19013,"Nuxt3 cannot use tailwindcss in dynamic class","2023-02-14T08:39:44Z","https://github.com/nuxt/nuxt/issues/19013",0.7055644,{"labels":2055,"number":2060,"owner":1985,"repository":1985,"state":1987,"title":1995,"updated_at":2061,"url":2062,"score":2063},[2056,2057],{"name":2011,"color":2012},{"name":2058,"color":2059},"bug","d73a4a",11937,"2023-01-19T16:08:26Z","https://github.com/nuxt/nuxt/issues/11937",0.7062343,{"description":2065,"labels":2066,"number":2069,"owner":1985,"repository":1985,"state":1987,"title":2070,"updated_at":2071,"url":2072,"score":2073},"I develop a website that runs with Nuxt 3.13.2, DaisyUI and TailwindCSS with bunch of nuxt modules, kindly please check the package.json details below:\n\n```\n{\n \"name\": \"the-website\",\n \"private\": true,\n \"type\": \"module\",\n \"scripts\": {\n \"build\": \"nuxt build\",\n \"dev\": \"nuxt dev\",\n \"generate\": \"nuxt generate --modern\",\n \"preview\": \"nuxt preview\",\n \"postinstall\": \"nuxt prepare\"\n },\n \"dependencies\": {\n \"@nuxt/image\": \"^1.8.1\",\n \"@nuxtjs/google-fonts\": \"^3.2.0\",\n \"@nuxtjs/i18n\": \"^8.3.3\",\n \"@nuxtjs/seo\": \"^2.0.0-rc.15\",\n \"@nuxtjs/tailwindcss\": \"^6.12.0\",\n \"@vee-validate/nuxt\": \"^4.13.2\",\n \"@vee-validate/yup\": \"^4.13.2\",\n \"@vueuse/core\": \"^11.1.0\",\n \"@vueuse/nuxt\": \"^11.1.0\",\n \"nuxt\": \"^3.13.2\",\n \"nuxt-booster\": \"^3.1.5\",\n \"nuxt-icons\": \"^3.2.1\",\n \"nuxt-mail\": \"^5.0.1\",\n \"nuxt-marquee\": \"^1.0.4\",\n \"nuxt-snackbar\": \"^1.0.4\",\n \"sass\": \"^1.77.5\",\n \"vue\": \"^3.4.27\",\n \"vue-countup-v3\": \"^1.4.2\",\n \"vue-router\": \"^4.3.3\",\n \"yup\": \"^1.4.0\"\n },\n \"devDependencies\": {\n \"@egoist/tailwindcss-icons\": \"^1.8.1\",\n \"@iconify/json\": \"^2.2.218\",\n \"@tailwindcss/forms\": \"^0.5.7\",\n \"@tailwindcss/typography\": \"^0.5.13\",\n \"daisyui\": \"^4.12.2\",\n \"nuxt-aos\": \"^1.2.5\"\n },\n \"packageManager\": \"yarn@4.4.1\"\n}\n```\n\nnuxt.config.ts:\n```\n// https://nuxt.com/docs/api/configuration/nuxt-config\nexport default defineNuxtConfig({\n $production: {\n devtools: { enabled: false },\n },\n\n ssr: true,\n devtools: { enabled: true },\n\n app: {\n head: {\n titleTemplate: '%s %separator WSoft Labs',\n meta: [\n {\n \"name\": \"viewport\",\n \"content\": \"width=device-width, initial-scale=1\"\n },\n {\n \"charset\": \"utf-8\"\n }\n ],\n link: [\n {\n rel: 'icon',\n href: '/favicon.ico'\n }\n ]\n }\n },\n\n runtimeConfig: {\n // apiSecret: '', // can be overridden by NUXT_API_SECRET environment variable\n public: {\n baseURL: process.env.NUXT_BASE_URL ?? 'http://localhost:3000',\n apiBase: process.env.NUXT_API_BASE ?? 'http://localhost:8000/api', // can be overridden by NUXT_PUBLIC_API_BASE environment variable\n apiUrl: process.env.NUXT_API_URL ?? 'http://localhost:8000',\n }\n },\n\n imports: {\n dirs: [\n // Scan top-level modules\n 'composables',\n // ... or scan modules nested one level deep with a specific name and file extension\n 'composables/*/index.{ts,js,mjs,mts}',\n // ... or scan all modules within given directory\n 'composables/**',\n 'utils/**'\n ]\n },\n\n components: [\n {\n path: './components',\n extensions: ['.vue'],\n pathPrefix: false\n }\n ],\n\n modules: [\n '@nuxtjs/tailwindcss',\n 'nuxt-snackbar',\n 'nuxt-icons',\n 'nuxt-marquee',\n '@vee-validate/nuxt',\n 'nuxt-aos',\n '@nuxtjs/i18n',\n '@nuxtjs/seo',\n 'nuxt-booster',\n '@nuxt/image',\n '@nuxtjs/google-fonts',\n '@vueuse/nuxt',\n [\n 'nuxt-mail', {\n message: {\n to: process.env.NUXT_MAIL_TO,\n },\n smtp: {\n host: process.env.NUXT_MAIL_SMTP,\n port: process.env.NUXT_MAIL_PORT ?? 