//Sets data to store in useAsyncData\r\n {{ store.data }} //Initial value instead of data there\r\n \u003Cmy-component-that-rely-on-store/> //Reactivity is lost for this guy\r\n \u003C/div>\r\n\u003C/template>\r\n```\r\n\r\nThe reason behind this as I understand looks like this:\r\n\r\n```vue\r\n\u003Ctemplate>\r\n \u003Cdiv>\r\n \u003Cslot/> //Inits useAsyncData, leaves to separate flow after first await\r\n {{ store.data }} //Would be valid, if would not initiated on async\r\n \u003Cmy-component-that-rely-on-store/> //useAsyncData has been called, but not completed\r\n \u003C/div>\r\n\u003C/template>\r\n```\r\n\r\n## Solution\r\n\r\n### New (probably SSR-only) hook\r\n\r\nInproduce new hook, that will fire after all asyncDatas will be completed and most of components rendered\r\n\r\n### New component\r\n\r\nThe name could be like `\u003Cnuxt-server-rendered>`, `\u003Cnuxt-server-init>`, e.t.c.\r\n\r\nComponent will rely on this new hook and could use `onServerPrefetch` under-the-hood. Then it could be used like this:\r\n```vue\r\n\u003Ctemplate>\r\n \u003Cdiv>\r\n \u003Cslot/> //Inits useAsyncData, leaves to separate flow after first await\r\n \u003Cnuxt-server-rendered>\r\n {{ store.data }} //Valid data\r\n \u003Cmy-component-that-rely-on-store/> //Valid data\r\n \u003C/nuxt-async-resolve>\r\n \u003C/div>\r\n\u003C/template>\r\n```\r\n\r\nIt will also resolve reactivity problems with parent components, and not only async problems, the problem that was described in issues/discussion above.\r\n\r\n## Pure implementation\r\n\r\nI've tried to implement this by myself using this:\r\n\r\n```vue\r\n\u003Ctemplate>\r\n \u003Cslot/>\r\n\u003C/template>\r\n\r\n\u003Cscript lang=\"ts\" setup>\r\nonServerPrefetch(async () => {\r\n await Promise.allSettled(Object.values(toValue(app._asyncDataPromises)));\r\n});\r\n\u003C/script>\r\n```\r\n\r\nThis solution is not good actually, but it goes as workaround with limitations:\r\n- I have no guarantee that _asyncDataPromises are complete on this stage\r\n- I can only use this component after page's \u003Cslot>, therefore I currently can't use it with components above page, e.g. header\r\n- It does not rely on render or anything like that (if that's even possible at all in current SSR implementation)\r\n\r\nI could try to implement this myself, if this looks valid, but I'm not sure I can do that with my limited knowlenge of Nuxt's internals. \n\n### Additional information\n\n- [X] Would you be willing to help implement this feature?\n- [ ] Could this feature be implemented as a module?\n\n### Final checks\n\n- [X] Read the [contribution guide](https://nuxt.com/docs/community/contribution).\n- [X] Check existing [discussions](https://github.com/nuxt/nuxt/discussions) and [issues](https://github.com/nuxt/nuxt/issues).",[1984],{"name":1985,"color":1986},"pending triage","E99695",25316,"nuxt","open","Allow to await for useAsyncData in template","2024-06-30T11:06:12Z","https://github.com/nuxt/nuxt/issues/25316",0.65239364,{"description":1995,"labels":1996,"number":2001,"owner":1988,"repository":1988,"state":1989,"title":2002,"updated_at":2003,"url":2004,"score":2005},"### Environment\n\nNuxt project info: 1:24:04 AM\r\n\r\n------------------------------\r\n- Operating System: Windows_NT\r\n- Node Version: v20.11.0\r\n- Nuxt Version: 3.11.2\r\n- CLI Version: 3.11.1\r\n- Nitro Version: 2.9.6\r\n- Package Manager: yarn@1.22.21\r\n- Builder: -\r\n- User Config: devtools, runtimeConfig, routeRules, storyblok, modules, vue, vite, components, app, css, postcss\r\n- Runtime Modules: @pinia/nuxt@0.5.1, @storyblok/nuxt@6.0.10\r\n- Build Modules: -\r\n------------------------------\n\n### Reproduction\n\nAs far as i can tell this is pretty obvious and it seems to be happening in most nuxt3 projects.