\r\n \u003CAppHeader/>\r\n \r\n \u003Cdiv class=\"container\">\r\n \u003Cdiv class=\"columns\">\r\n \r\n \u003Cdiv class=\"column is-two-thirds\">\r\n \u003Cnuxt/>\r\n \u003C/div>\r\n \r\n \u003Cdiv class=\"column\">\r\n \u003Csection class=\"section\">\r\n \u003CTwitterFollowMe/>\r\n \u003Chr/>\r\n \u003Ch2 class=\"title is-2\">Derniers billets\u003C/h2>\r\n \u003CPostsSidebar :posts=\"posts\" />\r\n \u003C/section>\r\n \u003C/div>\r\n \r\n \u003C/div>\r\n \u003C/div>\r\n \u003CAppFooter />\r\n \u003C/div>\r\n\u003C/template>\r\n\r\n\u003Cscript>\r\nimport AppHeader from '~/components/AppHeader'\r\nimport AppNavigation from '~/components/AppNavigation'\r\nimport AppFooter from '~/components/AppFooter'\r\nimport TwitterFollowMe from '~/components/TwitterFollowMe'\r\nimport PostsSidebar from '~/components/PostsSidebar'\r\nimport { getPosts } from '~/services/wpContentApi'\r\n\r\nexport default {\r\n components: { AppHeader, AppNavigation, AppFooter, TwitterFollowMe, PostsSidebar },\r\n data () {\r\n return {\r\n posts: []\r\n }\r\n },\r\n async created () {\r\n this.posts = await getPosts(20)\r\n }\r\n}\r\n\u003C/script>\r\n```\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1197\">#c1197\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2042,2045],{"name":2043,"color":2044},"question","cc317c",{"name":2046,"color":2047},"2.x","d4c5f9",1351,"Server Side Rendering for components called from layouts","2023-01-18T15:41:42Z","https://github.com/nuxt/nuxt/issues/1351",0.7693778,{"description":2054,"labels":2055,"number":2057,"owner":1985,"repository":1985,"state":1987,"title":2058,"updated_at":2059,"url":2060,"score":2061},"I use external resources, I would like to know how to call a function in a component\r\n\r\n**from**\r\n` plugins: [\r\n { src: '~plugins/custom.js', ssr: false }\r\n ]\r\n`\r\n\r\n**call to**\r\n`\r\nmethods: {\r\n myfunction() { \r\n myJavascriptFunctionIntoCustomJS() \r\n }\r\n`\r\n\u003C!--\r\n🚨 IMPORTANT 🚨\r\n\r\nPlease use the following link to create a new issue:\r\n\r\n- 🚨 Bug report - https://bug.nuxtjs.org/ \r\n- 🙋 Feature request - https://feature.nuxtjs.org/ \r\n- 🤔 Help wanted - https://otechie.com/nuxt \r\n- ❗️ All other issues - https://cmty.nuxtjs.org/ \r\n\r\nIf your issue was not created using the app above, **it will be closed immediately**.\r\n\r\n🚨 注意事项 🚨\r\n\r\n请务必使用下述链接来创建 issue:\r\n\r\n- 🚨 Bug 提交 - https://bug.nuxtjs.org/ \r\n- 🙋 新功能提案 - https://feature.nuxtjs.org/ \r\n- 🤔 寻求帮助 - https://otechie.com/nuxt \r\n- ❗️ 其他问题 - https://cmty.nuxtjs.org/ \r\n\r\n如果 issue 不是通过上述链接进行创建, **该 issue 会被系统自动关闭**\r\n-->\r\n\r\n\r\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\r\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://cmty.app/nuxt\">Nuxt\u003C/a> community (\u003Ca href=\"https://cmty.app/nuxt/nuxt.js/issues/c9072\">#c9072\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2056],{"name":2046,"color":2047},5555,"How to call a javascript function from a plugin in a component","2023-01-18T20:12:56Z","https://github.com/nuxt/nuxt/issues/5555",0.7716343,{"description":2063,"labels":2064,"number":2071,"owner":1985,"repository":1985,"state":1987,"title":2072,"updated_at":2073,"url":2074,"score":2075},"### Environment\n\nrc-13\r\nnode-18\n\n### Reproduction\n\n//composable\r\n```javascript\r\nconst myfetch = async (url,options,emits) => {\r\n const { data, pending, error, refresh } = await useFetch(url, {\r\n \r\n ...options,\r\n baseURL: useRuntimeConfig().API_URL, \r\n initialCache: false,\r\n headers: new Headers({ 'authorization': (process.client)?localStorage.getItem(\"token\"):''}),\r\n onResponse ({ response }) {\r\n return response._data\r\n },\r\n onResponseError ({ response }) {\r\n return response._data\r\n }\r\n })\r\n const resData = data.value\r\n \r\n return resData\r\n }\r\n```\r\n//component 1\r\n\r\n```javascript\r\n\u003Cscript setup>\r\ngetAllItems();\r\nasync function getAllItems() {\r\n let response = await myfetch('/article/artPosts?' +\r\n 'page=' + page.value + '&' +\r\n 'artPostGroup=' + artPostGroup.value + '&' +\r\n 'limit=' + limit.value, {\r\n method: 'GET'\r\n });\r\n if (response.data)\r\n list.value = response.data.docs;\r\n pages.value = response.data.pages;\r\n}\r\n\u003C/script>\r\n```\r\n\r\n//component 2\r\n\r\n```javascript\r\n\u003Cscript setup>\r\ngetAllItems();\r\nasync function getAllItems() {\r\n let response = await myfetch('/store/storeCourses?' +\r\n 'page=' + page.value + '&' +\r\n 'storeCourseGroup=' + storeCourseGroup.value + '&' +\r\n 'limit=' + limit.value, {\r\n method: 'GET'\r\n });\r\n if (response.data)\r\n list.value = response.data.docs;\r\n pages.value = response.data.pages;\r\n}\r\n\u003C/script>\r\n```\r\n//home.vue\r\n```javascript\r\n\u003Cdiv>\r\n\u003Ccomponent1>\u003C/component1>\r\n\u003Ccomponent2>\u003C/component2>\r\n\u003C/div>\r\n```\n\n### Describe the bug\n\nthe bug is here...\r\nin the page load:\r\nresponse of component1 is the same as response of component2...\r\n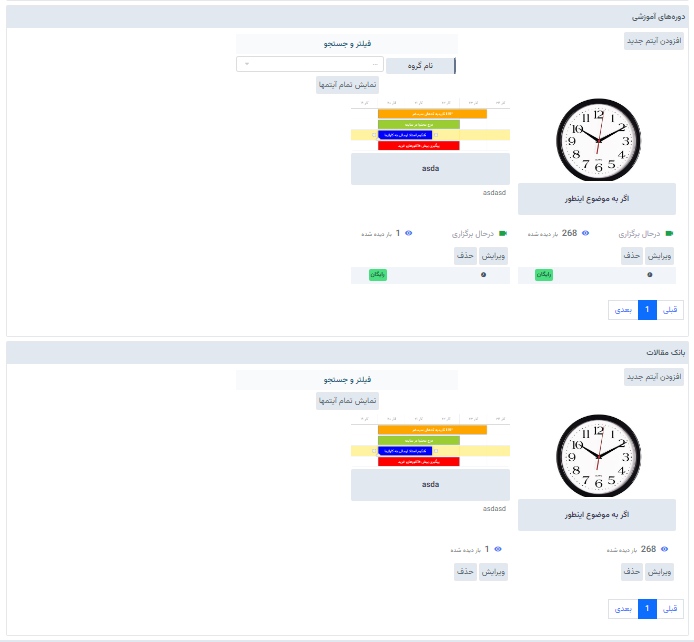\r\n\r\nwhen i use $fetch instead of useFetch, it works good but it makes another problem for me...\r\n\r\non refreshing page,it send a request twice to server\r\n\n\n### Additional context\n\nhow can i write function in composables?\n\n### Logs\n\n_No response_",[2065,2068],{"name":2066,"color":2067},"3.x","29bc7f",{"name":2069,"color":2070},"pending triage","E99695",15451,"UseFetch() calling multiple request, only the last data is fetched and $fetch send requests twice","2023-01-19T17:50:37Z","https://github.com/nuxt/nuxt/issues/15451",0.77239174,{"description":2077,"labels":2078,"number":2081,"owner":1985,"repository":1985,"state":1987,"title":2082,"updated_at":2083,"url":2084,"score":2085},"### Version\n\n[v2.3.0](https://github.com/nuxt.js/releases/tag/v2.3.0)\n\n### Reproduction link\n\n[https://codesandbox.io/s/ko1j0qryvv](https://codesandbox.io/s/ko1j0qryvv)\n\n### Steps to reproduce\n\nCreate a lodash template using a lodash function to compile a javascript file. The function does not exist. Only _.escape exists.\n\n### What is expected ?\n\nLodash functions to exist still - this is a breaking change.\n\n### What is actually happening?\n\nThe _.forEach function does not exist\n\n### Additional comments?\n\nI'm happy to pop in an alternative workaround if there is one.\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This bug report is available on \u003Ca href=\"https://cmty.app/nuxt\">Nuxt\u003C/a> community (\u003Ca href=\"https://cmty.app/nuxt/nuxt.js/issues/c8156\">#c8156\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2079,2080],{"name":2069,"color":2070},{"name":2046,"color":2047},4350,"Lodash templates no longer have lodash functions","2023-01-22T15:30:07Z","https://github.com/nuxt/nuxt/issues/4350",0.77288246,{"description":2087,"labels":2088,"number":2092,"owner":1985,"repository":2022,"state":1987,"title":2093,"updated_at":2094,"url":2095,"score":2096},"### For what version of Nuxt UI are you asking this question?\n\nv3.0.0-alpha.x\n\n### Description\n\nIs there a way we can add a custom class to the tag `\u003Cth>`, in my case I would like to place `w-28` so that it has a fixed width\n\n\n\n\n",[2089,2091],{"name":2043,"color":2090},"d876e3",{"name":2019,"color":2020},2762,"[v3] Custom class to the tag \u003Cth>","2024-12-09T11:14:42Z","https://github.com/nuxt/ui/issues/2762",0.7742469,["Reactive",2098],{},["Set"],["ShallowReactive",2101],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"obiUHHb8injSXnjWYbdSTT_mnbtW8E3Px29h_8qB-DY":-1},"/nuxt/ui/2518"]