\r\n\u003C/template>\r\n\u003Cscript setup lang=\"ts\">\u003C/script>\r\n```\r\n\r\nApp.vue\r\n```\r\n\u003Ctemplate>\r\n \u003Cdiv>Message: {{ getMessage(\"Hello World\") }}\u003C/div>\r\n\u003C/template>\r\n\u003Cscript setup lang=\"ts\">\r\nimport { getMessage } from \"@/service/message-service\";\r\n```\r\n\r\nApp.test.ts\r\n```\r\nimport { it, expect, vi } from \"vitest\";\r\nimport { mountSuspended } from \"@nuxt/test-utils/runtime\";\r\nimport App from \"../../layouts/default.vue\";\r\n\r\nvi.mock(\"@/service/message-service\", () => {\r\n return {\r\n getMessage: () => \"hello from mock!!\",\r\n };\r\n});\r\n\r\n\r\nit(\"nuxt unit testing\", async () => {\r\n const component = await mountSuspended(App, { route: \"/\" });\r\n expect(component.html()).toMatchInlineSnapshot(\r\n `\"\u003Cdiv>Message: hello Hello World\u003C/div>\"`\r\n );\r\n});\r\n\r\n```\r\n\r\nmessage-service.ts\r\n```\r\nexport function getMessage(message: string): string {\r\n console.log(\"FROM ACTUAL\");\r\n return `hell ${message}`;\r\n}\r\n```\r\n\n\n### Describe the bug\n\nI was expecting vitest vi.mock should be able to mock imports to provide custom/mock implementation, however it seems that vi.mock is not working in the context of nuxt test?\r\n\r\nIf so, how are we suppose to create unit tests and/or E2E using nuxt/test-utils package and be able to provide flexible mocking mechanism?\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2001,2002,2005],{"name":1985,"color":1986},{"name":2003,"color":2004},"vitest-environment","b60205",{"name":2006,"color":2007},"pending triage","5D08F5",935,"test-utils","How to mock custom imports while using mountSuspended to test","2024-09-09T02:17:10Z","https://github.com/nuxt/test-utils/issues/935",0.75852454,{"description":2015,"labels":2016,"number":2018,"owner":1991,"repository":2009,"state":1993,"title":2019,"updated_at":2020,"url":2021,"score":2022},"### Environment\r\n\r\n- Operating System: `Darwin`\r\n- Node Version: `v20.8.1`\r\n- Nuxt Version: `3.9.0`\r\n- CLI Version: `3.10.0`\r\n- Nitro Version: `2.8.1`\r\n- Package Manager: `yarn@1.22.19`\r\n- Builder: `-`\r\n- User Config: `devtools`, `modules`\r\n- Runtime Modules: `@nuxt/test-utils/module@3.9.0`\r\n- Build Modules: `-`\r\n\r\n\r\n### Reproduction\r\n\r\nhttps://stackblitz.com/edit/github-gee6qy?file=app.vue\r\n\r\n### Describe the bug\r\n\r\nOnce I expose a variable called `error` within the setup, the component fails to render. \r\nSince everything works within the dev server / build, I suspect this is something within `@nuxt/test-utils`\r\n\r\nAfter renaming the `error` variable to something else, the component can be mounted within the tests again.