\n\n### Describe the bug\n\nIf `useLazyAsyncData` is used together with an `await` (to be able to show for example ghosts before the data has finished fetching) and together with a `getCachedData` function, the lazy async data is no longer returning pending and is instead waiting until the data is available (similar to how just a normal `useAsyncData` works).\n\n### Additional context\n\nWe found this issue while trying to use `getCachedData` to improve loading times since our app keeps fetching the same data on navigation.\n\n### Logs\n\n_No response_",[1997,1998],{"name":1985,"color":1986},{"name":1999,"color":2000},"needs reproduction","FBCA04",27351,"Using `getCachedData` together with `await useLazyAsyncData` causing it to not be pending anymore","2025-03-17T15:01:13Z","https://github.com/nuxt/nuxt/issues/27351",0.6637444,{"description":2007,"labels":2008,"number":2013,"owner":1988,"repository":1988,"state":2014,"title":2015,"updated_at":2016,"url":2017,"score":2018},"### Describe the feature\r\n\r\nIt would be nice to make refreshNuxtData util re-execute asyncData define using options api\r\n```vue\r\n\u003Cscript>\r\nexport default defineNuxtComponent({\r\n asyncData() {\r\n await exampleApiCall()\r\n return {}\r\n }\r\n})\r\n\u003C/script>\r\n```\r\n\r\nNuxt 2 had this behaviour with `this.$nuxt.refresh()` which re-executes fetch and asyncData functions\r\n\r\n### Additional information\r\n\r\n- [ ] Would you be willing to help implement this feature?\r\n- [ ] Could this feature be implemented as a module?\r\n\r\n### Final checks\r\n\r\n- [X] Read the [contribution guide](https://nuxt.com/docs/community/contribution).\r\n- [X] Check existing [discussions](https://github.com/nuxt/nuxt/discussions) and [issues](https://github.com/nuxt/nuxt/issues).",[2009,2012],{"name":2010,"color":2011},"3.x","29bc7f",{"name":1985,"color":1986},20722,"closed","refreshNuxtData should reload options API's asyncData ","2024-10-10T14:45:33Z","https://github.com/nuxt/nuxt/issues/20722",0.6384845,{"description":2020,"labels":2021,"number":2024,"owner":1988,"repository":1988,"state":2014,"title":2025,"updated_at":2026,"url":2027,"score":2028},"### Describe the feature\r\n\r\nI thought this was already the case in useAsyncData but after some issues in my project and finding this issue (https://github.com/nuxt/nuxt/issues/22622) it is clear this is not the case yet.\r\n\r\nIt would be great if we would have an option to integrate caching using useNuxtData in the useAsyncData itself. \r\nI have been able to implement this myself with a workaround like:\r\n\r\n``\r\nuseAsyncData(`page-${id}`, async () => {\r\n const { data: cachedPage } = useNuxtData(`page-${id}`);\r\n return (\r\n cachedPage.value || (await getPage(id))\r\n );\r\n})\r\n``\r\n\r\nWhich works great, it's just a bit less clean.\r\nThe only issue is that if you would want to use the default refresh function provided by the useAsyncData composable this would not really refresh but just use the cache.\r\n\r\n### Additional information\r\n\r\n- [ ] Would you be willing to help implement this feature?\r\n- [ ] Could this feature be implemented as a module?\r\n\r\n### Final checks\r\n\r\n- [X] Read the [contribution guide](https://nuxt.com/docs/community/contribution).