\r\n\r\nself.addEventListener(\r\n 'message',\r\n (\r\n evt: MessageEvent\u003C{\r\n message: string\r\n }>,\r\n ) => {\r\n const { message } = evt.data\r\n const greeting = `Hello ${upperCase(message)} from assets!`\r\n\r\n postMessage({ greeting })\r\n },\r\n)\r\n```\r\n\r\nCreate a app.vue file\r\n\r\n```vue\r\n\u003Cscript setup lang=\"ts\">\r\nimport Runner from './utils/workers/index?worker'\r\n\r\nconst message = ref('Nuxt')\r\n\r\nfunction sayHello(from: 'workers' | 'composable') {\r\n if (from === 'workers') {\r\n const worker = new Runner()\r\n worker.postMessage({ message: message.value })\r\n worker.addEventListener(\r\n 'message',\r\n (\r\n evt: MessageEvent\u003C{\r\n greeting: string\r\n }>,\r\n ) => {\r\n const { greeting } = evt.data\r\n alert(greeting)\r\n },\r\n )\r\n } else {\r\n sayHelloFromComposable(message.value)\r\n }\r\n}\r\n\u003C/script>\r\n\r\n\u003Ctemplate>\r\n \u003Ch1>Demo with auto imports\u003C/h1>\r\n \u003CCustomInput v-model=\"message\" />\r\n \u003Cbutton type=\"submit\" @click=\"sayHello('composable')\">\r\n Hello from composable\r\n \u003C/button>\r\n \u003Cbutton type=\"submit\" @click=\"sayHello('workers')\">Hello from workers\u003C/button>\r\n\u003C/template>\r\n./assets/workers\r\n```\r\n\r\n### Describe the bug\r\n\r\n## Actual Behavior\r\n\r\nIn development mode, the utils/ directory is auto imported, so the upperCase function is available on the assets/index.ts file.\r\n\r\nIn production mode, we have this error:\r\n\r\n```txt\r\nUncaught ReferenceError: upperCase is not defined\r\n at index-8e797b43.js:1:88\r\n```\r\n\r\n## Expected Behavior\r\n\r\nThis should work with the same behavior in development and production mode.\r\n\r\n\r\n### Additional context\r\n\r\n\r\nI call the utils to function in `worker` file\r\n\r\n### Logs\r\n\r\n_No response_",[3019,3022,3025,3028],{"name":3020,"color":3021},"workaround available","11376d",{"name":3023,"color":3024},"bug","d73a4a",{"name":3026,"color":3027},"vite","3574D1",{"name":3029,"color":3030},"🍰 p2-nice-to-have","0E8A16",24590,"nuxt","open","Utils folder is not auto imported on production mode when using in worker file","2024-11-19T11:52:00Z","https://github.com/nuxt/nuxt/issues/24590",0.74350524,{"description":3039,"labels":3040,"number":3043,"owner":3032,"repository":3044,"state":3045,"title":3046,"updated_at":3047,"url":3048,"score":3049},"Repro :\n\n- Chose E-commerce\n- Chose Asia\n- Chose North America",[3041],{"name":3023,"color":3042},"ff281a",1166,"nuxt.com","closed","[Agencies] Facets filters not filtering correctly","2023-02-15T12:31:13Z","https://github.com/nuxt/nuxt.com/issues/1166",0.7099239,{"description":3051,"labels":3052,"number":3056,"owner":3032,"repository":3032,"state":3045,"title":3057,"updated_at":3058,"url":3059,"score":3060},"### Environment\r\n\r\nWorking directory: C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer 17:54:12\r\nNuxt project info: 17:54:12\r\n\r\n------------------------------\r\n- Operating System: Windows_NT\r\n- Node Version: v21.1.0\r\n- Nuxt Version: 3.13.1\r\n- CLI Version: 3.10.0\r\n- Nitro Version: 2.9.7\r\n- Package Manager: pnpm@8.14.1\r\n- Builder: -\r\n- User Config: compatibilityDate, devtools, vue, ssr\r\n- Runtime Modules: -\r\n- Build Modules: -\r\n------------------------------\r\n\r\n### Reproduction\r\n\r\nHere is reproduction repo:\r\nhttps://github.com/set5u/nuxt-worker-custom-renderer\r\n\r\n### Describe the bug\r\n\r\nWhen I try to use Vue's custom renderer API in a WebWorker, it works in dev mode but doesn't work when I generate it.\r\n\r\n### Additional context\r\n\r\n_No response_\r\n\r\n### Logs\r\n\r\n```shell-script\r\nC:\\Users\\vtvsn\\Documents\\.node_projects\\nuxt-worker-custom-renderer>nuxi generate\r\nNuxt 3.13.1 with Nitro 2.9.7 17:52:14\r\nℹ Using Nitro server preset: static 17:52:14\r\nℹ Building client... 17:52:17\r\nℹ vite v5.4.5 building for production... 17:52:17\r\nℹ ✓ 107 modules transformed. 17:52:18 \r\n\r\n ERROR x Build failed in 789ms 17:52:18 \r\n\r\n\r\n[17:52:18] ERROR Nuxt Build Error: [vite:worker] [plugin vite:build-import-analysis] utils/SomeComponent.vue (2:51): Failed to parse source for import analysis because the content contains invalid JS syntax. Install @vitejs/plugin-vue to handle .vue files.\r\nfile: C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/utils/Worker.ts?worker:2:51\r\n\r\n1: \u003Ctemplate>\r\n2: \u003Ccustom-renderer-element>\u003C/custom-renderer-element>\r\n ^\r\n3: \u003C/template>\r\n4: \u003Cscript setup lang=\"ts\">\r\n\r\n\r\n file: C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/utils/Worker.ts?worker:2:51\r\n\r\n 1: \u003Ctemplate>\r\n 2: \u003Ccustom-renderer-element>\u003C/custom-renderer-element>\r\n ^\r\n 3: \u003C/template>\r\n 4: \u003Cscript setup lang=\"ts\">\r\n\r\n at getRollupError (/C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/node_modules/.pnpm/rollup@4.21.3/node_modules/rollup/dist/es/shared/parseAst.js:392:41)\r\n at error (/C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/node_modules/.pnpm/rollup@4.21.3/node_modules/rollup/dist/es/shared/parseAst.js:388:42)\r\n at Object.error (/C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/node_modules/.pnpm/rollup@4.21.3/node_modules/rollup/dist/es/shared/node-entry.js:19734:20)\r\n at Object.error (/C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/node_modules/.pnpm/rollup@4.21.3/node_modules/rollup/dist/es/shared/node-entry.js:18854:42)\r\n at Object.transform (/C:/Users/vtvsn/Documents/.node_projects/nuxt-worker-custom-renderer/node_modules/.pnpm/vite@5.4.5/node_modules/vite/dist/node/chunks/dep-CUvs3bbV.js:64586:14)\r\n```",[3053],{"name":3054,"color":3055},"pending triage","E99695",29006,"Importing .vue file in WebWorker causes error in