\r\n \u003C/div>\r\n\u003C/template>\r\n\u003Cscript setup lang=\"ts\">\r\nwithDefaults(\r\n defineProps\u003C{\r\n variant: \"fill\" | \"ghost\";\r\n }>(),\r\n { variant: \"fill\" }\r\n);\r\n\u003C/script>\r\n```\r\n\r\n`~/.nuxt/components.d.ts`\r\n\r\n```TypeScript\r\ndeclare module 'vue' {\r\n export interface GlobalComponents {\r\n //...\r\n 'MyButton': typeof import(\"../../src/runtime/components/MyButton.vue\")['default']\r\n //...\r\n }\r\n}\r\n\r\nexport const MyButton: typeof import(\"../../src/runtime/components/MyButton.vue\")['default']\r\n\r\nexport const componentNames: string[]\r\n```\r\n\r\n\r\n### Correct Intellisense\r\n\r\nVsCode popopver hovering `'MyButton'` in `~/.nuxt/components.d.ts`\r\n\r\n```TypeScript\r\n(property) GlobalComponents['MyButton']: __VLS_WithTemplateSlots\u003CDefineComponent\u003C{\r\n variant: {\r\n type: PropType\u003C\"fill\" | \"ghost\">;\r\n required: true;\r\n default: string;\r\n };\r\n}, {}, unknown, {}, {}, ComponentOptionsMixin, ComponentOptionsMixin, ... 5 more ..., {}>, {\r\n ...;\r\n}>\r\n````\r\n\r\n### Wrong Intellisense\r\n\r\nVsCode popopver hovering `\u003CMyButton />` in the template block of `~/app.vue` (or any other template)\r\n\r\n```TypeScript\r\n(property) 'MyButton': DefineComponent\u003C{}, {}, any>\r\n```\r\n\r\n### Additional context\r\n\r\nThe above example is the current state of the reproduction repo. But a different, but similar behavior happened in other reports (for example a yarn workspace repo). Strange enough, sometimes, it works fine...\r\n\r\n### Logs\r\n\r\n_No response_",[2898,2901,2904,2907],{"name":2899,"color":2900},"types","2875C3",{"name":2902,"color":2903},"3.x","29bc7f",{"name":2905,"color":2906},"pending triage","E99695",{"name":2908,"color":2909},"upstream","E8A36D",21933,"Component appears to be untyped in template block","2023-12-12T14:36:22Z","https://github.com/nuxt/nuxt/issues/21933",0.6723591,{"labels":2916,"number":2920,"owner":2877,"repository":2877,"state":2890,"title":2921,"updated_at":2922,"url":2923,"score":2924},[2917],{"name":2918,"color":2919},"2.x","d4c5f9",4134,"TS2314: Generic type 'ComponentOptions\u003CV>' requires 1 type argument(s).","2023-01-18T20:03:58Z","https://github.com/nuxt/nuxt/issues/4134",0.67453843,{"description":2926,"labels":2927,"number":2930,"owner":2877,"repository":2878,"state":2890,"title":2931,"updated_at":2932,"url":2933,"score":2934},"\u003Cdetails>\n \u003Csummary>Environment\u003C/summary>\n\n------------------------------\n- Operating System: Linux\n- Node Version: v22.16.0\n- Nuxt Version: 3.17.4\n- CLI Version: 3.25.1\n- Nitro Version: 2.11.12\n- Package Manager: npm@11.4.1\n- Builder: -\n- User Config: modules, ssr, devtools, app, css, ui, runtimeConfig, alias, build, devServer, watch, future, experimental, compatibilityDate, nitro, vite, eslint, heyApi, i18n, icon, svgIconSprite\n- Runtime Modules: @nuxt/ui@3.1.2, @nuxt/test-utils/module@3.19.0, @nuxt/eslint@1.4.1, @pinia/nuxt@0.11.0, @vueuse/nuxt@13.2.0, @hey-api/nuxt@0.1.6, @nuxtjs/i18n@9.5.4, nuxt-svg-icon-sprite@2.0.0\n- Build Modules: -\n------------------------------\n\u003C/details>\n\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\nv3.1.2\n\n### Reproduction\n\nhttps://www.npmjs.com/package/@nuxt/ui?activeTab=code - `interface ModalSlots`\n\n### Description\n\nGreetings, thank you very much for the work you have done!