\r\n- [X] Check existing [discussions](https://github.com/nuxt/nuxt/discussions) and [issues](https://github.com/nuxt/nuxt/issues).",[2022,2023],{"name":2010,"color":2011},{"name":1985,"color":1986},23540,"NuxtData caching built into useAsyncData","2023-10-27T13:01:36Z","https://github.com/nuxt/nuxt/issues/23540",0.64277023,{"description":2030,"labels":2031,"number":2039,"owner":1988,"repository":1988,"state":2014,"title":2040,"updated_at":2041,"url":2042,"score":2043},"### Environment\r\n\r\n------------------------------\r\n- Operating System: Linux\r\n- Node Version: v18.16.1\r\n- Nuxt Version: 3.6.1\r\n- Nitro Version: 2.5.2\r\n- Package Manager: yarn@1.22.19\r\n- Builder: vite\r\n- User Config: runtimeConfig, alias, css, components, vite, dir, plugins, modules, pwa\r\n- Runtime Modules: nuxt-jsonld@2.0.8, @vite-pwa/nuxt@0.1.0, @pinia/nuxt@0.4.11, @nuxtjs/eslint-module@4.1.0\r\n- Build Modules: -\r\n------------------------------\r\n\r\n### Reproduction\r\n\r\nhttps://stackblitz.com/edit/github-tex5o8?file=pages%2Findex.vue\r\n\r\n### Describe the bug\r\n\r\nI've checked docs and as I see `useAsyncData` should cache data for response with same key.\r\n\r\nSo for example:\r\n```\r\nconst KEY = 'test';\r\nconst { data: data } = await useAsyncData(KEY, async () => {\r\n console.log('test');\r\n return {};\r\n});\r\nconst { data: data2 } = await useAsyncData(KEY, async () => {\r\n console.log('test');\r\n return {};\r\n});\r\nconst { data: data3 } = await useAsyncData(KEY, async () => {\r\n console.log('test');\r\n return {};\r\n});\r\n```\r\n\r\nThis should show only once `'test'` in console, but it shows it three times.\r\n\r\nIn real world app I need to fetch data in component A and then use same data in page B.\r\nRight now the only option that I see is to make two api calls.\r\n\r\nIn nuxt 2, I've used `fetch` component option method to make call in component A and I didn't even need to make call in page B, because I've already had data in vuex (from component A). How to handle that (without duplicated requests) if `useAsyncData` doesn't provide cache?\r\n\r\n### Additional context\r\n\r\n_No response_\r\n\r\n### Logs\r\n\r\n_No response_",[2032,2035,2038],{"name":2033,"color":2034},"documentation","5319e7",{"name":2036,"color":2037},"good first issue","fbca04",{"name":2010,"color":2011},22622,"Clarify that `useAsyncData` results are not cached","2023-12-14T11:09:24Z","https://github.com/nuxt/nuxt/issues/22622",0.64567554,{"labels":2045,"number":2053,"owner":1988,"repository":1988,"state":2014,"title":2054,"updated_at":2055,"url":2056,"score":2057},[2046,2049,2050],{"name":2047,"color":2048},"discussion","538de2",{"name":2010,"color":2011},{"name":2051,"color":2052},"app","17512D",11742,"Improve `asyncData` API","2023-01-19T15:42:33Z","https://github.com/nuxt/nuxt/issues/11742",0.6488878,{"description":2059,"labels":2060,"number":2063,"owner":1988,"repository":1988,"state":2014,"title":2064,"updated_at":2065,"url":2066,"score":2067},"### Environment\n\n- Operating System: Windows_NT\r\n- Node Version: v21.1.0\r\n- Nuxt Version: 3.10.2\r\n- CLI Version: 3.10.1\r\n- Nitro Version: 2.8.1\r\n- Package Manager: npm@10.2.5\r\n- Builder: -\r\n- User Config: devtools\r\n- Runtime Modules: -\r\n- Build Modules: -\n\n### Reproduction\n\nhttps://stackblitz.com/edit/github-yjwcwx\n\n### Describe the bug\n\nWhen\r\n`useAsyncData` `key` is set to `defer`\r\n\r\nHaving the request on `App.vue` and the children causes it to make the request twice. I would expect this to be make the request once when the page is reloaded. I guess currently It doesn't dedupe the request correctly when it's on different levels even though the key is the same. Also the `refreshNuxtData` runs once as it should. But this behavior is different than what happens when you refresh the page.