\r\n\u003C/template>\r\n\r\n\u003Cscript setup lang=\"ts\">\r\nimport { DatePicker as VCalendarDatePicker } from 'v-calendar'\r\nimport type { DatePickerDate } from 'v-calendar/dist/types/src/use/datePicker'\r\nimport 'v-calendar/dist/style.css'\r\n\r\nconst props = defineProps({\r\n modelValue: {\r\n type: Date as PropType\u003CDatePickerDate>,\r\n default: null\r\n }\r\n})\r\n\r\nconst emit = defineEmits(['update:model-value', 'close'])\r\n\r\nconst date = computed({\r\n get: () => props.modelValue,\r\n set: (value) => {\r\n emit('update:model-value', value)\r\n emit('close')\r\n }\r\n})\r\n\r\nconst attrs = {\r\n transparent: true,\r\n borderless: true,\r\n color: 'primary',\r\n 'is-dark': { selector: 'html', darkClass: 'dark' },\r\n 'first-day-of-week': 1\r\n}\r\n\u003C/script>\r\n```\r\n\r\nAnd I use this component like this:\r\n```vue\r\n\u003Ctemplate>\r\n \u003CPagesForm\r\n :title=\"title\"\r\n :action=\"action\"\r\n @submit=\"formRef.submit()\"\r\n :submit-button-label=\"submitButtonLabel\"\r\n >\r\n \u003CUForm\r\n :validate=\"validate\"\r\n :state=\"state\"\r\n class=\"flex w-full flex-col gap-8\"\r\n @error=\"onError\"\r\n @submit=\"onSubmit\"\r\n ref=\"formRef\"\r\n >\r\n \u003CUFormGroup label=\"Name\" name=\"name\" required>\r\n \u003CUInput placeholder=\"Enter member name...\" size=\"xl\" v-model=\"state.name\" />\r\n \u003C/UFormGroup>\r\n\r\n \u003CUFormGroup label=\"Email\" name=\"email\" required>\r\n \u003CUInput placeholder=\"Enter member email...\" size=\"xl\" v-model=\"state.email\" />\r\n \u003C/UFormGroup>\r\n\r\n \u003CUFormGroup label=\"School\" name=\"school\" required>\r\n \u003CUInput placeholder=\"Enter member school...\" size=\"xl\" v-model=\"state.school\" />\r\n \u003C/UFormGroup>\r\n\r\n \u003CUFormGroup label=\"Position\" name=\"position\" required>\r\n \u003CUSelect\r\n placeholder=\"Select\"\r\n size=\"xl\"\r\n v-model=\"state.position\"\r\n :options=\"positionOptions\"\r\n />\r\n \u003C/UFormGroup>\r\n\r\n \u003CUFormGroup label=\"Joined At\" name=\"joinedAt\" required>\r\n \u003CUPopover :popper=\"{ placement: 'bottom-start' }\" class=\"w-full\">\r\n \u003CUButton\r\n icon=\"i-heroicons-calendar-days\"\r\n :label=\"format(state.joinedAt || new Date(), 'd MMM, yyy')\"\r\n color=\"white\"\r\n class=\"w-full\"\r\n size=\"xl\"\r\n />\r\n\r\n \u003Ctemplate #panel=\"{ close }\">\r\n \u003CCommonDatePicker v-model=\"state.joinedAt\" @close=\"close\" />\r\n \u003C/template>\r\n \u003C/UPopover>\r\n \u003C/UFormGroup>\r\n \u003C/UForm>\r\n \u003C/PagesForm>\r\n\u003C/template>\r\n\r\n\u003Cscript setup lang=\"ts\">\r\nimport { format } from 'date-fns'\r\nimport type { FormSubmitEvent, FormError, FormErrorEvent } from '#ui/types'\r\nimport { positionOptions } from '~/constants/select-options/positions'\r\nimport type { MemberForm, MemberFormState } from '~/types/members'\r\n\r\nconst props = defineProps\u003CMemberForm>()\r\n\r\nconst formRef = ref()\r\n\r\nconst state = reactive\u003CMemberFormState>({\r\n name: props.form?.name || '',\r\n email: props.form?.email || '',\r\n school: props.form?.school || '',\r\n position: props.form?.position || '',\r\n joinedAt: props.form?.joinedAt || new Date(),\r\n gen: props.form?.gen || undefined,\r\n aboutThisMember: props.form?.aboutThisMember || '',\r\n facebook: props.form?.facebook || '',\r\n github: props.form?.github || '',\r\n linkedin: props.form?.linkedin || ''\r\n})\r\n\r\nconst