\r\n\r\n### Additional context\r\n\r\n_No response_\r\n\r\n### Logs\r\n\r\n```shell-script\r\nVitest caught 1 unhandled error during the test run.\r\nThis might cause false positive tests. Resolve unhandled errors to make sure your tests are not affected.\r\n\r\n⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯ Unhandled Rejection ⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯\r\nTypeError: 'set' on proxy: trap returned falsish for property 'error'\r\n ❯ clonedComponent.render node_modules/@nuxt/test-utils/dist/runtime-utils/index.mjs:128:44\r\n 126| renderContext[key] = passedProps[key];\r\n 127| }\r\n 128| return render.call(this, renderContext, ...args);\r\n | ^\r\n 129| } : void 0,\r\n 130| setup: setup ? (props2) => wrappedSetup(props2, setupContext) : void 0\r\n ❯ renderComponentRoot node_modules/@vue/runtime-core/dist/runtime-core.cjs.js:868:16\r\n ❯ ReactiveEffect.componentUpdateFn node_modules/@vue/runtime-core/dist/runtime-core.cjs.js:5922:46\r\n ❯ ReactiveEffect.run node_modules/@vue/reactivity/dist/reactivity.cjs.js:174:19\r\n ❯ instance.update node_modules/@vue/runtime-core/dist/runtime-core.cjs.js:6053:16\r\n ❯ setupRenderEffect node_modules/@vue/runtime-core/dist/runtime-core.cjs.js:6063:5\r\n ❯ eval node_modules/@vue/runtime-core/dist/runtime-core.cjs.js:1613:9\r\n\r\nThis error originated in \"test/app.nuxt.test.ts\" test file. It doesn't mean the error was thrown inside the file itself, but while it was running.\r\nThe latest test that might've caused the error is \"Should mount\". It might mean one of the following:\r\n- The error was thrown, while Vitest was running this test.\r\n- This was the last recorded test before the error was thrown, if error originated after test finished its execution.\r\n⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯\r\n\r\n```\r\n```\r\n",[2017],{"name":1985,"color":1986},684,"mountSuspended fails: 'set' on proxy: trap returned falsish for property 'error'","2025-03-14T15:14:45Z","https://github.com/nuxt/test-utils/issues/684",0.76193255,{"description":2024,"labels":2025,"number":2033,"owner":1991,"repository":1992,"state":1993,"title":2034,"updated_at":2035,"url":2036,"score":2037},"### Environment\n\nN/A\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\nN/A\n\n### Reproduction\n\nhttps://ui.nuxt.com/components/dashboard-sidebar#control-open-state\n\n### Description\n\n\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2026,2027,2030],{"name":1985,"color":1986},{"name":2028,"color":2029},"documentation","0075ca",{"name":2031,"color":2032},"v3","49DCB8",3561,"Cloudflare error in