\r\n\u003C/template>\r\n```\r\n\r\nI want to have the post components within that page NOT async BUT still dynamic (so I can loop over the array) I want each instance of AbstractPage to be generated as one .js file.\r\nAbstractPage.vue:\r\n```\r\n\u003Ctemplate>\r\n \u003Cdiv v-for=\"postRoute of posts[page]\">\r\n \u003Ccomponent :is=\"postComponents.find(comp => comp.route === postRoute)\"/>\r\n \u003C/div>\r\n\u003C/template>\r\n\r\nfor (const postRoute of posts[this.page]) {\r\n const filename = routeToComponentFilename(postRoute)\r\n let esComponent = await import(`../PromoCards/${filename}`)\r\n const component = {\r\n route: postRoute,\r\n component: esComponent.default\r\n }\r\n this.postComponents.push(component)\r\n}\r\n```\r\n\r\nMy end goal for network requests (simplified) is: \r\nGET index.html\r\nGET app.js\r\nGET page0.js \u003C-- precompiles the dynamic NON async post components\r\n\r\nhere is my repo for more context: https://github.com/Coletrane/mountain-bike-virginia/tree/posts-json-authors-json\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c2681\">#c2681\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2961],{"name":2950,"color":2951},3097,"How to use dynamic NON-async components for nuxt generate","2023-01-18T16:09:58Z","https://github.com/nuxt/nuxt/issues/3097",0.65205544,{"description":2968,"labels":2969,"number":2971,"owner":2910,"repository":2910,"state":2953,"title":2972,"updated_at":2973,"url":2974,"score":2975},"Hello. I am trying to use manually created pages/routes along with dynamically generated, but seems like it is not working.\r\n\r\nIs there any option to use Nuxt like this:\r\n\r\n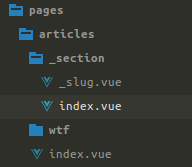\r\n\r\n`wtf` route doesn't work on `articles/wtf/` endpoint. Only the routes that comes from API in `_section` is working.\r\n\r\nSince I can't find proper way to insert components in dynamic routes (that fetch data and then `v-html` it from API), I want to have an opportunity sometimes create manually rich pages, where I can use components etc, and not being dependent on `v-for` and `v-html` schemes.\r\n\r\nHow to achieve that?\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1526\">#c1526\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2970],{"name":2950,"color":2951},1703,"Dynamic routes along with manually created?","2023-01-18T15:54:38Z","https://github.com/nuxt/nuxt/issues/1703",0.6612167,{"description":2977,"labels":2978,"number":2984,"owner":2910,"repository":2910,"state":2953,"title":2985,"updated_at":2986,"url":2987,"score":2988},"### What problem does this feature solve?\r\n\r\nI'm refactoring a system with nuxtjs and that system can be used by users to create your own \"sites\". So each site can be accessed with a configured path, for example mydomain.com/client1, mydomain.com/client2-my-site and mydomain.com/other-user-url (the pages inside each site are fixed, like mydomain.com/client1/about-us, mydomain.com/client1/our-work etc). \r\n\r\nI was able to work with custom routes (extendRoute), like this:\r\n\r\n```\r\nextendRoutes (routes, resolve) {\r\n routes.splice(0, routes.length); // reset nuxt automatic routes config\r\n routes.push({\r\n name: 'about-us',\r\n path: '/:myclient?/about-us',\r\n component: 'pages/about-us.vue',\r\n });\r\n}\r\n```\r\n\r\nBut that way I need to set a base path variable on store (on nuxtServerInit) and use it on all links because sites can also be accessed from external domain (premium feature), like myclient-owndomain.com/about-us. So this base path variable can be \"/\" (accessing from external domain) or \"/:myclient\" (accessing from internal domain) and must be used to render links, like:\r\n\r\n```\r\n\u003Cnuxt-link :to=\"$store.routerBasePath+'/about-us'\">About us\u003C/nuxt-link>\r\n```\r\n\r\nSo I think a better way to solving this is setting the router.base config dynamically (with a function), instead a fixed string.