\n\nUsing the example of the `UModal` component, you can see that props in scoped slots, such as `#footer=“{ close }”`, is untyped \n\u003Cdetails>\n \u003Csummary>Example\u003C/summary>\n\n \n\u003C/details>\n\nin the bundle package it can be seen that there are indeed no valid types of props in `ModalSlots` - https://www.npmjs.com/package/@nuxt/ui?activeTab=code `interface ModalSlots`, although they are present in the source files - https://github.com/nuxt/ui/blob/v3/src/runtime/components/Modal.vue#L70\n\n\nAs I understand it, it is not only `UModal`, but all components with scoped slots.",[2928,2929],{"name":2868,"color":2869},{"name":2871,"color":2872},4260,"Scoped slots are not typed","2025-06-02T09:08:41Z","https://github.com/nuxt/ui/issues/4260",0.6751212,{"labels":2936,"number":2940,"owner":2877,"repository":2877,"state":2890,"title":2941,"updated_at":2942,"url":2943,"score":2944},[2937,2938,2939],{"name":2899,"color":2900},{"name":2905,"color":2906},{"name":2918,"color":2919},10468,"Nuxt 2 Broken Typescript Typings when Specifying head","2023-03-17T12:50:05Z","https://github.com/nuxt/nuxt/issues/10468",0.6841408,{"description":2946,"labels":2947,"number":2951,"owner":2877,"repository":2877,"state":2890,"title":2952,"updated_at":2953,"url":2954,"score":2955},"### Environment\n\n- Operating System: `Darwin`\r\n- Node Version: `v16.18.1`\r\n- Nuxt Version: `3.3.2`\r\n- Nitro Version: `2.3.2`\r\n- Package Manager: `npm@8.19.2`\r\n- Builder: `vite`\r\n- User Config: `-`\r\n- Runtime Modules: `-`\r\n- Build Modules: `-`\r\n\n\n### Reproduction\n\nhttps://github.com/niklv/nuxt-component-props-def-bug\n\n### Describe the bug\n\nFor my project config typing I need to refer to typing of component props.\r\nI try extract props typing in this way. But looks like there are no props typing at all.\r\n```vue\r\n\u003Cscript lang=\"ts\" setup>\r\n// ComponentA.vue\r\nconst {propA} = defineProps\u003C{\r\n propA: string\r\n}>()\r\n\u003C/script>\r\n\r\n\u003Ctemplate>\r\n \u003Cdiv v-text=\"propA\" />\r\n\u003C/template>\r\n\r\n```\r\n\r\n```ts\r\n// config.ts\r\nimport type {ComponentA} from \"#components\";\r\nimport {ComponentOptionsBase} from \"@vue/runtime-core\";\r\n\r\n\r\ntype ComponentProps\u003CT> = T extends ComponentOptionsBase\u003Cinfer P, // Props\r\n any, // RawBindings\r\n any, // D\r\n any, // C\r\n any, // M\r\n any, // Mixin\r\n any, // Extends\r\n any> ? unknown extends P ? {} : P : {};\r\n\r\n\r\ntype CompAProps = ComponentProps\u003Ctypeof ComponentA>\r\n\r\n\r\nconst someConfig:CompAProps = {\r\n // @ts-expect-error\r\n notExistedProp: true // should be error\r\n}\r\n```\n\n### Additional context\n\n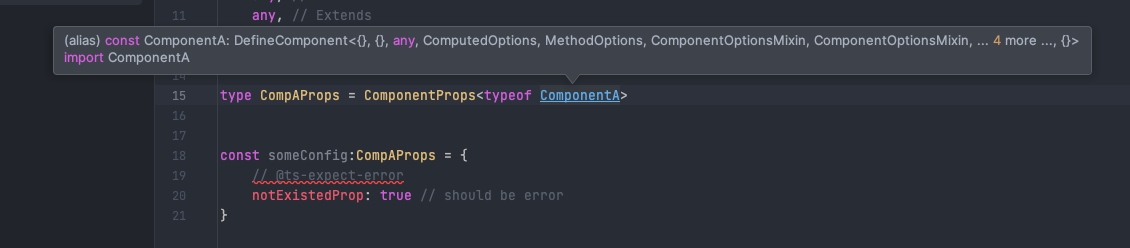\r\n\r\nThere are must be some props typing in first generic argument of `DefineComponent`\n\n### Logs\n\n_No response_",[2948,2949,2950],{"name":2899,"color":2900},{"name":2902,"color":2903},{"name":2908,"color":2909},20010,"Empty global components props typings","2023-10-22T09:01:34Z","https://github.com/nuxt/nuxt/issues/20010",0.6870058,{"description":2957,"labels":2958,"number":2965,"owner":2877,"repository":2877,"state":2890,"title":2966,"updated_at":2967,"url":2968,"score":2969},"### Environment\n\n```\r\n------------------------------\r\n- Operating