\n \u003C/UFormGroup>\n\n \u003CUButton type=\"submit\">\n Submit\n \u003C/UButton>\n\n\u003C/UForm>\n```\n\n```ts\n\u003Cscript setup lang=\"ts\">\n import { z } from 'zod';\n\n const state = reactive({\n picture: undefined,\n })\n\n const schema = z.object({\n picture: z.custom\u003CFileList>()\n .transform((val) => {\n if (val instanceof File) return val;\n if (val instanceof FileList) return val[0];\n return null;\n })\n .superRefine((file, ctx) => {\n if (!(file instanceof File)) {\n ctx.addIssue({\n code: z.ZodIssueCode.custom,\n fatal: true,\n message: 'Not a file',\n });\n return z.NEVER;\n }\n if (file.size > 5 * 1024 * 1024) {\n ctx.addIssue({\n code: z.ZodIssueCode.custom,\n message: 'Max file size allowed is 5MB',\n });\n }\n if (\n !['image/jpeg', 'image/png', 'image/webp', 'image/jpg'].includes(\n file.type\n )\n ) {\n ctx.addIssue({\n code: z.ZodIssueCode.custom,\n message: 'File must be an image (jpeg, jpg, png, webp)',\n });\n }\n })\n });\n\n type Schema = z.infer\u003Ctypeof schema>;\n\n async function onSubmit (event: FormSubmitEvent\u003CSchema>) {\n console.log(event.data);\n }\n\u003C/script>\n```\n\nAs aspected the following onChange function logs a FileList\n\n```ts\nfunction onChangeFile(event: Event) {\n console.log(event)\n}\n```\n\nBut when I log val in the transform function of Zod\n\n```ts\n.transform((val) => {\n console.log(val);\n if (val instanceof File) return val;\n if (val instanceof FileList) return val[0];\n return null;\n})\n```\n\nI get a string like this:\n\n```\nC:\\fakepath\\Screenshot 2024-10-25 at 10.26.36.png\n```\n\nCan someone please help me out, i'm looking for a few days now for a solution.",[1984],{"name":1985,"color":1986},"question","d876e3",2462,"nuxt","ui","open","UInput with type='file', how to validate with Zod?","2025-03-10T01:51:18Z","https://github.com/nuxt/ui/issues/2462",0.74469155,{"description":1996,"labels":1997,"number":1998,"owner":1988,"repository":1999,"state":1990,"title":2000,"updated_at":2001,"url":2002,"score":2003},"Hi team, \r\n\r\nThis time i founded this vulnerability in your website: \r\nhttps://nuxtjs.org/\r\n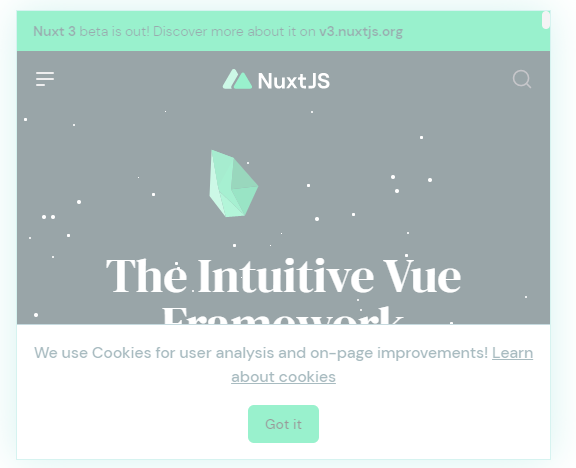\r\n\r\n\r\nClick jacking (User Interface redress attack, UI redress attack, UI redressing) is a malicious technique of tricking a Web user into clicking on something different from what the user perceives they are clicking on, thus potentially revealing confidential information or taking control of their computer while clicking on seemingly innocuous web pages.\r\n\r\nThe server didn't return an X-Frame-Options header which means that this website could be at risk of a click jacking attack. The X-Frame-Options HTTP response header can be used to indicate whether or not a browser should be allowed to render a page in a \u003Cframe> or \u003Ciframe>. Sites can use this to avoid click jacking attacks, by ensuring that their content is not embedded into other sites.\r\n\r\nThis vulnerability affects Web Server.\r\n\r\nHere are the steps to reproduce the vulnerability:\r\n\r\n1.open notepad and paste the following code.\r\n\u003C!DOCTYPE HTML>\r\n\u003Chtml lang=\"en-US\">\r\n\u003Chead>\r\n\u003Cmeta charset=\"UTF-8\">\r\n\u003Ctitle>i Frame\u003C/title>\r\n\u003C/head>\r\n\u003Cbody>\r\n\u003Ch3>This is clickjacking vulnerable\u003C/h3>\r\n\u003Ciframe src=\" https://nuxtjs.org/ \" frameborder=\"2 px\" height=\"500px\" width=\"500px\">\u003C/iframe>\r\n\u003C/body>\r\n\u003C/html>\r\n\r\n2.save it as \u003Canyname>.html eg s.html\r\n\r\n3.and just simply open that...