\r\n\r\nWhen\r\n`useAsyncData` `key` is set to `cancel`\r\n\r\nThis is the worst case scenario. Makes request for every `useAsyncData`. The same goes for `refreshNuxtData`. No dedupe at all.\r\n\r\n\r\n\r\n\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2061,2062],{"name":2010,"color":2011},{"name":1985,"color":1986},25853,"UseFetch / UseAsyncData Dedupe not Working","2024-06-20T11:09:06Z","https://github.com/nuxt/nuxt/issues/25853",0.6498105,{"description":2069,"labels":2070,"number":2073,"owner":1988,"repository":1988,"state":2014,"title":2074,"updated_at":2075,"url":2076,"score":2077},"### Describe the feature\r\n\r\nCommon use case: performing some asynchronous actions, but not when the component is loaded, but in response to user actions. It would be very useful to have a utility that offers the same api as `useAsyncData`, but writes the callback function not when the component is mounted, but only when it is explicitly called\r\n\r\n```vue\r\n\u003Cscript setup>\r\nconst { error, pending, execute, data } = useAsyncAction(\r\n // The first argument is nuxt context to be consistent with useAsyncData. \r\n // All next arguments passed from `execute` call\r\n (ctx, event) => $fetch('api/save-data', new FormData(event.target)) \r\n) \r\n\u003C/script>\r\n\r\n\u003Ctemplate>\r\n\u003Cform @submit.prevent=\"execute\">\r\n \u003Cdiv v-if=\"error\">Failed to save data to server {{error}}\u003C/div>\r\n \u003Cdiv v-else-if=\"pending\">Saving ...\u003C/div>\r\n \u003Cdiv v-else-if=\"data\">Succesfully saved\u003C/div>\r\n\u003C/form>\r\n\u003C/template>\r\n```\r\n\r\n### Additional information\r\n\r\n- [ ] Would you be willing to help implement this feature?\r\n- [ ] Could this feature be implemented as a module?\r\n\r\n### Final checks\r\n\r\n- [X] Read the [contribution guide](https://v3.nuxtjs.org/community/contribution).\r\n- [X] Check existing [discussions](https://github.com/nuxt/framework/discussions) and [issues](https://github.com/nuxt/framework/issues).",[2071,2072],{"name":2010,"color":2011},{"name":1985,"color":1986},15673,"Provide `useAsyncAction` helper function","2023-03-28T14:09:45Z","https://github.com/nuxt/nuxt/issues/15673",0.6511244,{"description":2079,"labels":2080,"number":2083,"owner":1988,"repository":1988,"state":2014,"title":2084,"updated_at":2085,"url":2086,"score":2087},"### Describe the feature\r\n\r\nThe cache for the data fetching mechanism is already accessible via `useNuxtApp().payload.data`, I'm suggesting exposing it with a separate composable (`useNuxtData` or `getNuxtData`). it can introduce some interesting data-fetching patterns such as optimistic updates.\r\n\r\n### Examples\r\n- Showing stale data instead of a loading spinner (reading from cache): \r\n\r\n```ts\r\ninterface Post {\r\n userId: number;\r\n title: string;\r\n body: string;\r\n id: number\r\n}\r\n\r\nconst id = useRoute().params.id\r\n\r\nconst { data } = useLazyFetch(`/api/post/${id}`, {\r\n key: `post-${id}`,\r\n server: false,\r\n default: () => {\r\n return (useNuxtData().posts as Post[]).find(d => d.id === +id)\r\n }\r\n})\r\n```\r\n\r\n- Update the UI while the query is being invalidated in the background (writing to cache): \r\n\r\n```ts\r\n// in List.vue\r\nconst { data } = useLazyFetch('/api/notes', {\r\n key: 'notes'\r\n})\r\n\r\n// In Form.vue\r\nconst text = ref('')\r\n\r\nconst add = () => {\r\n (useNuxtData().notes as string[]).push(text.value)\r\n // ... Some mutation\r\n refreshNuxtData('notes')\r\n}\r\n```\r\n\r\n### Additional information\r\n\r\n- [X] Would you be willing to help implement this feature?