\r\n \u003C/div>\r\n\u003C/template>\r\n```\r\n\r\nand the test file looks like this:\r\n\r\n\r\n```\r\n// TestA.spec.ts\r\nimport { describe, it, expect } from 'vitest';\r\nimport { mount } from '@vue/test-utils';\r\nimport TestA from './TestA.vue';\r\n\r\ndescribe('Competition TestA', () => {\r\n it('renders component', async () => {\r\n const wrapper = mount(TestA);\r\n expect(wrapper.text()).toContain('Client component here...');\r\n });\r\n});\r\n\r\n```\r\n\r\nFinally, the client component:\r\n\r\n```\r\n// ClientComponent.client.vue\r\n\u003Ctemplate>\r\n \u003Cdiv>Client component here...\u003C/div>\r\n\u003C/template>\r\n```\r\n\r\nIf I remove the \".client\" from the component file name (to make it just ClientComponent.vue), then the tests will run and pass normally. However, there are some cases where I want to use \".client\" to make sure it's only rendered client-side.\r\n\r\n### Reproduction \r\nhttps://stackblitz.com/edit/github-ndby6m?file=components/TestA.spec.ts\r\n\r\nFailures can be seen there by running `pnpm run test`",[2031],{"name":2032,"color":2033},"vitest-environment","b60205",555,"closed","Tests fail when using .client components","2023-12-02T00:27:10Z","https://github.com/nuxt/test-utils/issues/555",0.45177928,{"description":2041,"labels":2042,"number":2046,"owner":1985,"repository":1999,"state":2035,"title":2047,"updated_at":2048,"url":2049,"score":2050},"### Environment\n\n- Operating System: `Linux`\r\n- Node Version: `v20.10.0`\r\n- Nuxt Version: `3.9.3`\r\n- CLI Version: `3.10.0`\r\n- Nitro Version: `2.8.1`\r\n- Package Manager: `npm@10.4.0`\r\n- Builder: `-`\r\n- User Config: `devtools`, `modules`, `devServer`, `srcDir`, `css`, `postcss`, `i18n`, `runtimeConfig`\r\n- Runtime Modules: `@nuxt/test-utils/module@3.10.0`, `@nuxtjs/i18n@8.0.0`\r\n- Build Modules: `-`\r\n\n\n### Reproduction\n\nhttps://stackblitz.com/edit/spy-use-fetch-is-not-working?file=src%2FuseLogin.nuxt.test.ts\n\n### Describe the bug\n\nI'm trying to create a spy for nuxt's composable useFetch and validate what is being passed as a parameter in the call, but for some reason a third random parameter is added\r\n\r\n\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2043],{"name":2044,"color":2045},"pending triage","5D08F5",749,"Mock adding third random parameter","2025-02-21T09:06:42Z","https://github.com/nuxt/test-utils/issues/749",0.6973224,{"description":2052,"labels":2053,"number":2059,"owner":1985,"repository":2023,"state":2035,"title":2060,"updated_at":2061,"url":2062,"score":2063},"### Environment\n\n System:\n OS: Linux 6.5 Ubuntu 23.10 23.10 (Mantic Minotaur)\n CPU: (8) x64 11th Gen Intel(R) Core(TM) i5-1135G7 @ 2.40GHz\n Memory: 44.48 GB / 62.57 GB\n Container: Yes\n Shell: 5.9 - /usr/bin/zsh\n