25,\n secure: process.env.NUXT_MAIL_SMTP_SECURE ?? false,\n auth: {\n user: process.env.NUXT_MAIL_USER,\n pass: process.env.NUXT_MAIL_PASSWORD,\n }\n },\n }\n ],\n ],\n\n build: {\n transpile: ['vue-countup-v3'],\n },\n\n tailwindcss: {\n cssPath: [\n '~/assets/scss/main.scss',\n {\n injectPosition: 'first'\n }\n ],\n configPath: 'tailwind.config',\n exposeConfig: {\n level: 2\n },\n config: {},\n viewer: true,\n editorSupport: true\n },\n\n css: [\n \"@/assets/scss/main.scss\",\n ],\n\n snackbar: {\n top: true,\n right: true,\n duration: 5000\n },\n\n veeValidate: {\n autoImports: true,\n componentNames: {\n Form: 'VeeForm',\n Field: 'VeeField',\n FieldArray: 'VeeFieldArray',\n ErrorMessage: 'VeeErrorMessage',\n },\n },\n\n i18n: {\n locales: [\n {\n code: 'en',\n language: 'en-US',\n name: 'English',\n file: 'en-US.js'\n },\n {\n code: 'kr',\n language: 'kr-KR',\n name: 'Korean',\n file: 'kr-KR.js'\n },\n ],\n defaultLocale: 'kr',\n lazy: true,\n langDir: 'lang',\n compilation: {\n strictMessage: false\n },\n detectBrowserLanguage: {\n useCookie: true,\n cookieKey: 'wsoftlabs_i18n_redirected',\n alwaysRedirect: true,\n fallbackLocale: 'en',\n },\n },\n\n booster: {\n detection: {\n performance: true,\n browserSupport: true,\n battery: true,\n },\n performanceMetrics: {\n timing: {\n fcp: 800,\n dcl: 1200\n }\n }\n },\n\n image: {\n screens: {\n default: 320,\n xxs: 480,\n xs: 576,\n sm: 768,\n md: 996,\n lg: 1200,\n xl: 1367,\n xxl: 1600,\n '4k': 1921\n },\n provider: \"ipx\",\n dir: \"assets\"\n },\n\n googleFonts: {\n families: {\n Poppins: {\n wght: [100, 200, 300, 400, 500, 600, 700, 800, 900],\n ital: [100, 200, 300, 400, 500, 600, 700, 800, 900],\n }\n },\n display: 'swap',\n prefetch: false,\n preconnect: false,\n preload: false,\n download: true,\n base64: true,\n overwriting: true,\n },\n\n compatibilityDate: '2024-10-14'\n})\n```\n\nmy issue is when I try to run it via dev server via `yarn dev`, it will not show a warning for hydration mismatches:\n\n[![running in yarn dev][1]][1]\n\nbut when I do run `yarn generate && yarn preview`, it does show the said error:\n\n[![running in yarn generate][2]][2]\n\nwith this current `app.vue` codebase:\n\n[![prev. ClientOnly placement][3]][3]\n\n\nNow, I did a workaround and trying to tweak `ClientOnly` component to move the wrap and include the `NuxtLayout` component\n\n[![curr. ClientOnly placement][4]][4]\n\nafter then, I re-run `yarn generate && yarn preview`, the hydration error is gone but my images is now not working anymore.\n\n[![enter image description here][5]][5]\n\nbut when I run the workaround with `yarn dev`, it has the same result as the first screenshot (no hydration mismatches warnings/errors).\n\nI'm running a module called `nuxt-image` to optimize the images on load when uploaded to the server and `nuxt-booster` to boost the SEO-related things of the app.\n\nhere's my [repo][6] for further details and do some workaround about it, please do leave some comments/suggestions/guidance/help with the repo as a form of help will be much appreciated in order to improve the overall project and most importantly, myself.\n\n\n [1]: https://i.sstatic.net/AzYGTJ8J.png\n [2]: https://i.sstatic.net/JzzVXt2C.png\n [3]: https://i.sstatic.net/bZJL5luU.png\n [4]: https://i.sstatic.net/TyT166Jj.png\n [5]: https://i.sstatic.net/kZ6XfSmb.png\n [6]: https://github.com/noelc10/wsl-website",[2067,2068],{"name":2014,"color":2015},{"name":2020,"color":2021},29704,"Nuxt 3.13.2: nuxt generate build hydration completed but contains mismatches errors","2025-03-24T23:15:34Z","https://github.com/nuxt/nuxt/issues/29704",0.70631194,{"labels":2075,"number":2077,"owner":1985,"repository":1985,"state":1987,"title":2078,"updated_at":2079,"url":2080,"score":2081},[2076],{"name":2011,"color":2012},11875,"TailwindCSS only works on client-side","2023-01-19T15:48:25Z","https://github.com/nuxt/nuxt/issues/11875",0.70666075,{"description":2083,"labels":2084,"number":2087,"owner":1985,"repository":2088,"state":1987,"title":2089,"updated_at":2090,"url":2091,"score":2092},"\u003Cimg width=\"825\" alt=\"SCR-20240304-tzmi\" src=\"https://github.com/nuxt/fonts/assets/133459587/c6d01cda-6d0c-4151-870a-d2701594d55d\">\r\n",[2085],{"name":2020,"color":2086},"C94E53",29,"fonts","An error occurred from the font module of @nuxtjs to using nuxt/fonts","2024-03-05T05:13:39Z","https://github.com/nuxt/fonts/issues/29",0.7083403,["Reactive",2094],{},["Set"],["ShallowReactive",2097],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"y6Ccp2G9iVSheXdJ_QM-2HfndFFF35pWQcEQ3mZ03mY":-1},"/nuxt/fonts/332"]