validate = (state: MemberFormState): FormError[] => {\r\n const errors = []\r\n if (!state.name) errors.push({ path: 'name', message: 'Required' })\r\n if (!state.email) errors.push({ path: 'email', message: 'Required' })\r\n if (!state.school) errors.push({ path: 'school', message: 'Required' })\r\n if (!state.position) errors.push({ path: 'position', message: 'Required' })\r\n if (!state.joinedAt) errors.push({ path: 'joinedAt', message: 'Required' })\r\n if (!state.gen) errors.push({ path: 'gen', message: 'Required' })\r\n if (!state.aboutThisMember) errors.push({ path: 'aboutThisMember', message: 'Required' })\r\n if (!state.facebook) errors.push({ path: 'facebook', message: 'Required' })\r\n if (!state.github) errors.push({ path: 'joinedAt', message: 'Required' })\r\n if (!state.linkedin) errors.push({ path: 'linkedin', message: 'Required' })\r\n return errors\r\n}\r\n\r\nconst onError = async (event: FormErrorEvent) => {\r\n const element = document.getElementById(event.errors[0].id)\r\n element?.focus()\r\n element?.scrollIntoView({ behavior: 'smooth', block: 'center' })\r\n}\r\n\r\nconst emit = defineEmits\u003C{\r\n (e: 'form-submit', data: object): void\r\n}>()\r\n\r\nconst onSubmit = async (event: FormSubmitEvent\u003CMemberFormState>) => {\r\n emit('form-submit', event.data)\r\n}\r\n\u003C/script>\r\n```\r\n\r\n\r\nWhen I click the button, the DatePicker is shown but the label of the Button is not change. I still don't know that caused by the date is not changed or I set the label of the button wrongly. But when I put a `console.log` inside the `set()`, it does not run.\r\n\r\n\r\n### Additional context\r\n\r\n_No response_\r\n\r\n### Logs\r\n\r\n_No response_",[1984,1987],{"name":1985,"color":1986},"bug","d73a4a",{"name":1988,"color":1989},"triage","ffffff",2106,"nuxt","ui","open","Need help: `DatePicker` set value not work","2024-11-11T16:09:38Z","https://github.com/nuxt/ui/issues/2106",0.7119853,{"description":1999,"labels":2000,"number":2007,"owner":1991,"repository":2008,"state":1993,"title":2009,"updated_at":2010,"url":2011,"score":2012},"we'd love to have the nuxt.com docs available in translations for users across the world\n\nalthough this has been a plan for some time, and was indeed the case in nuxt v2, we're still not there with v3 docs\n\nRelated issues: https://github.com/nuxt/nuxt/issues/19926, https://github.com/nuxt/nuxt/issues/21926, https://github.com/nuxt/nuxt/discussions/16054, https://github.com/nuxt/nuxt.com/issues/1448 and https://github.com/nuxt/translations/discussions/4 (private repo - relevant content copied into this issue).\n\n## Key requirements\n\n- needs to be performant and not regress performance of the documentation\n- translations need to remain always in sync, falling back to english text if required\n- needs to allow community contribution\n\n## Translation and sync automation\n\nDocumentation sources currently come from https://github.com/nuxt/nuxt.com, https://github.com/nuxt/nuxt and https://github.com/nuxt/examples. upstream changes need to be localisable, and trigger call for updates for translators, without blocking the documentation.\n\nIt would be nice to consider exploring LLM triggers for translation (see for example the way https://github.com/formkit uses ai in the process of building their documentation website) - at least for 'fallback' content.