Browsers:\n Chrome: 126.0.6478.126\n\n### Version\n\nv3.0.0-alpha.6\n\n### Reproduction\n\nhttps://ui3.nuxt.dev/components/select-menu\n\n### Description\n\n1. open docs\n2. open any select menu\n3. select any option\n4. tab to next field\n5. tab index is reset\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2054,2055,2056],{"name":1996,"color":1997},{"name":2020,"color":2021},{"name":2057,"color":2058},"reka-ui","56d799",2350,"[SelectMenu] Broken focus/tab index after selecting","2024-12-03T15:11:34Z","https://github.com/nuxt/ui/issues/2350",0.69984436,{"description":2065,"labels":2066,"number":1998,"owner":1985,"repository":1986,"state":2035,"title":2070,"updated_at":2071,"url":2072,"score":2003},"Blocked by #720 ",[2067],{"name":2068,"color":2069},"enhancement","1ad6ff","[Community] Nuxters filter to select community only","2022-07-25T13:34:54Z","https://github.com/nuxt/nuxt.com/issues/684",{"description":2074,"labels":2075,"number":2078,"owner":1985,"repository":1986,"state":2035,"title":2079,"updated_at":2080,"url":2081,"score":2082},"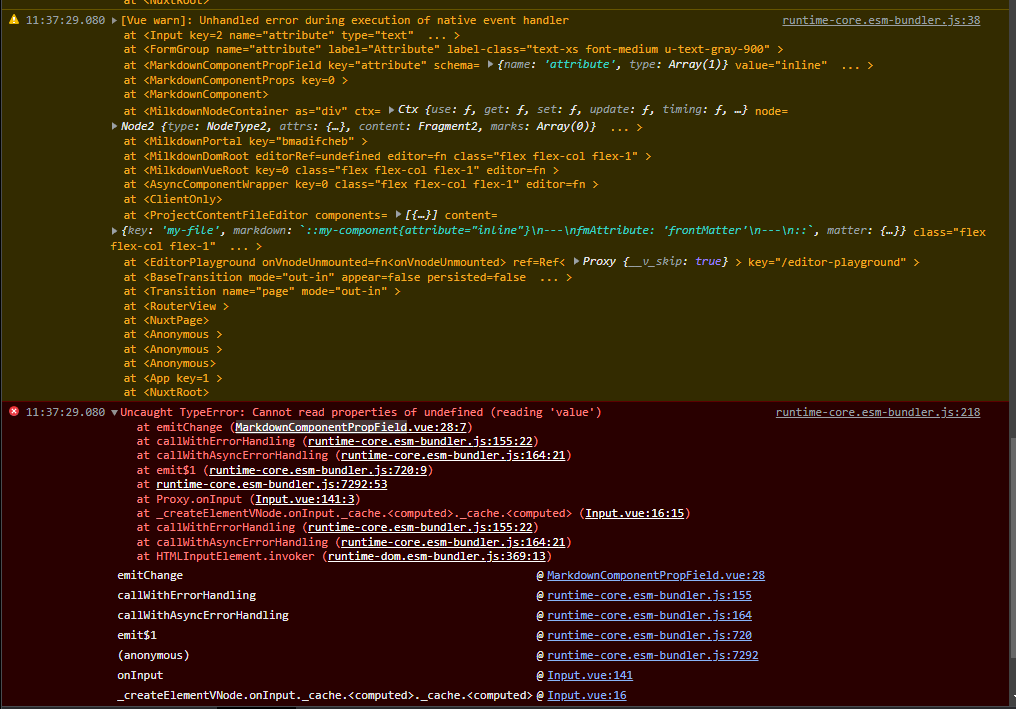\n",[2076],{"name":1996,"color":2077},"ff281a",507,"[Milkdown] Fix component props update","2022-05-16T15:51:55Z","https://github.com/nuxt/nuxt.com/issues/507",0.72248065,{"description":2084,"labels":2085,"number":2078,"owner":1985,"repository":1999,"state":2035,"title":2087,"updated_at":2037,"url":2088,"score":2082},"```\r\n[Vue warn]: A plugin must either be a function or an object with an \"install\" function.\r\n[Vue warn]: Failed to resolve component: RouterLink\r\nIf this is a native custom element, make sure to exclude it from component resolution via compilerOptions.isCustomElement.\r\n at \u003CNuxtLink to=\"/supplier-information\" >\r\n```\r\n\r\nRead docs here: https://test-utils.vuejs.org/guide/advanced/vue-router.html#using-a-real-router-with-composition-api cannot fix this.\r\n\r\n ```\r\nimport { mount } from '@vue/test-utils'\r\nimport {describe, it, expect, beforeEach} from 'vitest'\r\nimport Component from './app.vue'\r\nimport {Router} from 'vue-router'\r\n\r\nlet router:Router;\r\n\r\nbeforeEach(async () => {\r\n router = useRouter()\r\n\r\n router.push('/')\r\n await router.isReady()\r\n});\r\n\r\ndescribe(\"footer component\", async () => {\r\n const page = mount(Component, {\r\n props: {},\r\n global: {\r\n plugins: [router]\r\n }\r\n })\r\n it(\"should xxx\", async () => {})\r\n})\r\n```\r\n",[2086],{"name":2032,"color":2033},"Failed to resolve component: RouterLink","https://github.com/nuxt/test-utils/issues/507",["Reactive",2090],{},["Set"],["ShallowReactive",2093],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"w2RSiyHQhPqKdjM-ME7fHobySTMT1Jxd6YHBaAZoJEo":-1},"/nuxt/nuxt.com/555"]