\r\n\r\nOR\r\nCopy the link below and paste on your updated browser (Chrome,Firefox).\r\nhttps://clickjacker.io/test?url=https://nuxtjs.org/\r\nAs far as i know this data is enough to prove that your site is vulnerable to Click jacking\r\naccording to OWASP its more than enough.\r\n\r\nhttps://www.owasp.org/index.php/Testing_for_Clickjacking_(OWASP-CS-004) \r\n\r\nSOLUTION: \r\n\r\nhttps://www.owasp.org/index.php/Clickjacking_Defense_Cheat_Sheet\r\n\r\nCheck this out and here is the solution for that.\r\n \r\n\r\nI Hope that you will fix this issue as soon as possible. Looking forward to hear from you. Thank you\r\n\r\nSincerely,\r\nHassan Raza\r\n",[],1456,"nuxt.com","Vulnerability type Click Jacking","2023-12-27T17:14:15Z","https://github.com/nuxt/nuxt.com/issues/1456",0.74499506,{"description":2005,"labels":2006,"number":2016,"owner":1988,"repository":1989,"state":1990,"title":2017,"updated_at":2018,"url":2019,"score":2020},"### For what version of Nuxt UI are you suggesting this?\n\nv3.0.0-alpha.x\n\n### Description\n\nHello everyone, I’d like to discuss the possibility of setting `props` by default from `AppConfig`. Currently, it’s very convenient that we can configure the theme through the config. It would be great to have a similar option for props. Previously, @benjamincanac mentioned [here](https://github.com/nuxt/ui/issues/1289#issuecomment-2480556540) that this is not currently planned.\n\nMy proposal is simple: wrap all `withDefaults` in our own implementation. This way, we can define props without making significant changes to the code.\n\n## What it could look like\n\nLet’s look at the `Accordion.vue` component. At the moment, its props look like this:\n```ts\nconst props = withDefaults(defineProps\u003CAccordionProps\u003CT>>(), {\n type: 'single',\n collapsible: true,\n labelKey: 'label'\n})\n```\nLet’s assume our wrapper is called `withUiDefaults`. Then the code would look like this:\n```diff\n-const props = withDefaults(defineProps\u003CAccordionProps\u003CT>>(), {\n+const props = withUiDefaults(defineProps\u003CAccordionProps\u003CT>>(), {\n type: 'single',\n collapsible: true,\n labelKey: 'label'\n})\n```\nThe implementation of `withUiDefaults` is quite simple:\n```ts\nfunction withUiDefaults(props, defaults) {\n const defaultFromConfig = {}\n\n return withDefaults(props, {\n ...defaults,\n ...defaultFromConfig\n })\n}\n``` \n\n## Issues I See\n\n1) **Typing `AppConfig` might be challenging** \n We would need to somehow generate types based on the components. \n **My opinion:** I think we can retrieve this using `vue-component-meta` during the UI build stage. So, in my opinion, this isn’t a significant problem.\n\n2) **Developers might set `modelValue` or other critical props by default** \n **My opinion:** In such cases, we can log a warning to the console. Additionally, developers should understand which props they are overriding. We won’t be able to cover all edge cases, but I don’t think we need to.\n\n### Additional context\n\n_No response_",[2007,2010,2013],{"name":2008,"color":2009},"enhancement","a2eeef",{"name":2011,"color":2012},"v3","49DCB8",{"name":2014,"color":2015},"triage","ffffff",2662,"Override default props from `AppConfig`","2024-11-17T08:30:46Z","https://github.com/nuxt/ui/issues/2662",0.7491438,{"description":2022,"labels":2023,"number":2028,"owner":1988,"repository":1989,"state":2029,"title":2030,"updated_at":2031,"url":2032,"score":2033},"### Environment\n\n------------------------------\n- Operating System: Windows_NT\n- Node