\r\n\u003C/template>\r\n\r\n\u003Cscript setup>\r\nconst route = useRoute();\r\nconst uid = ref(route.params.uid[0] || \"home\");\r\n// Create the prismic endpoint.\r\nconst prism = usePrismic({ type: \"page\", uid: uid.value });\r\nconst { data: page } = await useFetch(prism.endpoint, {\r\n key: uid.value,\r\n params: prism.params,\r\n transform: (data) => {\r\n let result = data.results[0].data;\r\n // Remove unwanted special characters from the Prismic body.\r\n result = JSON.stringify(result);\r\n result = result.replace(/[\\u2028]/g, \"\");\r\n result = JSON.parse(result);\r\n return result;\r\n },\r\n});\r\n\r\n// Prepare the async loading of the slices.\r\nconst sliceList = {\r\n navigation: defineAsyncComponent(() => import(\"~/components/Navigation.vue\")),\r\n landing_hero: defineAsyncComponent(() => import(\"~/components/LandingHero.vue\")),\r\n heading: defineAsyncComponent(() => import(\"~/components/Heading.vue\")),\r\n footer: defineAsyncComponent(() => import(\"~/components/Footer.vue\")),\r\n};\r\n\u003C/script>\r\n```\r\n\r\nDuring SSG, all the content coming from Prismic is properly rendered as HTML, which is awesome.\r\n\r\n#### The issue \r\n\r\nHowever, after building and uploading the page to production, a \"flicker\" appears when visiting the page.\r\nWhen I say flicker, what I mean is: the page is visible, the page disappears, the page re-appears. \r\n\r\nThis is how it looks like on mobile:\r\n\r\n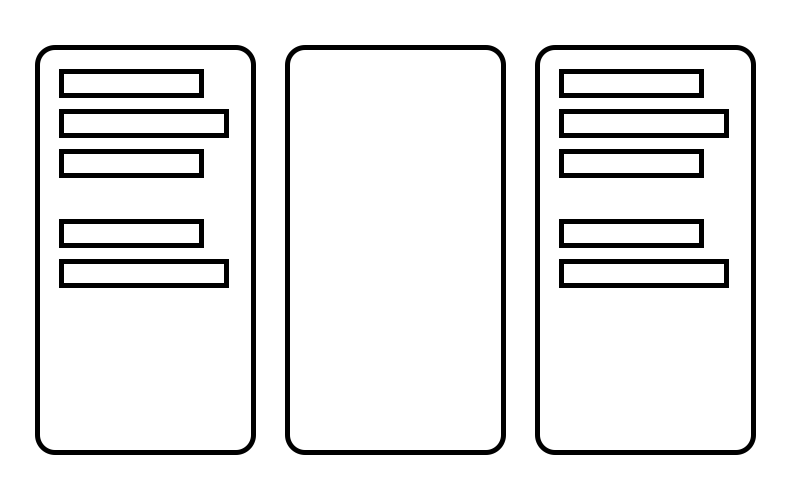\r\n\r\nI don't encounter the issue when avoiding to dynamically load the components, like this:\r\n\r\n```vue\r\n\u003Ctemplate>\r\n \u003CFlex direction=\"col\">\r\n \u003Ctemplate\r\n v-for=\"(slice, index) in page.body\"\r\n :key=\"`${uid}-${slice.slice_type}-${index}`\"\r\n >\r\n \u003CNavigation v-if=\"slice.slice_type === 'navigation'\" :slice=\"slice\" />\r\n \u003CLandingHero v-if=\"slice.slice_type === 'landing-hero'\" :slice=\"slice\" />\r\n \u003CHeading v-if=\"slice.slice_type === 'heading'\" :slice=\"slice\" />\r\n \u003CFooter v-if=\"slice.slice_type === 'footer'\" :slice=\"slice\" />\r\n \u003C/template>\r\n \u003C/Flex>\r\n\u003C/template>\r\n\r\n\u003Cscript setup>\r\nconst route = useRoute();\r\nconst uid = ref(route.params.uid[0] || \"home\");\r\n// Create the prismic endpoint.\r\nconst prism = usePrismic({ type: \"page\", uid: uid.value });\r\nconst { data: page } = await useFetch(prism.endpoint, {\r\n key: uid.value,\r\n params: prism.params,\r\n transform: (data) => {\r\n let result = data.results[0].data;\r\n // Remove unwanted special characters from the Prismic body.\r\n result = JSON.stringify(result);\r\n result = result.replace(/[\\u2028]/g, \"\");\r\n result = JSON.parse(result);\r\n return result;\r\n },\r\n});\r\n\u003C/script>\r\n``` \r\n\r\nHowever, this is of course not maintainable when you have a longer list of components.\r\n\r\n\r\n### Additional context\r\n\r\nTime to share you additional information. Which is where I'm _really_ scratching my head.\r\n\r\n#### @nuxtjs/prismic\r\nI'm aware that what I'm doing is a custom made solution, since @nuxtjs/prismic has a v3 version. However, at the time I was using this, it was still unstable and now the [https://v3.prismic.nuxtjs.org/](https://v3.prismic.nuxtjs.org/) seems to be completely down.