\r\n\t\t\t\t\u003C/nuxt-link>\r\n\t\t\t\u003C/el-dialog>\r\n\t\t\u003C/ClientOnly>\r\n```\n\n### Describe the bug\n\n打包后PM2启动后出现同一组件渲染两次的情况,我尝试删除Element的el-dialog 可以解决这个问题,但是我需要这个弹窗\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[3095,3096,3097,3098],{"name":3080,"color":3081},{"name":3066,"color":3067},{"name":3084,"color":3085},{"name":3042,"color":3043},22458,"打包后PM2启动后出现同一组件渲染两次的情况","2023-08-13T01:47:25Z","https://github.com/nuxt/nuxt/issues/22458",0.7327944,{"description":3105,"labels":3106,"number":3109,"owner":3025,"repository":3025,"state":3047,"title":3110,"updated_at":3111,"url":3112,"score":3113},"### Describe the bug\r\n\r\nNuxt 3.3 allow developers to use `useAppConfig` in Nitro environment and `server` directory.\r\n\r\n> We now support useAppConfig in nitro server routes (https://github.com/nuxt/nuxt/pull/19489) - a long-awaited change. Now useAppConfig is consistently available throughout your app for non-runtime configuration from layers, modules, etc.\r\n\r\nconsidering that, the docuemntation on [`app-config`](https://nuxt.com/docs/guide/directory-structure/app-config) is outdated.\r\n> Support for accessing useAppConfig() in Nitro and server directory is [coming soon](https://github.com/nuxt/nuxt/pull/19489) and available on [edge-channel](https://nuxt.com/docs/guide/going-further/edge-channel).\r\n\r\nthe solution would be removing this banner completely and since the previous paragraph states that:\r\n> We can universally access theme both when server-rendering the page and in the browser using [useAppConfig](https://nuxt.com/docs/api/composables/use-app-config) composable.\r\n\r\nthere would be no need to add more context.\r\n",[3107,3108],{"name":3080,"color":3081},{"name":3066,"color":3067},19816,"Outdated docs after nuxt 3.3.1 release","2023-03-20T09:25:12Z","https://github.com/nuxt/nuxt/issues/19816",0.73564243,{"description":3115,"labels":3116,"number":3119,"owner":3025,"repository":3025,"state":3047,"title":3120,"updated_at":3121,"url":3122,"score":3123},"### Environment\n\nNuxi 3.4.1 \n\n### Reproduction\n\nany page\r\n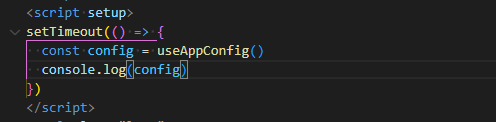\r\n\n\n### Describe the bug\n\nget error 'nuxt instance unavailable' on server side\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[3117,3118],{"name":3080,"color":3081},{"name":3066,"color":3067},20452,"useAppConfig() within setTimeout Error","2023-04-25T05:28:10Z","https://github.com/nuxt/nuxt/issues/20452",0.73807573,{"description":3125,"labels":3126,"number":3131,"owner":3025,"repository":3026,"state":3047,"title":3132,"updated_at":3133,"url":3134,"score":3135},"### For what version of Nuxt UI are you suggesting this?\n\nv2.x\n\n### Description\n\nMuch like in https://github.com/nuxt/ui/issues/1387, I have a need to listen for clicks on `\u003CUTable />` rows. `@select` brings you part of the way there, but doesn't make it easy to get access to the full underlying event, for example to check if the user is holding the \u003Ckey>Ctrl\u003C/key>.\n\nSimilarly, even with `@select` I can't see any easy way to focus the table rows using keyboard input. \n\n### Additional context\n\n_No response_",[3127,3128,3129,3130],{"name":3036,"color":3037},{"name":3039,"color":3040},{"name":3042,"color":3043},{"name":3045,"color":3043},2796,"UTable row click event","2025-06-18T09:05:27Z","https://github.com/nuxt/ui/issues/2796",0.73878497,{"description":3137,"labels":3138,"number":3143,"owner":3025,"repository":3025,"state":3047,"title":3144,"updated_at":3145,"url":3146,"score":3147},"### Environment\n\ndev\n\n### Reproduction\n\nnone\n\n### Describe the bug\n\n页面使用\u003CNuxtLayout>后在设置 keepalive: true,路由从别处跳转过来报错,在layout中能够正常跳转,非layout组件跳转过来报错\r\nTypeError: Cannot read properties of null (reading 'insertBefore')\n\n### Additional context\n\n\u003Cimg width=\"575\" alt=\"WeChat26d774ce2a549537517b76b333bc53de\" src=\"https://github.com/nuxt/nuxt/assets/48787313/d51c4464-8d4d-4b47-b77c-2290ce2e5c26\">\r\n\n\n### Logs\n\n_No response_",[3139,3140,3141,3142],{"name":3080,"color":3081},{"name":3066,"color":3067},{"name":3084,"color":3085},{"name":3042,"color":3043},22959,"nuxt3设置keepalive:true后路由跳转报错","2023-12-16T04:01:11Z","https://github.com/nuxt/nuxt/issues/22959",0.7400789,["Reactive",3149],{},["Set"],["ShallowReactive",3152],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$f40Jh0-cDtEdu3WGp6N5EqLhjHNwmaH_1L7sc5dUCR3o":-1},"/nuxt/ui/2710"]