Version: v20.9.0\n- Nuxt Version: 3.13.1\n- CLI Version: 3.13.1\n- Nitro Version: 2.9.7\n- Package Manager: npm@10.9.0\n- Builder: -\n- User Config: devtools, modules, css, ui, colorMode, compatibilityDate, icon, sourcemap, experimental, nitro, future, pwa, routeRules, spaLoadingTemplate, runtimeConfig\n- Runtime Modules: @nuxt/ui@3.0.0-alpha.9, @vueuse/nuxt@10.11.1, @nuxtjs/mdc@0.6.1, @formkit/auto-animate/nuxt@0.8.2, @vite-pwa/nuxt@0.10.5\n- Build Modules: -\n------------------------------\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\nv3.0.0-alpha.9\n\n### Reproduction\n\n`style tag`\n\n`Use`\n\n`debug`\n\n\n\n### Description\n\nSorry, I don't know if it's a bad configuration on my part, however the styles in which I use @apply don't work for me.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2024,2027],{"name":2025,"color":2026},"bug","d73a4a",{"name":2011,"color":2012},2763,"closed","postcss style not applying","2024-12-05T13:48:59Z","https://github.com/nuxt/ui/issues/2763",0.71543294,{"description":2035,"labels":2036,"number":2042,"owner":1988,"repository":1989,"state":2029,"title":2043,"updated_at":2044,"url":2045,"score":2046},"### Description\n\nI really love all the work that went into this, but it's currently connected to vue-router for `Link` and `Pagination` components. I would love to see these become optional dependencies so it can be used with projects that do not use `vue-router`.\n\nMaybe there is some way to stub out the link components to enable support for Inertia \\ default HTML?",[2037,2038,2039],{"name":1985,"color":1986},{"name":2011,"color":2012},{"name":2040,"color":2041},"vue","42b883",3001,"How likely is it that we'll see a routerless version?","2025-04-14T08:47:28Z","https://github.com/nuxt/ui/issues/3001",0.71574146,{"description":2048,"labels":2049,"number":2052,"owner":1988,"repository":1989,"state":2029,"title":2053,"updated_at":2054,"url":2055,"score":2056},"### Description\n\nHey!\n\nIt would be nice to have the primary/secondary/neutral/etc colours defined in `app.config.ts` directly as tailwind variables (ex: text-primary-500)\n\nRight now we have to define the colours both in `app.config.ts` and `tailwind.config.js` to be able to do that. (the new `main.css` would require to create a variable for each sub-colour manually so not worth using it) \n\nFor `neutral`, since it's a tailwind colour, it could be named `text-neutr-500`\nOr maybe name all colours with something like `text-Uneutral-500`\n\n\n### Additional context\n\n_No response_",[2050,2051],{"name":2008,"color":2009},{"name":2011,"color":2012},2948,"Add theme colors as tailwind variables","2025-02-06T16:29:04Z","https://github.com/nuxt/ui/issues/2948",0.71985936,{"description":2058,"labels":2059,"number":2062,"owner":1988,"repository":1989,"state":2029,"title":2063,"updated_at":2064,"url":2065,"score":2066},"### For what version of Nuxt UI are you asking this question?\n\nv3-alpha\n\n### Description\n\nI'm experience problems with the VS Code Tailwind Intellisense plugin. I have used ui v2 without problems so far, but when using v3 it only shows suggestions when a tailwindcss.config.ts is freshly edited, but then after reloading or restarting VS Code, it doesn't work anymore. When it's working, it only shows a few of the classes, but not the most common ones like padding, margin etc..