\r\n Loading\r\n \u003C/div>\r\n \u003C/template>\r\n \u003Cdiv class=\"content\" ref=\"someRef\">\r\n This text should NOT be large!\r\n Remove the \u003Cpre>ref=\"someRef\"\u003C/pre> from the parent and it works\r\n \u003C/div>\r\n \u003C/ClientOnly>\r\n\u003C/template>\r\n```\r\n\r\n**Expected behaviour when loading the page:**\r\nA big loading spinner, and, when everything is done loading, some normal text, like this:\r\n\r\n\r\n**Instead,** you get this:\r\n\r\n\r\nWhen you remove the `ref=\"someRef\"` from the content div, it works as expected",[],"nuxt","icon","open","Unexpected behaviour when using an Icon within ClientOnly fallback","2023-12-18T12:08:48Z","https://github.com/nuxt/icon/issues/129",0.74387914,{"description":3026,"labels":3027,"number":3034,"owner":3018,"repository":3035,"state":3020,"title":3036,"updated_at":3037,"url":3038,"score":3039},"### Description\n\nThis is a follow up to this: https://github.com/nuxt/ui/issues/4498\n\nI'm using NuxtUi and not the NuxtJS framework (inertiajs), and I would to do something like this:\n\n```js\n{\n icon: {\n provider: 'server', // \u003C-- own server here\n customCollections: [\n {\n prefix: 'my-icon',\n dir: './assets/my-icons'\n },\n ],\n },\n}\n```\n\nThe original bug report suggests workaround that aren't that plug-and-play. For example, it may be possible to use an Laravel instance that pushes the icon-set to S3, and nuxt-ui using the S3-instance (e.g. https://s3.example.org/icons/) as a base for the icon collection(s).\n\n### Additional context\n\n_No response_",[3028,3031],{"name":3029,"color":3030},"enhancement","a2eeef",{"name":3032,"color":3033},"triage","ffffff",4541,"ui","Custom Icon server path","2025-07-16T14:22:40Z","https://github.com/nuxt/ui/issues/4541",0.7439982,{"description":3041,"labels":3042,"number":3050,"owner":3018,"repository":3035,"state":3020,"title":3051,"updated_at":3052,"url":3053,"score":3054},"### Environment\n\n- Operating System: \"linux/ubuntu 24.04\"\n- Node Version: \"22.15.1\"\n- Nuxt Version: \"3.17.6\"\n- Package Manager: \"10.9.2\"\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\n3.17.6\n\n### Reproduction\n\nhttps://github.com/pbartyla/nuxt_color_mode_bug\n\n### Description\n\nWhen using [useColorMode](https://ui.nuxt.com/getting-started/color-mode/nuxt), if a user manually selects a theme mode (light or dark) that differs from their system preference, and then refreshes the page, the stored mode is correctly applied, and isDark returns the expected value immediately.\n\nHowever, UI elements that rely on isDark for conditional rendering (e.g., toggling an icon) do not update on initial load. The component renders with an incorrect icon, despite isDark being correct.\n\n1. Visit a Nuxt UI page using useColorMode and a toggle button like:\n\n`\u003CUButton :icon=\"isDark ? 'i-lucide-moon' : 'i-lucide-sun'\" color=\"neutral\" variant=\"ghost\"`\n\n2. Manually toggle to \"dark\" mode (while system is set to \"light\").\n\n3. Refresh the page.\n\n4. isDark is true, the theme is applied as dark — but the icon still shows as \"moon\" (suggesting light mode).\n\nExpected behavior:\n\nSince isDark is correct immediately, the icon should render correctly ('sun' in dark mode). It seems like the initial render uses stale/default data or runs before the reactive state is fully respected by the component.\n\n### Additional context\n\nManually toggling the mode after page load causes the icon to update correctly.\n\nLikely related to hydration or timing of when isDark is reactive in relation to rendering the component.\n\nVideo recording of the issue is available for reference — showing the incorrect icon on initial page load despite the correct theme and isDark value.