\n\n👉 See https://github.com/nuxt/nuxt/discussions/29949 for a proposal\n\n## Building a team\n\nWe've had many offers of help for translating the docs, and we'll need to build a team - but first we need to add the infrastructure to make updating translations possible.\n\nSo initially I would love for someone or a small group to take on the task of building up the automation + integration into the [nuxt/nuxt.com](https://github.com/nuxt/nuxt.com) repository, before we move ahead to create a bigger team of translators.",[2001,2004],{"name":2002,"color":2003},"enhancement","1ad6ff",{"name":2005,"color":2006},"roadmap","ff7a1a",1711,"nuxt.com","internationalisation for nuxt.com","2025-01-18T03:00:02Z","https://github.com/nuxt/nuxt.com/issues/1711",0.7125821,{"description":2014,"labels":2015,"number":2016,"owner":1991,"repository":2008,"state":1993,"title":2017,"updated_at":2018,"url":2019,"score":2020},"Hi team, \r\n\r\nThis time i founded this vulnerability in your website: \r\nhttps://nuxtjs.org/\r\n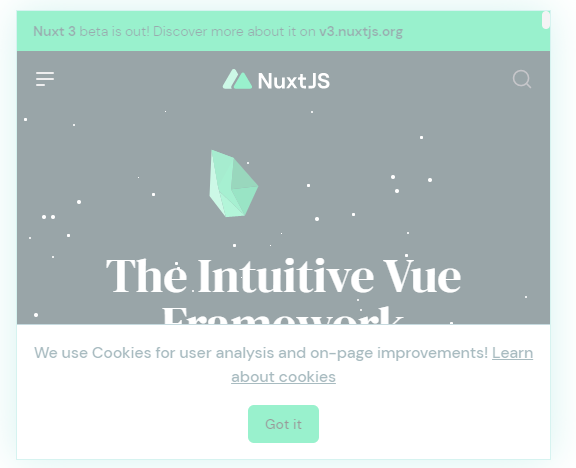\r\n\r\n\r\nClick jacking (User Interface redress attack, UI redress attack, UI redressing) is a malicious technique of tricking a Web user into clicking on something different from what the user perceives they are clicking on, thus potentially revealing confidential information or taking control of their computer while clicking on seemingly innocuous web pages.\r\n\r\nThe server didn't return an X-Frame-Options header which means that this website could be at risk of a click jacking attack. The X-Frame-Options HTTP response header can be used to indicate whether or not a browser should be allowed to render a page in a \u003Cframe> or \u003Ciframe>. Sites can use this to avoid click jacking attacks, by ensuring that their content is not embedded into other sites.\r\n\r\nThis vulnerability affects Web Server.\r\n\r\nHere are the steps to reproduce the vulnerability:\r\n\r\n1.open notepad and paste the following code.\r\n\u003C!DOCTYPE HTML>\r\n\u003Chtml lang=\"en-US\">\r\n\u003Chead>\r\n\u003Cmeta charset=\"UTF-8\">\r\n\u003Ctitle>i Frame\u003C/title>\r\n\u003C/head>\r\n\u003Cbody>\r\n\u003Ch3>This is clickjacking vulnerable\u003C/h3>\r\n\u003Ciframe src=\" https://nuxtjs.org/ \" frameborder=\"2 px\" height=\"500px\" width=\"500px\">\u003C/iframe>\r\n\u003C/body>\r\n\u003C/html>\r\n\r\n2.save it as \u003Canyname>.html eg s.html\r\n\r\n3.and just simply open that...\r\n\r\nOR\r\nCopy the link below and paste on your updated browser (Chrome,Firefox).\r\nhttps://clickjacker.io/test?url=https://nuxtjs.org/\r\nAs far as i know this data is enough to prove that your site is vulnerable to Click jacking\r\naccording to OWASP its more than enough.\r\n\r\nhttps://www.owasp.org/index.php/Testing_for_Clickjacking_(OWASP-CS-004) \r\n\r\nSOLUTION: \r\n\r\nhttps://www.owasp.org/index.php/Clickjacking_Defense_Cheat_Sheet\r\n\r\nCheck this out and here is the solution for that.\r\n \r\n\r\nI Hope that you will fix this issue as soon as possible. Looking forward to hear from you. Thank you\r\n\r\nSincerely,\r\nHassan Raza\r\n",[],1456,"Vulnerability type Click Jacking","2023-12-27T17:14:15Z","https://github.com/nuxt/nuxt.com/issues/1456",0.719642,{"description":2022,"labels":2023,"number":2026,"owner":1991,"repository":1992,"state":1993,"title":2027,"updated_at":2028,"url":2029,"score":2030},"### Environment\n\n- Operating System: Darwin\r\n- Node Version: v22.4.0\r\n- Nuxt Version: 