nuxi generate","2024-09-16T03:02:53Z","https://github.com/nuxt/nuxt/issues/29006",0.7107706,{"description":3062,"labels":3063,"number":3072,"owner":3032,"repository":3032,"state":3045,"title":3073,"updated_at":3074,"url":3075,"score":3076},"### Environment\n\n------------------------------\r\n- Operating System: `Windows_NT`\r\n- Node Version: `v19.7.0`\r\n- Nuxt Version: `3.3.3`\r\n- Nitro Version: `2.3.2`\r\n- Package Manager: `npm@9.6.0`\r\n- Builder: `vite`\r\n------------------------------\n\n### Reproduction\n\nhttps://stackblitz.com/edit/github-7tssgl?file=package.json\r\n\r\nI created close enought example as my app looking. So you need to `build & preview` it to see the error.\n\n### Describe the bug\n\nI'm trying to use webworkers according to vite documntation https://vitejs.dev/guide/features.html#import-with-query-suffixes\r\n\r\nAnd it looked smoothly, before `3.3.3`. Right now the whole app not working with `npm run build` - `npm run preview`.\r\nBut it works normally with `dev`.\r\n\r\nI mean it builds without error and start normally, no errors eather. But at any page of the app looks like chunk imports broken or something like that.\r\n\r\nFor example I/m importing `myWorker` at `/profile` - page of the app. But never the less at `/home` page still be broken app\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[3064,3067,3068,3069],{"name":3065,"color":3066},"3.x","29bc7f",{"name":3054,"color":3055},{"name":3026,"color":3027},{"name":3070,"color":3071},"upstream","E8A36D",20191,"Import Web Workers with query suffixes break app in production","2023-04-20T14:41:07Z","https://github.com/nuxt/nuxt/issues/20191",0.7143424,{"description":3078,"labels":3079,"number":3083,"owner":3032,"repository":3084,"state":3045,"title":3085,"updated_at":3086,"url":3087,"score":3088},"### 🐛 The bug\n\nOn **google-tag-manager** page the content isn't rendered but is present in (main) code.\n\n- https://scripts.nuxt.com/scripts/tracking/google-tag-manager\n- https://github.com/nuxt/scripts/blob/main/docs/content/scripts/tracking/google-tag-manager.md\n\n### 🛠️ To reproduce\n\nhttps://scripts.nuxt.com/scripts/tracking/google-tag-manager\n\n### 🌈 Expected behavior\n\nDisplay text content.\n\n### ℹ️ Additional context\n\n_No response_",[3080],{"name":3081,"color":3082},"documentation","0075ca",430,"scripts","Website: Callout component not displaying content","2025-03-13T05:07:49Z","https://github.com/nuxt/scripts/issues/430",0.7186866,{"description":3090,"labels":3091,"number":3094,"owner":3032,"repository":3032,"state":3045,"title":3095,"updated_at":3096,"url":3097,"score":3098},"### Environment\n\n------------------------------\r\n- Operating System: Windows_NT\r\n- Node Version: v20.9.0\r\n- Nuxt Version: 3.8.2\r\n- CLI Version: 3.10.0\r\n- Nitro Version: 2.8.0\r\n- Package Manager: pnpm@8.3.1\r\n- Builder: -\r\n- User Config: alias, app, components, devtools, experimental, modules, nitro, runtimeConfig, session, sourcemap, typescript, vite, vue\r\n- Runtime Modules: @unocss/nuxt@0.57.7, @vueuse/nuxt@10.6.1\r\n- Build Modules: -\r\n------------------------------\n\n### Reproduction\n\nhttps://stackblitz.com/edit/github-6zhzee?file=services%2Fdemo%2Fworker.ts,pages%2Findex.vue\n\n### Describe the bug\n\nIn the webworker file, the referenced Auto-imports function will not be packaged, and will appear \"Uncaught (in promise) ReferenceError: log is not defined\r\n at globalThis.onmessage (worker-6dbb1795.js:1:255)\r\n\"\n\n### Additional context\n\nstep:\r\n1. pnpm build\r\n2. node .output/...\r\n3. see browser devtools console\n\n### Logs\n\n_No response_",[3092,3093],{"name":3065,"color":3066},{"name":3054,"color":3055},24443,"Auto-imports in webworker bundle not work","2023-12-10T20:47:40Z","https://github.com/nuxt/nuxt/issues/24443",0.7194553,{"description":3100,"labels":3101,"number":3109,"owner":3032,"repository":3110,"state":3045,"title":3111,"updated_at":3112,"url":3113,"score":3114},"### Environment\n\n- Operating System: Linux\n- Node Version: v20.17.0\n- Nuxt Version: ^3.13.0\n- CLI Version: 3.14.0\n- Package Manager: bun\n- Nuxt Ui: ^3.0.0-alpha.6\n\n\n### Version\n\n3.0.0-alpha.6\n\n### Reproduction\n\n1. Clone https://github.com/malte-baumann/z-index\n2. bun dev (or similar)\n3. Open modal / slideover / drawer\n4. Click on Select / DropdownMenu / InputMenu\n5. See what you don't see\n\n### Description\n\nI opend #2404 recently and noticed some more z-index problems. \nAt least these Components: \n- Select\n- DropdownMenu\n- InputMenu \ndon't have high enough z-indexes inside a Drawer / Modal / Slideover to show their popover contents. \n\n\n\n### Additional context\n\n\n\n\n### Logs\n\n_No response_",[3102,3103,3106],{"name":3023,"color":3024},{"name":3104,"color":3105},"duplicate","cfd3d7",{"name":3107,"color":3108},"v3","49DCB8",2421,"ui","Z-Indexes inside Modal / Slideover / Drawer","2024-10-21T10:15:59Z","https://github.com/nuxt/ui/issues/2421",0.72250086,{"description":3116,"labels":3117,"number":3119,"owner":3032,"repository":3044,"state":3045,"title":3120,"updated_at":3121,"url":3122,"score":3123},"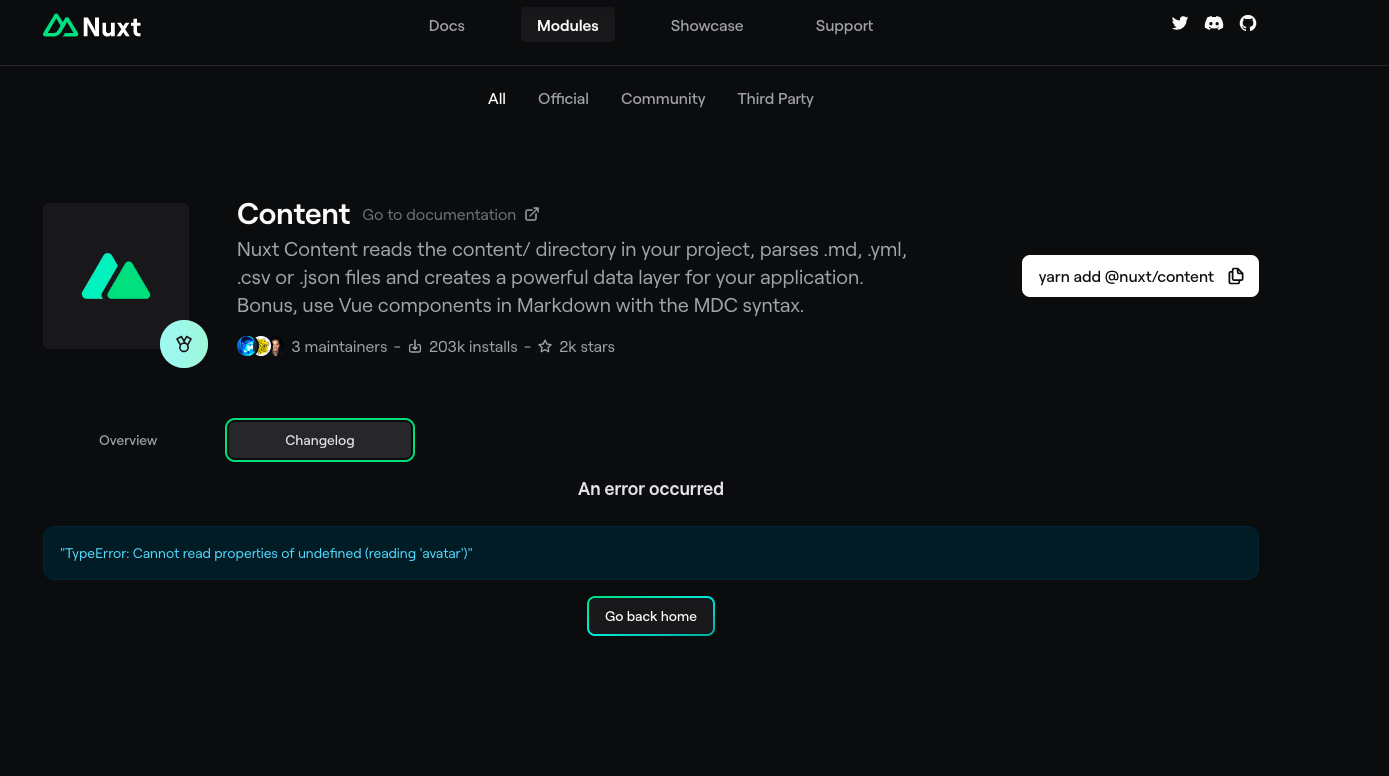\nSeems to have something to do with avatar ?",[3118],{"name":3023,"color":3042},980,"[Modules/:slug/changelog] Error on client-side navigation","2023-06-06T12:14:19Z","https://github.com/nuxt/nuxt.com/issues/980",0.73550975,{"description":3125,"labels":3126,"number":3129,"owner":3032,"repository":3032,"state":3045,"title":3130,"updated_at":3131,"url":3132,"score":3133},"### Environment\n\n```\r\n[11:21:41 AM] RootDir: C:/code/bad-imports\r\n[11:21:42 AM] Nuxt project info: \r\n\r\n------------------------------\r\n- Operating System: Windows_NT\r\n- Node Version: v16.19.1\r\n- Nuxt Version: 3.6.4\r\n- Nitro Version: 2.5.2\r\n- Package Manager: npm@8.19.3\r\n- Builder: vite\r\n- User Config: devtools\r\n- Runtime Modules: -\r\n- Build Modules: -\r\n------------------------------\r\n```\n\n### Reproduction\n\nhttps://github.com/joel-wenzel/bad-imports\r\n\r\n1. Clone repo\r\n2. Open app.vue\r\n3. Hit ctrl+space on the HelloWorld component to bring up the import suggestion\r\n4. Notice the invalid absolute path suggestion of `pages/..