docs","2025-03-17T14:40:36Z","https://github.com/nuxt/ui/issues/3561",0.76541394,{"description":2039,"labels":2040,"number":2044,"owner":1991,"repository":2045,"state":2046,"title":2047,"updated_at":2048,"url":2049,"score":2050},"## Description\r\n\r\niconify's `getIcon` uses `validateIconName` which enforce some validation for an icon's name. \r\n\r\nOne validation is that a name must be split with `-` which is often not the case with users custom icons\r\n\r\n\r\n## Reproduction \r\n\r\n\r\nhttps://stackblitz.com/edit/nuxt-starter-qlykyd?file=icons%2FEuro.svg,nuxt.config.ts,app.vue\r\n\r\nin this reproduction, we can't see `my-icons:Euro` because Euro is in PascalCase\r\n\r\n## Solution \r\n\r\nEither a documentation fix about naming convention or fix it in iconify or we could also merge prefix with the name ? ",[2041],{"name":2042,"color":2043},"upstream","B4199A",265,"icon","closed","Custom icons must be in kebab case","2024-12-10T07:10:48Z","https://github.com/nuxt/icon/issues/265",0.72744256,{"description":2052,"labels":2053,"number":2054,"owner":1991,"repository":2055,"state":2046,"title":2056,"updated_at":2057,"url":2058,"score":2059},"Going on this Page: https://nuxt.com/docs/getting-started/configuration and clicking on \"nuxt.config.ts\" results in a 404\r\nalso going to https://nuxt.com/docs/guide and going to nuxt.config.ts via the directory structure also returns into 404.",[],1391,"nuxt.com","nuxt config returning 404","2023-10-25T09:32:28Z","https://github.com/nuxt/nuxt.com/issues/1391",0.7413292,{"description":2061,"labels":2062,"number":2077,"owner":1991,"repository":1991,"state":2046,"title":2078,"updated_at":2079,"url":2080,"score":2081},"### Environment\r\n\r\n- Operating System: `Darwin`\r\n- Node Version: `v18.15.0`\r\n- Nuxt Version: `3.2.3`\r\n- Nitro Version: `2.3.2`\r\n- Package Manager: `npm@9.6.2`\r\n- Builder: `vite`\r\n- User Config: `-`\r\n- Runtime Modules: `-`\r\n- Build Modules: `-`\r\n\r\n\r\n### Reproduction\r\n\r\nI cannot reproduce it in small scale and ofc also cannot share our project code...\r\nI hope someone other will have similar problem and be able to reproduce in from scratch.\r\n\r\n### Describe the bug\r\n\r\n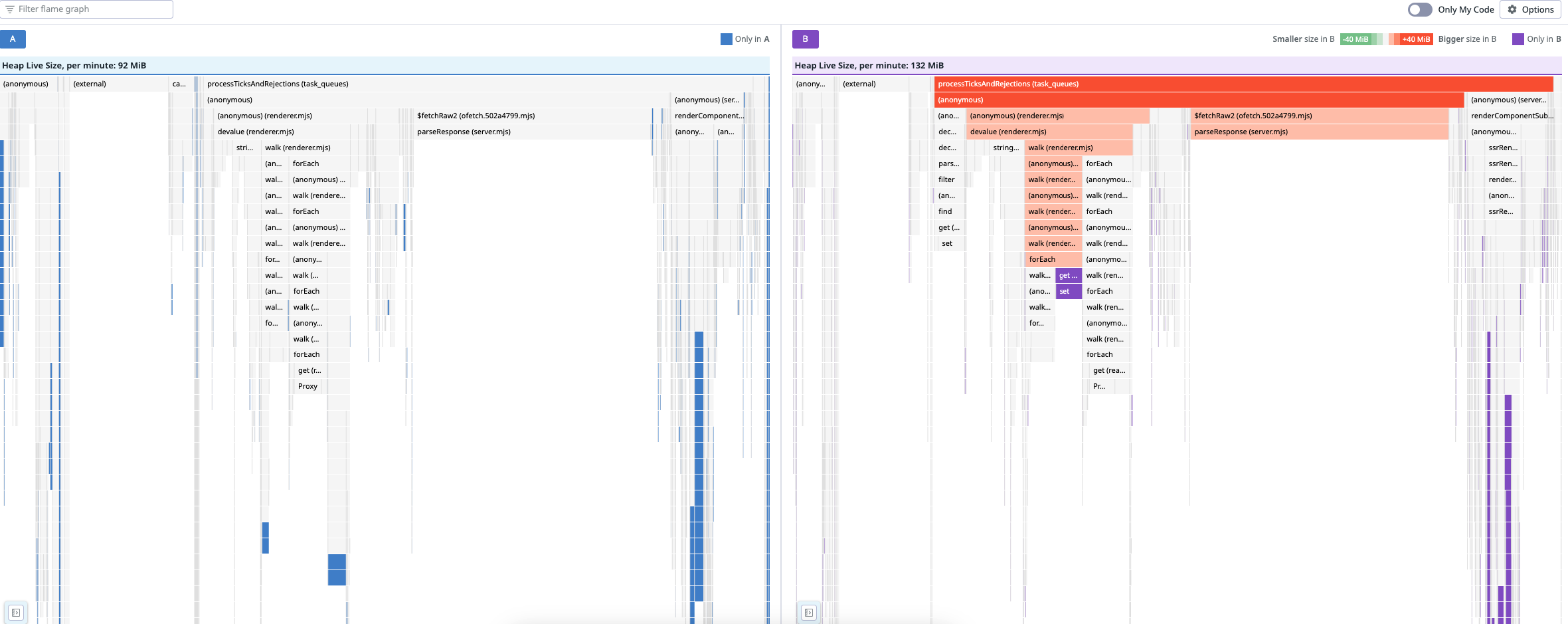\r\n\r\n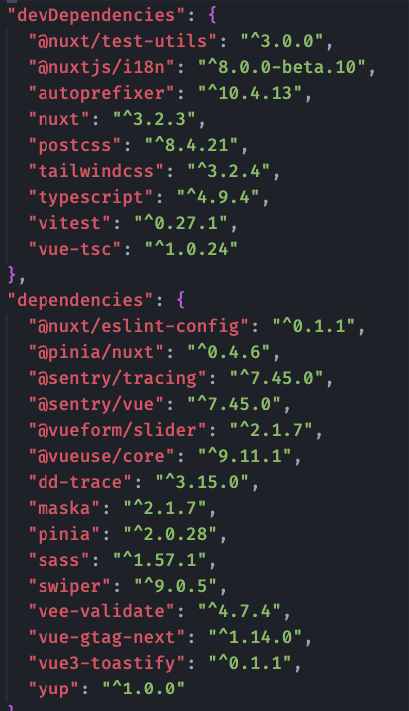\r\n\r\n\r\n\r\n### Additional context\r\n\r\nWe disabled all plugins and unnecessary third party libraries.\r\nI was also trying the solution from this issue:\r\nhttps://github.com/unjs/destr/issues/9\r\nI passed a parseResponse option to useFetch but it didn't change anything.