\r\n\r\nOr is there another way to do that on nuxtjs?\r\n\r\nThanks!\r\n\r\n### What does the proposed changes look like?\r\n\r\nThis feature will make router.base config more flexible (an alternative when necessary), using a syntax like that:\r\n\r\n```\r\nrouter: {\r\n base: function(context) {return context.params.myclient}\r\n}\r\n```\r\n\r\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\r\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This feature request is available on \u003Ca href=\"https://cmty.app/nuxt\">Nuxt\u003C/a> community (\u003Ca href=\"https://cmty.app/nuxt/nuxt.js/issues/c9299\">#c9299\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2979,2980,2983],{"name":2920,"color":2921},{"name":2981,"color":2982},"available soon","6de8b0",{"name":2950,"color":2951},5852,"Dynamic router base","2023-01-22T15:50:56Z","https://github.com/nuxt/nuxt/issues/5852",0.66160995,{"description":2990,"labels":2991,"number":2992,"owner":2910,"repository":2910,"state":2953,"title":2993,"updated_at":2994,"url":2995,"score":2996},"### Discussed in https://github.com/nuxt/nuxt/discussions/22444\r\n\r\n\u003Cdiv type='discussions-op-text'>\r\n\r\n\u003Csup>Originally posted by **sync42johnny** August 2, 2023\u003C/sup>\r\nI'm currently exploring dynamic routing in Nuxt 3 and I came across the documentation that explains how to define dynamic routes in the pages directory. According to the documentation here: https://nuxt.com/docs/guide/directory-structure/pages#dynamic-routes, dynamic routes can be easily defined in the pages directory using the file and directory names. For example:\r\n```\r\n-| pages/\r\n---| index.vue\r\n---| users-[group]/\r\n-----| [id].vue\r\n```\r\nI'm particularly intrigued by the users-[group]/ part of the structure, which seems to suggest that dynamic parameters can be used in parent directory names as well. This could greatly simplify the routing logic for some of my use cases.\r\n\r\nMy question is this: Does Nuxt 3 support the same functionality for server routes? Can I dynamically generate server routes based on parameters like in the users-[group]/ example?\r\n\r\nIf so, could you kindly guide me on how to implement this? If not, are there plans to include this feature in future releases, or could you recommend a workaround?\r\n\r\nYour insights would be greatly appreciated!\r\n\r\nThank you.😀\u003C/div>",[],23704,"🔥Dynamic Routes in Server Routes for Nuxt 3🔥","2023-10-16T20:09:34Z","https://github.com/nuxt/nuxt/issues/23704",0.6679103,{"labels":2998,"number":3001,"owner":2910,"repository":2910,"state":2953,"title":3002,"updated_at":3003,"url":3004,"score":3005},[2999,3000],{"name":2920,"color":2921},{"name":2950,"color":2951},7186,"Support of dynamic routes on client","2023-01-22T15:52:36Z","https://github.com/nuxt/nuxt/issues/7186",0.6700441,{"description":3007,"labels":3008,"number":3013,"owner":2910,"repository":2910,"state":2953,"title":3014,"updated_at":3015,"url":3016,"score":3017},"The problem with adding routes dynamically \r\nIn the production version, I plan to use an ajax request that gives the \"page type\" in the plugin and add the appropriate routes. For the demonstration, I created the minimum set of code required to run and test the dynamic addition of routes, please see \r\n\r\nMy nuxt.config.js\r\n```\r\nmodule.exports = {\r\n ssr: true,\r\n mode: 'universal',\r\n srcDir: __dirname,\r\n loading: { color: '#007bff' },\r\n plugins: [\r\n {src: '~/plugins/route'}\r\n ],\r\n modules: [\r\n \"@nuxtjs/axios\",\r\n [\r\n 'nuxt-i18n', {\r\n