\n\nhttps://github.com/user-attachments/assets/8c2bac86-f7f9-4bc0-8e11-64d107c60a18\n\n\n### Logs\n\n```shell-script\n\n```",[3043,3046,3049],{"name":3044,"color":3045},"bug","d73a4a",{"name":3047,"color":3048},"v3","49DCB8",{"name":3032,"color":3033},4459,"useColorMode does not respect storage value on initial load (icon mismatch)","2025-07-04T08:57:31Z","https://github.com/nuxt/ui/issues/4459",0.745758,{"description":3056,"labels":3057,"number":3058,"owner":3018,"repository":3019,"state":3020,"title":3059,"updated_at":3060,"url":3061,"score":3062},"I'm using only custom icon collections via customCollections, but when clientBundle.scan is enabled, the build includes thousands of icons from unrelated collections in the final bundle (icons.json). This happens even though no other icon sets are used anywhere in the project. I expect the bundle to include only the icons I actually use from my defined collections.\n\n\n\nThis is the request that happens at the start of the app, loading nearly 8,000 icons, while my custom icon set only has 60 icons. How can I configure the bundler or Nuxt Icon so that only these 60 icons are included in the final bundle instead of the entire collection?",[],410,"@nuxt/icon includes unrelated icons in icons.json when only using customCollections with scan: true","2025-06-30T17:38:24Z","https://github.com/nuxt/icon/issues/410",0.74695957,{"description":3064,"labels":3065,"number":3075,"owner":3018,"repository":3018,"state":3020,"title":3076,"updated_at":3077,"url":3078,"score":3079},"## Environment\r\n\r\n```\r\n- Operating System: `MacOs`\r\n- Node Version: `v19.3.0`\r\n- Nuxt Version: `3.0.0`\r\n- Nitro Version: `1.0.0`\r\n- Package Manager: `yarn@1.22.19`\r\n- Builder: `vite`\r\n- User Config: `-`\r\n- Runtime Modules: `-`\r\n- Build Modules: `-`\r\n```\r\n\r\n## Describe the issue\r\n\r\nI am using dynamic import to import icons conditionally but I don't want to prefetch them.\r\nThis might stack up to many unused icons because it prefetches all icons from the `./icons` folder.\r\n\r\nWhen I want to load just Twitter icon, everything is imported.\r\n\r\n```\r\n\u003CInlineIcon name=\"twitter\" />\r\n```\r\n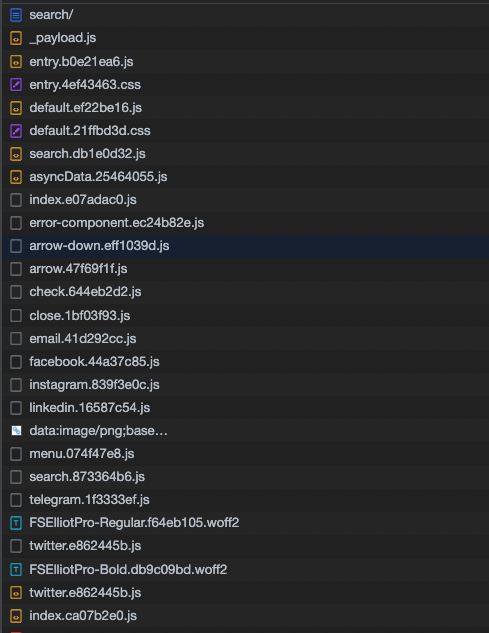\r\n\r\n## Code\r\n\r\n```\r\n\u003Ctemplate>\r\n \u003CIcon />\r\n\u003C/tempalte>\r\n\r\n\u003Cscript setup lang=\"ts\">\r\nconst props = defineProps\u003C{name: string}>()\r\n\r\nconst Icon = computed(() => defineAsyncComponent(() => import(`../icons/${props.name}.svg?component`)));\r\n\u003C/script>\r\n```\r\n\r\n\r\n\r\n",[3066,3069,3072],{"name":3067,"color":3068},"workaround available","11376d",{"name":3070,"color":3071},"❗ p4-important","D93F0B",{"name":3073,"color":3074},"performance","E84B77",18376,"Disable `prefetch` for dynamic imports","2025-03-16T23:31:44Z","https://github.com/nuxt/nuxt/issues/18376",0.7614594,{"description":3081,"labels":3082,"number":3083,"owner":3018,"repository":3019,"state":3084,"title":3085,"updated_at":3086,"url":3087,"score":3088},"### Motivation\r\n\r\nAs is, iconify is great for free, public icon sets. For commercial purposes, you may want to use commercially licensed icon pack - the most popular example being [Font Awesome Pro](https://fontawesome.com/icons). Iconify [already provides](https://iconify.design/docs/libraries/tools/examples/import-fa-pro.html) a way of converting custom icon packs into `IconifyJSON` definition. However, Including the whole `IconifyJSON` in the app is costly, it can easily weigh over 100 kB (raw). Therefore, iconify by default uses API provider to deliver only the requested icon definitions on demand. It is currently not possible to easily host your own icon packs definitions.\r\n\r\n### Feature Request\r\nSince nuxt already hosts server routes, it would be great if the module could provide a streamlined method of hosting Iconify API provider. This way, the end-user could easily use their non-free icon packs benefiting from the on-demand architecture of iconifi's `loadIcon`.