System: Linux\r\n- Node Version: v16.14.2\r\n- Nuxt Version: ^3.5.2\r\n- Nitro Version: 2.4.1\r\n- Package Manager: npm@7.17.0\r\n- Builder: webpack\r\n- User Config: builder, webpack\r\n- Runtime Modules: -\r\n- Build Modules: -\r\n------------------------------\r\n```\n\n### Reproduction\n\nhttps://stackblitz.com/edit/nuxt-starter-6ue8jc?file=nuxt.config.ts\n\n### Describe the bug\n\nNuxt has [a schema](https://github.com/nuxt/nuxt/blob/main/packages/schema/src/config/webpack.ts#L154-L163) of `vue-loader` options that [partially matches](https://github.com/vuejs/vue-loader/blob/main/src/index.ts#L32-L62) `vue-loader`. \r\n\r\nFor example, you can enable experimental features like Vue 3.3 `defineModel` in `webpack.loaders.vue`, but TypeScript won't recognize it as a valid property. However, if you ignore the error squiggles, it does work.\r\n\n\n### Additional context\n\nMentioned in https://github.com/nuxt/nuxt/pull/21303#issuecomment-1574295022\n\n### Logs\n\n```shell-script\n'{ defineModel: true; }' is not assignable to type '{ productionMode?: boolean | undefined; transformAssetUrls?: { video?: string | undefined; source?: string | undefined; object?: string | undefined; embed?: string | undefined; } | undefined; compilerOptions?: { ...; } | undefined; }'.\r\n Object literal may only specify known properties, and 'defineModel' does not exist in type '{ productionMode?: boolean | undefined; transformAssetUrls?: { video?: string | undefined; source?: string | undefined; object?: string | undefined; embed?: string | undefined; } | undefined; compilerOptions?: { ...; } | undefined; }'.(2322)\n```\n",[2959,2962,2963,2964],{"name":2960,"color":2961},"good first issue","fbca04",{"name":2899,"color":2900},{"name":2902,"color":2903},{"name":2868,"color":2869},21332,"Extend vue-loader option types ","2023-06-04T22:07:55Z","https://github.com/nuxt/nuxt/issues/21332",0.68890196,{"labels":2971,"number":2977,"owner":2877,"repository":2877,"state":2890,"title":2978,"updated_at":2979,"url":2980,"score":2981},[2972,2973,2974],{"name":2902,"color":2903},{"name":2905,"color":2906},{"name":2975,"color":2976},"needs reproduction","FBCA04",23460,"TS Typing issue.","2023-10-20T22:22:28Z","https://github.com/nuxt/nuxt/issues/23460",0.6897527,{"description":2983,"labels":2984,"number":2987,"owner":2877,"repository":2877,"state":2890,"title":2988,"updated_at":2989,"url":2990,"score":2991},"### Environment\n\nOS: Windows 10\r\nNuxt: 3.5.1\r\nIDE: VSCode\n\n### Reproduction\n\nhttps://stackblitz.com/edit/github-sxsjje?file=app.vue\n\n### Describe the bug\n\nTS marks following code as error - unresolved.\r\n\r\n```vue\r\n\u003Cscript setup lang=\"ts\" generic=\"T\">\r\ndefineProps\u003C{\r\n data: T[] // unresolved\r\n}>();\r\n\u003C/script>\r\n```\r\n\r\n\r\n\n\n### Additional context\n\nLatest Volar installed\r\nLatest Volar TS plugin installed\r\n@builtin TS disabled \n\n### Logs\n\n_No response_",[2985,2986],{"name":2902,"color":2903},{"name":2905,"color":2906},21041,"Generic components | Generic type unresolved inside \u003Cscript>","2023-05-25T16:48:10Z","https://github.com/nuxt/nuxt/issues/21041",0.6918342,["Reactive",2993],{},["Set"],["ShallowReactive",2996],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fiMk5OWXlIL4wysc1qho-GHqwB3WHS6h3lMw-odxf0bU":-1},"/nuxt/ui/2102"]