\r\n\r\n#### An impact of CloudFlare?\r\nOn production, there are 2 urls. For the sake of privacy, I'm gonna invent some domain names here that aren't related to this project.\r\n\r\nThe first is the official URL created by [CloudFlare pages](https://pages.cloudflare.com/) - which is how we deploy our website.\r\nThis could look like: `my-website-name.pages.dev`\r\nThe second url is added to our DNS. The path is: `nuxt.our-domain.com`, which is proxied to `my-website-name.pages.dev`\r\n\r\nOn the first URL, the flicker doesn't appear, but it does happen on the second url (`nuxt.our-domain.com`)\r\n\r\nThose who know about CloudFlare would probably ask: Did you [disable \"Rocket Loader\"](https://community.cloudflare.com/t/rocket-loader-causes-blink-flicker-on-page-load/27181)?\r\nThe flickering happens even with Rocket Loader disabled.\r\n\r\nDue to this, I'm not sure if it's a Nuxt 3 or a CloudFlare issue. So I do apologize if it ends up being the latter.\r\n\r\nRemember, I can resolve the issue with the second block in the above mentioned bug description, so maybe it's the way the components are loaded dynamically?\r\n\r\n#### Possibly a related issue\r\nThis may or may not be related to this open issue: [useHead causes flicker when hydrating SSR-rendered page](https://github.com/nuxt/nuxt.js/issues/14271). I still encounter the issue without using `useHead`, but since this user is also encountering flickering,\r\n\r\n### Logs\r\n\r\n_No response_",[2965,2968],{"name":2966,"color":2967},"3.x","29bc7f",{"name":2905,"color":2906},15019,"(SSG) Using dynamic components to load a page creates a page flicker","2023-01-19T17:43:50Z","https://github.com/nuxt/nuxt/issues/15019",0.75110984,{"labels":2975,"number":2982,"owner":2908,"repository":2908,"state":2921,"title":2983,"updated_at":2984,"url":2985,"score":2986},[2976,2978,2979],{"name":2918,"color":2977},"8DEF37",{"name":2966,"color":2967},{"name":2980,"color":2981},"upstream","E8A36D",13345,"non-`\u003Chead>` locations for scripts","2023-01-19T17:07:31Z","https://github.com/nuxt/nuxt/issues/13345",0.75644624,{"description":2988,"labels":2989,"number":2990,"owner":2908,"repository":2991,"state":2921,"title":2992,"updated_at":2993,"url":2994,"score":2995},"Does this module support variable fonts? It works with the google-fonts module, but I would be interested in switching to this module at some point if variable font support is added.",[],74,"fonts","Variable Fonts?","2024-04-01T15:00:49Z","https://github.com/nuxt/fonts/issues/74",0.7566303,{"description":2997,"labels":2998,"number":3000,"owner":2908,"repository":2920,"state":2921,"title":3001,"updated_at":3002,"url":3003,"score":3004},"> As of right now, the `ui` module does not support overriding the gray color",[2999],{"name":2918,"color":2919},486,"Dark mode with custom gray palette","2022-05-18T09:03:31Z","https://github.com/nuxt/nuxt.com/issues/486",0.7613023,["Reactive",3006],{},["Set"],["ShallowReactive",3009],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fHnRxLqCG9uEgCiWLEui-YAPiL47Fo8berM3xU799R7U":-1},"/nuxt/test-utils/497"]