\r\n\r\n_No response_\r\n\r\n### Logs\r\n\r\n_No response_",[2063,2066,2068,2071,2074],{"name":2064,"color":2065},"3.x","29bc7f",{"name":2006,"color":2067},"E99695",{"name":2069,"color":2070},"needs reproduction","FBCA04",{"name":2072,"color":2073},"❗ p4-important","D93F0B",{"name":2075,"color":2076},"performance","E84B77",20056,"Server Memory leaks, high CPU usage - appConfig","2023-05-05T09:54:30Z","https://github.com/nuxt/nuxt/issues/20056",0.74950516,{"description":2083,"labels":2084,"number":2089,"owner":1991,"repository":1992,"state":2046,"title":2090,"updated_at":2091,"url":2092,"score":2093},"### Environment\n\n- OS : Windows 11\n- Node : 20.14.0 \n- Nuxt: lastest\n- Nuxt prisma : lastest\n\n### Version\n\n2.19.1\n\n### Reproduction\n\ninit lastest nuxt 3\nadd nuxt prisma module\nadd nuxt ui module\n\n` ERROR RollupError: node_modules/@prisma/nuxt/dist/runtime/server/utils/prisma.d.ts (2:8): Expected ';', '}' or \u003Ceof> (Note that you need plugins to import files that are not JavaScript) nitro 21:27:12 \n\n\n1: import { PrismaClient } from \"@prisma/client\";\n2: declare const prismaClientSingleton: () => PrismaClient\u003Cimport(\".prisma/client\").Prisma.PrismaClientOptions, never, i...\n ^\n3: declare const prisma: PrismaClient\u003Cimport(\".prisma/client\").Prisma.PrismaClientOptions, never, import(\"@prisma/client...\n4: export type CustomPrismaClient = ReturnType\u003Ctypeof prismaClientSingleton>;`\n\n### Description\n\nwhen remove `'@nuxt/ui'` from modules: [''] in nuxt.config.ts it's work can use npm run dev\nbut if add `'@nuxt/ui'` it''s error\n\n` ERROR RollupError: node_modules/@prisma/nuxt/dist/runtime/server/utils/prisma.d.ts (2:8): Expected ';', '}' or \u003Ceof> (Note that you need plugins to import files that are not JavaScript) nitro 21:27:12 \n\n\n1: import { PrismaClient } from \"@prisma/client\";\n2: declare const prismaClientSingleton: () => PrismaClient\u003Cimport(\".prisma/client\").Prisma.PrismaClientOptions, never, i...\n ^\n3: declare const prisma: PrismaClient\u003Cimport(\".prisma/client\").Prisma.PrismaClientOptions, never, import(\"@prisma/client...\n4: export type CustomPrismaClient = ReturnType\u003Ctypeof prismaClientSingleton>;`\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2085,2086],{"name":1985,"color":1986},{"name":2087,"color":2088},"duplicate","cfd3d7",2813,"nuxt/ui conflict with nuxt/prisma","2024-12-04T08:33:58Z","https://github.com/nuxt/ui/issues/2813",0.75039035,{"description":2095,"labels":2096,"number":2100,"owner":1991,"repository":2055,"state":2046,"title":2101,"updated_at":2102,"url":2103,"score":2104},"- [ ] Invisible contrast https://www.notion.so/nuxt/Invisible-contrast-172c713f4cea43dc875fab68a76825be\n- [ ] Strange