3.12.4\r\n- CLI Version: 3.13.1\r\n- Nitro Version: -\r\n- Package Manager: pnpm@9.4.0\r\n- Builder: -\r\n- User Config: compatibilityDate, devtools, future, experimental, app, site, sitemap, routeRules, css, colorMode, runtimeConfig, modules, postcss, typescript\r\n- Runtime Modules: nuxt-headlessui@1.2.0, @nuxt/eslint@0.3.13, @nuxt/ui@2.18.4, @pinia/nuxt@0.5.4, @pinia-plugin-persistedstate/nuxt@1.2.1, @nuxt/scripts@0.8.4, nuxt-anchorscroll@1.0.3, @nuxtjs/seo@2.0.0-rc.21\r\n- Build Modules: -\n\n### Version\n\nv2.18.4\n\n### Reproduction\n\nhttps://ui.nuxt.com/components/command-palette#usage\n\n### Description\n\nOn mobile devices, when the modal is positioned at the bottom of the screen, the Command Palette becomes obscured by the virtual keyboard. This issue occurs when the Command Palette contains fewer than 6 elements or none at all, making it completely inaccessible for users.\r\n\r\nThe issue can be reproduced on the official component page: \r\n[https://ui.nuxt.com/components/command-palette](https://ui.nuxt.com/components/command-palette)\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2024,2025],{"name":1985,"color":1986},{"name":1988,"color":1989},2157,"Command Palette appears behind virtual keyboard when inside Modal on mobile devices","2024-09-23T03:49:22Z","https://github.com/nuxt/ui/issues/2157",0.721442,{"description":2032,"labels":2033,"number":2041,"owner":1991,"repository":1992,"state":2042,"title":2043,"updated_at":2044,"url":2045,"score":2046},"### Environment\n\n- Operating System: Darwin\n- Node Version: -\n- Nuxt Version: -\n- CLI Version: -\n- Nitro Version: -\n- Package Manager: -\n- Builder: -\n- User Config: -\n- Runtime Modules: -\n- Build Modules: -\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\n3.16.1\n\n### Reproduction\n\nhttps://dashboard-template.nuxt.dev/\n\nhttps://github.com/user-attachments/assets/c7c84465-1507-4ba8-a321-1e4f7def60f1\n\n### Description\n\nI was checking the pro dashboard template and found that.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2034,2035,2038],{"name":1985,"color":1986},{"name":2036,"color":2037},"v3","49DCB8",{"name":2039,"color":2040},"pro","5BD3CB",3713,"closed","Command behind Slideover in Nuxt UI Pro Template","2025-03-28T15:16:02Z","https://github.com/nuxt/ui/issues/3713",0.6823801,{"description":2048,"labels":2049,"number":2051,"owner":1991,"repository":1992,"state":2042,"title":2052,"updated_at":2053,"url":2054,"score":2055},"### Environment\n\n- Operating System: Darwin\n- Node Version: v22.10.0\n- Nuxt Version: 3.12.1\n- CLI Version: 3.15.0\n- Nitro Version: 2.10.4\n- Package Manager: yarn@1.22.22\n- Builder: -\n- User Config: default\n- Runtime Modules: @nuxt/ui@2.19.2, @vueuse/nuxt@10.11.1, @nuxtjs/eslint-module@4.1.0, @nuxtjs/stylelint-module@5.2.0, @nuxt/icon@1.7.5, @formkit/nuxt@1.6.9, @pinia/nuxt@0.4.11, @trandaison/nuxt-3-auth@0.1.4, @nuxtjs/i18n@8.5.6, notivue/nuxt, @nuxtjs/google-fonts@3.2.0, vue3-perfect-scrollbar/nuxt\n- Build Modules: -\n\n### Version\n\nv2.19.2\n\n### Reproduction\n\nhttps://stackblitz.com/edit/nuxt-ui-dj7gbt?file=app.vue\n\n\n\n### Description\n\nSlots for [selected columns](https://ui.nuxt.com/components/table#select-data), so #select-data, don't work at all.\n\nWhile it is not really clear in documentation if we should do anything more, whatever I did and tried, overriding the columns for selection simply doesn't work.