\n\nHas someone experienced the same issue and knows a fix for that? ",[2060,2061],{"name":1985,"color":1986},{"name":2011,"color":2012},2424,"V3 Intellisense not working correctly","2024-11-05T21:44:20Z","https://github.com/nuxt/ui/issues/2424",0.7292487,{"description":2068,"labels":2069,"number":2072,"owner":1988,"repository":1989,"state":2029,"title":2073,"updated_at":2074,"url":2075,"score":2076},"### Description\n\nIt would be nice to have a prop to set the `getRowId` method of `@tanstack/vue-table` to be able to maintain selection between pages.\nReference: https://tanstack.com/table/latest/docs/guide/row-selection#useful-row-ids\n\nAt the moment I bypassed this missing feature by adding the method inside one of the available options:\n\n```vue\n\u003CUTable :data :paginationOptions=\"{ manualPagination: true, rowCount: data?.totalItems, getRowId: (row: MyRowType) => row.id }\" />\n```\nbut it is not optimal as typescript is complaining about the types.\n\nI would suggest something like\n```vue\n\u003CUTable :data idKey=\"id\" :paginationOptions=\"{ manualPagination: true, rowCount: data?.totalItems }\" />\n```\n\nwhere `idKey` is a `keyof T` or it can be the entire `getRowId` method imported from TanStack.\n\nI can open a PR about this if it's fine :)\n\n### Additional context\n\n_No response_",[2070,2071],{"name":2008,"color":2009},{"name":2011,"color":2012},3255,"Unique row ID for `UTable` component","2025-02-15T17:44:03Z","https://github.com/nuxt/ui/issues/3255",0.73323095,{"description":2078,"labels":2079,"number":2085,"owner":1988,"repository":1989,"state":2029,"title":2086,"updated_at":2087,"url":2088,"score":2089},"### Environment\n\n```\n------------------------------\n- Operating System: Darwin\n- Node Version: v22.11.0\n- Nuxt Version: 3.15.0\n- CLI Version: 3.17.2\n- Nitro Version: 2.10.4\n- Package Manager: npm@10.9.0\n- Builder: -\n- User Config: default\n- Runtime Modules: @nuxt/content@2.13.4, @nuxt/eslint@0.7.4, @nuxt/fonts@0.10.3, @nuxt/image@1.8.1, @nuxt/ui@2.20.0, @nuxthq/studio@2.2.1, @vueuse/nuxt@12.2.0, nuxt-og-image@4.0.2\n- Build Modules: -\n------------------------------\n```\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\n3.15.0\n\n\n### Description\n\nHi, what did i do wrong ?\n\n\n\n```\nuser ~/d/nuxt> npx nuxi init -t github:nuxt-ui-pro/saas my-saas\n\n\n✔ Which package manager would you like to use?\nnpm\n◐ Installing dependencies... 2:59:28 PM\nnpm warn deprecated inflight@1.0.6: This module is not supported, and leaks memory. Do not use it. Check out lru-cache if you want a good and tested way to coalesce async requests by a key value, which is much more comprehensive and powerful.\nnpm warn deprecated glob@7.2.3: Glob versions prior to v9 are no longer supported\n\n> postinstall\n> nuxt prepare\n\nℹ Using default Tailwind CSS file nuxt:tailwindcss 3:00:48 PM\nℹ Nuxt Icon server bundle mode is set to local 3:00:49 PM\n[3:00:50 PM] ✔ Nuxt Icon discovered local-installed 3 collections: heroicons, simple-icons, vscode-icons\nℹ Running with compatibility version 4 3:00:50 PM\n✔ Types generated in .nuxt 3:00:50 PM\n\nadded 1277 packages, and audited 1279 packages in 1m\n\n347 packages are looking for funding\n run `npm fund` for details\n\nfound 0 vulnerabilities\n✔ Installation completed. 3:00:50 PM\n\n✔ Initialize git repository?\nYes\nℹ Initializing git repository... 