\r\n\r\nExample module configuration could look like this:\r\n```ts\r\ndefineAppConfig({\r\n nuxtIcon: {\r\n customCollections: [\r\n resolve('./assets/icons/collections/my-icons.json')\r\n ]\r\n }\r\n})\r\n```\r\n\r\nTo achieve this, the module would have to expose http API conforming to the [Iconify API standard](https://iconify.design/docs/api/icon-data.html#query). The API is very straight forward\r\n\r\nExample definition:\r\n```json\r\n{\r\n \"prefix\": \"my-icons\",\r\n \"lastModified\": 1691181322,\r\n \"icons\": {\r\n \"a\": { \"body\": \"\u003Cpath d=\\\"_path_a_\\\"/>\" },\r\n \"b\": { \"body\": \"\u003Cpath d=\\\"_path_b_\\\"/>\" },\r\n \"c\": { \"body\": \"\u003Cpath d=\\\"_path_c_\\\"/>\" }\r\n },\r\n \"width\": 24,\r\n \"height\": 24,\r\n}\r\n```\r\n\r\nExample API response to `GET /api/iconify-provider/my-icons.json?icons=a,c`\r\n```json\r\n{\r\n \"prefix\": \"my-icons\",\r\n \"icons\": {\r\n \"a\": { \"body\": \"\u003Cpath d=\\\"_path_a_\\\"/>\" },\r\n \"c\": { \"body\": \"\u003Cpath d=\\\"_path_c_\\\"/>\" }\r\n },\r\n \"width\": 24,\r\n \"height\": 24,\r\n}\r\n```\r\n\r\nModule would have to register the custom provider with iconify library:\r\n```ts\r\nimport { addAPIProvider } from '@iconify/vue'\r\naddAPIProvider('nuxt', { resources: ['/api/iconify-provider'] })\r\n```\r\n\r\nAnd the icons should be usable as per example:\r\n```html\r\n\u003Cicon name=\"@nuxt:my-icons:a\"/>\r\n\u003Cicon name=\"@nuxt:my-icons:c\"/>\r\n```",[],97,"closed","Feature Request: Built-in API provider for custom IconifyJSON","2024-10-25T16:58:25Z","https://github.com/nuxt/icon/issues/97",0.7309161,{"description":3090,"labels":3091,"number":3092,"owner":3018,"repository":3019,"state":3084,"title":3093,"updated_at":3094,"url":3095,"score":3096},"I have nuxt icon setup with a SSG app. I'm using lucide and the collection is installed as a dependency. I run nuxt generate, but at runtime, I see my app is calling out to the Iconify API. Is this intentional? If so, why? If not, what do I need to do to get the icons included in the SSG bundle.",[],396,"Nuxt Icon Using Inconify API w/ SSG","2025-05-18T21:03:54Z","https://github.com/nuxt/icon/issues/396",0.73265004,{"description":3098,"labels":3099,"number":3103,"owner":3018,"repository":3035,"state":3084,"title":3104,"updated_at":3105,"url":3106,"score":3107},"### Description\n\nHi there. We are moving our app to NuxtUI and we are loving it so far! Really appreciative of all your hard work on this amazing package. Our stack is NuxtUI+Inertia+Rails.\n\nI spent part of my weekend digging into UIcon and Iconify. Pardon my ignorance if I've missed something obvious, I'm new to this part of the ecosystem... I thought it would be useful to put some thoughts in here and collect what I've learned.\n\n1. UIcon/Iconify always load icons from the network (`api.iconify.design`)\n2. It's not really possible to use NuxtUI without relying heavily on UIcon/Iconify due to various icon attributes in the components.\n3. I know unplug-icons and Nuxt have some support for bundled icons, but they don't apply if you are using Nuxt UI standalone.\n\nSee #4167 and others\n\nWe want to bundle our icons to avoid a dependency on api.iconify.design. I ended up writing a script that scanned our app for icon names and builds a custom `icons.json` file. I put some custom icons in there too using `@iconify/tools`... `icons.json` can be loaded into iconify with `addCollection()`. In dev I also warn on missing icons via `setCustomIconsLoader()`.\n\nDoes that sound correct? Are there solutions I'm missing?? I'd be happy to contribute doc updates or whatever if that would be helpful.\n\nThanks again!\n\n### Additional context\n\n_No response_",[3100,3101,3102],{"name":3029,"color":3030},{"name":3047,"color":3048},{"name":3032,"color":3033},4481,"thoughts on UIcon/Iconify","2025-07-10T09:59:29Z","https://github.com/nuxt/ui/issues/4481",0.7378661,{"description":3109,"labels":3110,"number":3114,"owner":3018,"repository":3018,"state":3084,"title":3115,"updated_at":3116,"url":3117,"score":3118},"Hi Guys,\r\n\r\nI'm struggling to initialize my Vuex store with the account details of the logged in user from `localStorage`.