hover https://www.notion.so/nuxt/Strange-hover-5bb97015d8fc468485b7209d6f03bea8\n- [x] Broken images https://www.notion.so/nuxt/Broken-images-6b8c9cb921d34d3db8f27d9f5f36190a",[2097],{"name":2098,"color":2099},"needs refinement","A2C2AE",1092,"[Review] Prose","2023-10-10T14:45:05Z","https://github.com/nuxt/nuxt.com/issues/1092",0.75727105,{"description":2106,"labels":2107,"number":2100,"owner":1991,"repository":2009,"state":2046,"title":2109,"updated_at":2110,"url":2111,"score":2104},"### Environment\n\n------------------------------\n- Operating System: Linux\n- Node Version: v20.15.1\n- Nuxt Version: 3.15.2\n- CLI Version: 3.20.0\n- Nitro Version: 2.10.4\n- Package Manager: pnpm@8.7.4\n- Builder: -\n- User Config: compatibilityDate, devtools, modules\n- Runtime Modules: @nuxt/test-utils/module@3.15.4\n- Build Modules: -\n------------------------------\n\n### Reproduction\n\nhttps://stackblitz.com/~/github.com/romhml/nuxt-test-repro\n\n### Describe the bug\n\nHi! There seems to be an issue when using `mountSuspended` on a component with `defineOptions({ inheritAttrs: false })` where attributes bound using `v-bind=\"$attrs\"` are missing.\n\nFor instance with this component:\n\n``` vue\n\u003Ctemplate>\n \u003Cdiv>\n \u003Cbutton v-bind=\"$attrs\"> \u003Cslot /> \u003C/button>\n \u003C/div>\n\u003C/template>\n\n\u003Cscript setup lang=\"ts\">\ndefineOptions({ inheritAttrs: false })\n\u003C/script>\n```\n\nThe first test below using `mountSuspended` will fail because the attribute is missing, whereas the test using ` mount` from ` @vue/test-utils` works as expected.\n\n``` ts\nimport { test, expect } from 'vitest'\nimport { mount } from '@vue/test-utils'\nimport { mountSuspended } from '@nuxt/test-utils/runtime'\nimport ExampleComponent from '~/app/components/ExampleComponent.vue'\n\ntest('with mountSuspended', async () => {\n const wrapper = await mountSuspended(ExampleComponent, { attrs: { 'aria-label': 'Aria Label' } })\n expect(wrapper.find('[aria-label=\"Aria Label\"]').exists()).toBe(true)\n})\n\n\ntest('with mount', () => {\n const wrapper = mount(ExampleComponent, { attrs: { 'aria-label': 'Aria Label' } })\n expect(wrapper.find('[aria-label=\"Aria Label\"]').exists()).toBe(true)\n})\n```\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2108],{"name":2006,"color":2007},"`mountSuspended` fails to pass attributes when `inheritAttrs` is false","2025-02-01T13:02:07Z","https://github.com/nuxt/test-utils/issues/1092",["Reactive",2113],{},["Set"],["ShallowReactive",2116],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"JDJWepao-7LUgJ9XVtGwYCqUjtdifG1cug3bdzmjk_o":-1},"/nuxt/test-utils/591"]