parsePages: false,\r\n pages: {\r\n contacts: {\r\n en: '/kont_test2',\r\n uk: '/kont_test3',\r\n ru: '/kont_test4',\r\n }},\r\n\r\n strategy:'prefix_except_default',\r\n detectBrowserLanguage: {\r\n useCookie: true,\r\n cookieKey: 'i18n_redirected',\r\n alwaysRedirect: true,\r\n fallbackLocale: 'en'\r\n },\r\n vueI18nLoader: true,\r\n locales: [\r\n {\r\n name: 'English',\r\n code: 'en',\r\n iso: 'en-US',\r\n file: 'en.js'\r\n },\r\n {\r\n name: 'Русский',\r\n code: 'ru',\r\n iso: 'ru-RU',\r\n file: 'ru.js'\r\n },\r\n {\r\n name: 'Українська',\r\n code: 'uk',\r\n iso: 'uk-UA',\r\n file: 'uk.js'\r\n },\r\n ],\r\n\r\n lazy: true,\r\n loadLanguagesAsync: true,\r\n silentTranslationWarn:true,\r\n langDir: 'locales/',\r\n defaultLocale: 'ru',\r\n\r\n\r\n },\r\n ],],\r\n hooks: {\r\n generate: {\r\n done (generator) {\r\n // Copy dist files to public/_nuxt\r\n if (generator.nuxt.options.dev === false && generator.nuxt.options.mode === 'spa') {\r\n const publicDir = join(generator.nuxt.options.rootDir, 'public', '_nuxt')\r\n removeSync(publicDir)\r\n copySync(join(generator.nuxt.options.generate.dir, '_nuxt'), publicDir)\r\n copySync(join(generator.nuxt.options.generate.dir, '200.html'), join(publicDir, 'index.html'))\r\n removeSync(generator.nuxt.options.generate.dir)\r\n }\r\n },\r\n\r\n }\r\n },\r\n build: {\r\n extend (config, { isDev, isClient }) {\r\n config.node = {\r\n fs: \"empty\"\r\n }\r\n }\r\n }\r\n\r\n}\r\n\r\n```\r\n\r\nIn the config I add plugin \"route\", plugins/route.js source code\r\n```\r\nfunction interopDefault(promise) {\r\n return promise.then(m => m.default || m);\r\n}\r\n\r\n\r\nexport default ({app, router, routeres}) => {\r\n\r\n //axios.defaults.baseURL = process.env.apiUrl;\r\n // console.log(app.router);\r\n //afterEach\r\n\r\n app.router.beforeEach((to, from, next) => {\r\n\r\n const matched = app.router.getMatchedComponents(to);\r\n console.log(\"matched: \" + matched.length);\r\n console.log(\"to.path: \" + to.path);\r\n\r\n\r\n let dynamicView = () => interopDefault(import('~pages/post.vue'));\r\n \r\n if (!matched.length) {\r\n console.log('not-exist');\r\n\r\n app.router.addRoutes([\r\n {\r\n path: to.path,\r\n component: dynamicView, \r\n }], { override: true });\r\n\r\n\r\n next(to.path);\r\n\r\n \r\n } else {\r\n console.log('route exist');\r\n next() \r\n }\r\n \r\n })\r\n}\r\n```\r\n\r\npages/post.vue\r\n```\r\n\u003Ctemplate>\r\n \u003Cdiv class = \"test\">test post page for this path\u003C/div>\r\n\u003C/template>\r\n\r\n\u003Cscript>\r\n export default {\r\n \r\n }\r\n\u003C/script>\r\n```\r\n\r\n\r\nСode is quite simplified, when checking if (! Matched.length) we add a simple march page \"pages / post.vue\" and should display the simple text \"test post page for this path\"\r\nFor example, by contacting any url that does not exist, the route should be added.\r\nexample.com/other_url\r\nFrom time to time I see errors:\r\nCannot read property 'scrollToTop' of undefined (for now)\r\nCannot read property 'layout' of undefined error (periodically)\r\n404 page not found (periodically)\r\n\r\n\r\nLink to \"codesandbox\"\r\nhttps://codesandbox.io/s/nuxt-nuxt-i18n-issue-1044-forked-t33du\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n",[3009,3012],{"name":3010,"color":3011},"pending triage","E99695",{"name":2950,"color":2951},8696,"Nuxt dynamic routes, addRoutes ssr, 404 or Cannot read property 'layout' of undefined error or can't access property \"scrollToTop\", Page.options is undefined","2023-01-22T15:38:40Z","https://github.com/nuxt/nuxt/issues/8696",0.67082417,["Reactive",3019],{},["Set"],["ShallowReactive",3022],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fYhcm7yIpqnVnp9ufDV1HWgnKFj0QwCiVfofV47DrVL8":-1},"/nuxt/nuxt/6838"]