\r\n\r\nI've been through as many examples as I can of Auth using Nuxt, and none of them demonstrate how on the client side to pull an `authToken` from `localStorage` to re-use with subsequent API requests when the user refreshes the page or navigates to the App \r\n\r\nDisclaimer: I'm not using Nuxt in SSR (this might affect your answer).\r\n\r\nWhat is annoying is that I _can_ actually load from `localStorage` and initialize my state but then it gets overwritten. I'll show you what I mean with this small code example:\r\n\r\n```\r\nbuildInitialState () {\r\n if (!process.server) {\r\n // Query the localStorage and return accessToken, refreshToken and account details\r\n return {accessToken: \u003Cvalid-string>, refreshToken: \u003Cvalid-string>, account: \u003Cvalid-json-blob>}\r\n } else {\r\n // Return \"empty map\", we use the string \"INVALID\" for demonstration purposes.\r\n return {accessToken: \"INVALID\", refreshToken: \"INVALID\", account: \"INVALID\"}\r\n }\r\n}\r\n\r\nexport const state = () => buildInitialState()\r\n```\r\n\r\nIf I put a breakpoint on `buildInitialState` I can see that I correctly initialize the state with the value of `localStorage`, i.e. I get the `accessToken` and `refreshToken`, etc.. back.\r\n\r\nAll seems well.\r\n\r\nBut *then* .....in another part of the program I'm using Axois to send requests, and I use an axios interceptor to decorate the request with the `accessToken`. To do this I have to stick it into a plugin to get access to the `store`.\r\n\r\nSomething like so:\r\n\r\n```\r\nexport default ({ app, store }) => {\r\n axios.interceptors.request.use((config) => {\r\n const accessToken = _.get(store.state, ['account', 'accessToken'])\r\n if (accessToken) {\r\n config.headers.common['x-auth-token'] = accessToken\r\n }\r\n return config\r\n }, (error) => Promise.reject(error))\r\n}\r\n```\r\n\r\nHere the `store` is closed over in the arrow function supplied to `axios` so when I go to send the request it sees if there is a valid `accessToken`, and if so then use it.\r\n\r\nBUT, and here's the kicker, when a request is made, I look at the `store.state.account.accessToken` and low and behold its been _reinitialized_ back to the value of \"INVALID\".\r\n\r\nWhat? Que?\r\n\r\nIt's almost like the store was *reinitialized* behind the scenes? Or somehow the `state` in the plugin is \"server side state\"?? because if I try and log `buildInitialState` I don't get any messages indicating that the path that produced a map with `INVALID` is being run.\r\n\r\nBasically, I don't thoroughly understand the initialization pathway Nuxt is taking here at all.\r\n\r\nIf any Nuxt masters could help me out understand this a bit more that would be great, it's turning into a bit of a show stopper for me.\r\n\r\nEssentially! All I want to be able to do is save the user so that when they refresh their page we can keep on running without forcing them to re-login again....I thought that would be simple.\r\n\r\nThanks and regards, Jason.\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1633\">#c1633\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[3111],{"name":3112,"color":3113},"2.x","d4c5f9",1818,"Cannot initialise Vuex store from localStorage on App initialization for Authentication","2023-01-18T15:54:46Z","https://github.com/nuxt/nuxt/issues/1818",0.7434562,{"description":3120,"labels":3121,"number":3122,"owner":3018,"repository":3019,"state":3084,"title":3123,"updated_at":3124,"url":3125,"score":3126},"\r\n\r\n\r\n\r\n",[],78,"Excuse me, when v-for performs list rendering, the icon name will appear. May I ask what may be the problem. Thanks","2023-05-10T09:55:26Z","https://github.com/nuxt/icon/issues/78",0.75081944,["Reactive",3128],{},["Set"],["ShallowReactive",3131],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$frZOjQQzqSpdLXU7usmFk9mV7JybSQyDK_bwhkIx0Wdk":-1},"/nuxt/icon/267"]