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2050],{"name":1985,"color":1986},2758,"Table select slots not working","2025-01-13T16:32:33Z","https://github.com/nuxt/ui/issues/2758",0.68341905,{"description":2057,"labels":2058,"number":2062,"owner":1991,"repository":2008,"state":2042,"title":2063,"updated_at":2064,"url":2065,"score":2066},"### Environment\n\nNot needed\n\n### Reproduction\n\nNot necessary, any SSR/Prerender Nuxt will fail\n\n### Describe the bug\n\nI'm just creating this issue to warn anyone that is using Cloudflare with Nuxt SSR\r\n\r\nDISABLE ASSET MINIFICATION, it breaks Vue hydration\r\n\r\n\r\nI've spent weeks trying to fix it, and it was as simple as unchecking these boxes, hope it helps anyone\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2059],{"name":2060,"color":2061},"documentation","9DE2BA",1457,"SSR Issues with Coudflare Pages (SOLVED)","2023-12-27T17:11:13Z","https://github.com/nuxt/nuxt.com/issues/1457",0.69547814,{"description":2068,"labels":2069,"number":2076,"owner":1991,"repository":1992,"state":2042,"title":2077,"updated_at":2078,"url":2079,"score":2080},"### Environment\n\n```\nNuxt project info: 4:13:17 PM\n\n------------------------------\n- Operating System: Linux\n- Node Version: v20.16.0\n- Nuxt Version: 3.13.2\n- CLI Version: 3.14.0\n- Nitro Version: 2.9.7\n- Package Manager: pnpm@9.7.1\n- Builder: -\n- User Config: -\n- Runtime Modules: -\n- Build Modules: -\n------------------------------\n```\n\n### Version\n\n2.18.7\n\n### Reproduction\n\nhttps://ui.nuxt.com/components/button\n\n### Description\n\n\nMy issue is pretty close to https://github.com/nuxt/ui/issues/468.\n\nIt's when you switch between different types of props on a component document that this issue occurs, the first time you enter it no such issue occurs.\n\n\n\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2070,2071,2073],{"name":1985,"color":1986},{"name":2060,"color":2072},"0075ca",{"name":2074,"color":2075},"duplicate","cfd3d7",2429,"DOCS: You should use slots with \u003CContentRenderer>","2024-10-21T10:20:28Z","https://github.com/nuxt/ui/issues/2429",0.7024828,{"description":2082,"labels":2083,"number":2086,"owner":1991,"repository":1992,"state":2042,"title":2087,"updated_at":2088,"url":2089,"score":2090},"### Description\n\nI need to create custom components including @nuxt/ui components and forward props, emits on this components.\n\nWhen importing (in vue file)\n```ts\nimport type { FormFieldProps, FormFieldSlots } from '@nuxt/ui';\n\nconst props = defineProps\u003CFormFieldProps & { operations?: T[] }>();\nconst slots = defineEmits\u003CFormFieldSlots>();\n\nconst formFieldProps = reactiveOmit(props, ['operations']);\n```\nI receive this error on execution :\n```log\nIdentifier 'appConfig' has already been declared.\n\nInternal server error: Identifier 'appConfig' has already been declared. (105:6) 09:22:04\n Plugin: vite:vue\n File: .../app/pages/ResDossier/components/MyCustomComponent.vue:105:6\n at toParseError (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/parse-error.ts:95:45)\n at raise (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/tokenizer/index.ts:1497:19)\n at checkRedeclarationInScope (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/util/scope.ts:155:19)\n at declareName (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/util/scope.ts:109:12)\n at declareName (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/plugins/typescript/scope.ts:89:11)\n at declareNameFromIdentifier (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/parser/lval.ts:739:16)\n at checkIdentifier (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/parser/lval.ts:734:12)\n at checkLVal (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/parser/lval.ts:636:12)\n at parseVarId (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/parser/statement.ts:1605:10)\n at parseVarId (.../node_modules/.pnpm/@babel+parser@7.26.5/node_modules/@babel/parser/src/plugins/typescript/index.ts:3463:13)\n```\n\nIs there another way to import theses types ?",[2084,2085],{"name":1985,"color":1986},{"name":2036,"color":2037},3124,"how to import type xxxProps / xxxEmits / xxxSlots","2025-01-27T12:26:22Z","https://github.com/nuxt/ui/issues/3124",0.70321995,{"description":2092,"labels":2093,"number":2099,"owner":1991,"repository":1992,"state":2042,"title":2100,"updated_at":2101,"url":2102,"score":2103},"### Environment\n\nBuild error on vercel.app and on local windows 10, both on build and dev.\n\n### Version\n\nv1.4.4 @nuxt/ui-pro - Saas Template\n\n### Reproduction\n\nInstall a clean saas template.\nEnable typescript check in nuxt.config\n\nTry to deploy it on vercel.\n\nAnd try to copy/paste the url in a facebook post.\n\nHere is a 100% clean saas template, without typeCheck enabled:\nhttps://stackblitz.com/~/github.com/LutherApp/codespace-project\n\n(This is a copy of my [github repo](https://github.com/LutherApp/codespace-project))\n\n### Description\n\nOn build there will be \n## errors on the \"title\"-variable\nError-msg:\nObject literal may only specify known properties, and 'title' does not exist in type 'OgImageOptions\u003C\"NuxtSeo\"> | OgImagePrebuilt'. (etc.)\n\n## \"authors\"- and \"title\"-variable in blog/index\n3 x Type 'bla bla bla' is not assignable to type 'bla bla bla'.\n1 x Property 'avatar' is optional in type 'bla bla bla' but required in type 'bla bla bla'.\n\n## Fix\nI was adding this workaround for typecheck in three or four files:\n```\n// @ts-expect-error Object literal may only specify known properties, and 'title' does not exist in type 'OgImageOptions\u003C\"NuxtSeo\"> | OgImagePrebuilt'.\n```\n\nIn blog/index.vue a was adding this lines in the template in front of `UPageBody` (in blog/index.vue):\n``` \n\u003C!--\n @vue-expect-error The variable authors throws four errors;\n 3 x Type 'bla bla bla' is not assignable to type 'bla bla bla'.\n 1 x Property 'avatar' is optional in type 'bla bla bla' but required in type 'bla bla bla'.\n -->\n```\n\n## seo info from the page missing on facebook\nThere is still noe data about the page on facebook. \n(Other nuxt-content I have made earlier have this info when I copy/paste the url to facebook.)\n\n## Any questions to this issue?\nPlease add some questions to get more info about this issues. (This was written faster than normal.)",[2094,2095,2096],{"name":1985,"color":1986},{"name":2039,"color":2040},{"name":2097,"color":2098},"upstream","78bddb",2415,"og:fields in my 100% clean saas template doesn't shows on facebook, and your own public saas template don't show the og:image","2024-10-22T09:40:37Z","https://github.com/nuxt/ui/issues/2415",0.714807,["Reactive",2105],{},["Set"],["ShallowReactive",2108],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"0CsLnXkSk8dqkhofYvaWp4wmKHEhA6XMepWdz0HekUs":-1},"/nuxt/ui/2078"]