3:26:30 PM\n\nInitialized empty Git repository in /Users/amine-smahi/dev/nuxt/my-saas/.git/\n 3:26:30 PM\n✨ Nuxt project has been created with the nuxt-ui-pro-saas template. Next steps:\n › cd my-saas 3:26:30 PM\n › Start development server with npm run dev 3:26:30 PM\nuser ~/d/nuxt> cd my-saas/\nuser ~/d/n/my-saas (main)> npm run dev\n\n> dev\n> nuxt dev\n\nNuxt 3.15.0 with Nitro 2.10.4 3:26:37 PM\n 3:26:37 PM\n ➜ Local: http://localhost:3000/\n ➜ Network: use --host to expose\n\n\n ╭──────────────────────────────────────────────────────────────────────────────────────────────────────╮\n │ │\n │ Nuxt UI Pro │\n │ │\n │ Missing NUXT_UI_PRO_LICENSE env variable, please add it to your .env. │\n │ │\n │ Purchase Nuxt UI Pro at https://ui.nuxt.com/pro/pricing to build your app in production. │\n │ │\n ╰──────────────────────────────────────────────────────────────────────────────────────────────────────╯\n\nℹ Using default Tailwind CSS file nuxt:tailwindcss 3:26:38 PM\n ➜ DevTools: press Shift + Option + D in the browser (v1.7.0) 3:26:39 PM\n\nℹ Nuxt Icon server bundle mode is set to local 3:26:40 PM\n[3:26:41 PM] ✔ Nuxt Icon discovered local-installed 3 collections: heroicons, simple-icons, vscode-icons\nℹ Running with compatibility version 4 3:26:41 PM\n[nuxt:tailwindcss 3:26:41 PM] ℹ Tailwind Viewer: http://localhost:3000/_tailwind/\n✔ Vite client built in 19ms 3:26:42 PM\n✔ Vite server built in 94ms 3:26:42 PM\n✔ Nuxt Nitro server built in 1353 ms nitro 3:26:44 PM\nℹ Vite client warmed up in 372ms 3:26:45 PM\nℹ Vite server warmed up in 3607ms 3:26:48 PM\n\n ERROR [nuxt] [request error] [unhandled] [500] Unexpected token ';'\n\n\n\n ERROR [nuxt] [request error] [unhandled] [500] Unexpected token ';'\n\n```\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2080,2081,2084],{"name":2025,"color":2026},{"name":2082,"color":2083},"duplicate","cfd3d7",{"name":2011,"color":2012},2991,"ERROR [nuxt] [request error] [unhandled] [500] Unexpected token ';'","2024-12-28T17:43:05Z","https://github.com/nuxt/ui/issues/2991",0.7385033,{"description":2091,"labels":2092,"number":2100,"owner":1988,"repository":1989,"state":2029,"title":2101,"updated_at":2102,"url":2103,"score":2104},"### Environment\n\n- Operating System: `Darwin`\n- Node Version: `v18.20.4`\n- Nuxt Version: `3.11.2`\n- CLI Version: `3.11.1`\n- Nitro Version: `2.9.6`\n- Package Manager: `yarn@1.22.22`\n- Builder: `-`\n- User Config: `telemetry`, `server`, `runtimeConfig`, `devtools`, `ui`, `colorMode`, `swiper`, `app`, `site`, `ssr`, `ogImage`, `googleFonts`, `typescript`, `loading`, `tailwindcss`, `build`, `vite`, `i18n`, `gtag`, `dayjs`, `prometheus`, `modules`\n- Runtime Modules: `dayjs-nuxt@2.1.9`, `@pinia/nuxt@0.5.1`, `@pinia-plugin-persistedstate/nuxt@1.2.0`, `@nuxt/ui@2.16.0`, `@nuxtjs/i18n@8.3.1`, `@nuxtjs/google-fonts@3.2.0`, `nuxt-swiper@1.2.2`, `@nuxtjs/seo@2.0.0-rc.11`, `nuxt-gtag@2.0.6`, `nuxt-meta-pixel@2.0.2`, `nuxt-easy-lightbox@1.0.2`, `@artmizu/nuxt-prometheus@2.4.0`\n- Build Modules: `-`\n\n\n### Version\n\nv2.9.0\n\n### Reproduction\n\nhttps://stackblitz.com/edit/nuxt-ui-aaekcsib?file=app.config.ts,app.vue\n\n### Description\n\nEvery time that I want to apply a backdrop filter blur to the parent of the Tooltip component, the hover effect stops working and the tooltip doesn't show up. Can someone help me on this?\n\nThanks for your attention.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2093,2094,2095,2097],{"name":2025,"color":2026},{"name":2082,"color":2083},{"name":2096,"color":2015},"wontfix-v2",{"name":2098,"color":2099},"upstream","78bddb",2883,"Tooltip: stops working after backdrop filter is applied","2024-12-19T21:10:15Z","https://github.com/nuxt/ui/issues/2883",0.7434396,["Reactive",2106],{},["Set"],["ShallowReactive",2109],{"TRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"HEjU1rSZ_szkS2lE1AmJKutzPUlGdbPbgQCPLnQK8ek":-1},"/nuxt/ui/2688"]