` instead of `./pages/..`\r\n\r\n\r\nIf you delete the nuxt tsconfig it then properly suggests a relative path\n\n### Describe the bug\n\nSince somewhere around the 3.6.0 release the import path suggestions in vs code do not seem to be valid in most cases. \r\n\r\n\r\nIn the above image it is suggesting `pages/hello/HelloWorld.vue`. At runtime this fails with \r\n```\r\n[11:25:05 AM] ERROR Failed to resolve import \"pages/hello/HelloWorld.vue\" from \"app.vue\". Does the file exist?\r\n```\r\n\r\nPre 3.6.0 it would suggest `./pages/hello/HelloWorld.vue` which would be valid.\n\n### Additional context\n\nI believe some changes were done to the `.nuxt/tsconfig.json` that are causing the issue. Namely the path aliases now use an absolute path instead of relative ( I think that was done to remove some of the issues around vue 3.3 external prop type references ).\n\n### Logs\n\n_No response_",[3127,3128],{"name":3065,"color":3066},{"name":3054,"color":3055},22228,"Bad import path suggestions since 3.6.0","2023-07-19T21:15:53Z","https://github.com/nuxt/nuxt/issues/22228",0.7407029,{"description":3135,"labels":3136,"number":3141,"owner":3032,"repository":3110,"state":3045,"title":3142,"updated_at":3143,"url":3144,"score":3145},"### Description\n\nHi everyone,\n\nI’ve been stuck for hours and can’t seem to figure out the issue. Maybe someone here has an idea of what’s going wrong:\n\nI’m starting a fresh new project using the latest versions of NUXT UI Pro, and I’m trying to get it to work with PostCSS and Less (I also tried SCSS -> same issue). Things seem to work generally, but I’m not getting any live updates in the browser when I modify a .less file and having the development server activated — the changes only appear after fully restarting the NUXT dev server.\n\nHas anyone successfully set up a similar configuration using the latest NUXT UI Pro together with PostCSS and Tailwind CSS v4+? What am I missing?\n\nHere is a demo: https://codesandbox.io/p/devbox/k6f4tk\n(try to edit /styles/globals/defaults.less -> nothing happens 😩)",[3137,3140],{"name":3138,"color":3139},"question","d876e3",{"name":3107,"color":3108},3898,"NUXT UI 3 with PostCSS - no live updates possible?","2025-04-23T10:53:15Z","https://github.com/nuxt/ui/issues/3898",0.7429584,["Reactive",3147],{},["Set"],["ShallowReactive",3150],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fKnMymdSUGjQRlgY-s5XLB_EXi9OefsoQAtP76V6-nbA":-1},"/nuxt/test-utils/795"]