\r\n- [X] Could this feature be implemented as a module?\r\n\r\n### Final checks\r\n\r\n- [X] Read the [contribution guide](https://v3.nuxtjs.org/community/contribution).\r\n- [X] Check existing [discussions](https://github.com/nuxt/framework/discussions) and [issues](https://github.com/nuxt/framework/issues).",[2081,2082],{"name":2010,"color":2011},{"name":1985,"color":1986},15582,"Accessing async data cache object with a new composable","2023-01-19T17:56:33Z","https://github.com/nuxt/nuxt/issues/15582",0.653791,{"description":2089,"labels":2090,"number":2093,"owner":1988,"repository":1988,"state":2014,"title":2094,"updated_at":2095,"url":2096,"score":2097},"### Environment\n\nrc-13\r\nnode-18\n\n### Reproduction\n\n//composable\r\n```javascript\r\nconst myfetch = async (url,options,emits) => {\r\n const { data, pending, error, refresh } = await useFetch(url, {\r\n \r\n ...options,\r\n baseURL: useRuntimeConfig().API_URL, \r\n initialCache: false,\r\n headers: new Headers({ 'authorization': (process.client)?localStorage.getItem(\"token\"):''}),\r\n onResponse ({ response }) {\r\n return response._data\r\n },\r\n onResponseError ({ response }) {\r\n return response._data\r\n }\r\n })\r\n const resData = data.value\r\n \r\n return resData\r\n }\r\n```\r\n//component 1\r\n\r\n```javascript\r\n\u003Cscript setup>\r\ngetAllItems();\r\nasync function getAllItems() {\r\n let response = await myfetch('/article/artPosts?' +\r\n 'page=' + page.value + '&' +\r\n 'artPostGroup=' + artPostGroup.value + '&' +\r\n 'limit=' + limit.value, {\r\n method: 'GET'\r\n });\r\n if (response.data)\r\n list.value = response.data.docs;\r\n pages.value = response.data.pages;\r\n}\r\n\u003C/script>\r\n```\r\n\r\n//component 2\r\n\r\n```javascript\r\n\u003Cscript setup>\r\ngetAllItems();\r\nasync function getAllItems() {\r\n let response = await myfetch('/store/storeCourses?' +\r\n 'page=' + page.value + '&' +\r\n 'storeCourseGroup=' + storeCourseGroup.value + '&' +\r\n 'limit=' + limit.value, {\r\n method: 'GET'\r\n });\r\n if (response.data)\r\n list.value = response.data.docs;\r\n pages.value = response.data.pages;\r\n}\r\n\u003C/script>\r\n```\r\n//home.vue\r\n```javascript\r\n\u003Cdiv>\r\n\u003Ccomponent1>\u003C/component1>\r\n\u003Ccomponent2>\u003C/component2>\r\n\u003C/div>\r\n```\n\n### Describe the bug\n\nthe bug is here...\r\nin the page load:\r\nresponse of component1 is the same as response of component2...\r\n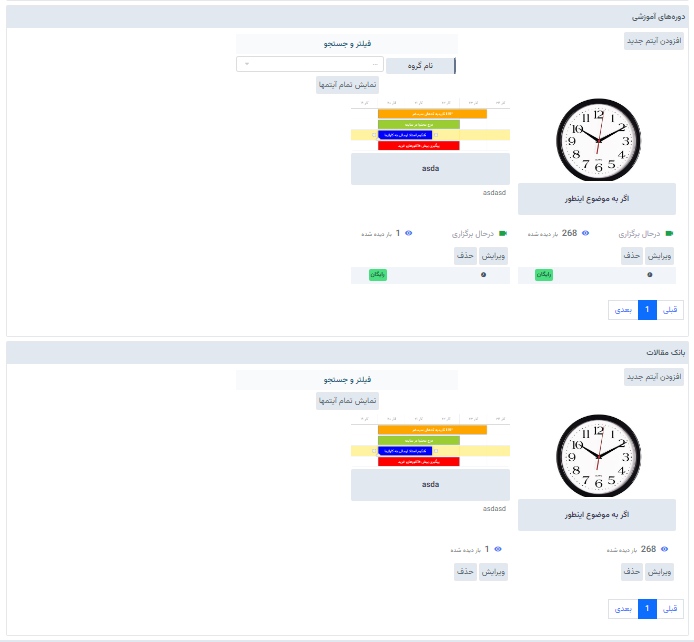\r\n\r\nwhen i use $fetch instead of useFetch, it works good but it makes another problem for me...\r\n\r\non refreshing page,it send a request twice to server\r\n\n\n### Additional context\n\nhow can i write function in composables?\n\n### Logs\n\n_No response_",[2091,2092],{"name":2010,"color":2011},{"name":1985,"color":1986},15451,"UseFetch() calling multiple request, only the last data is fetched and $fetch send requests twice","2023-01-19T17:50:37Z","https://github.com/nuxt/nuxt/issues/15451",0.6545399,["Reactive",2099],{},["Set"],["ShallowReactive",2102],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"o4Ox-E6gu8SO1EALjbFuIPDnZobzk2VnFl-pxbo5q7c":-1},"/nuxt/nuxt/29196"]