\n\u003C/template>\n\n\u003Cscript setup>\nconst translationField = ref(null)\n\nfunction nextCard() {\n translationField.value.focus() // throws: 'focus is not a function'\n \n nextTick(() => {\n translationField.value.focus() // same error\n })\n\n // below works ok\n nextTick(() => {\n const input = translationField.value?.$el?.querySelector('input')\n if (input) {\n input.focus()\n }\n })\n}\n\u003C/script>\n```\n\n\n### Description\nStandard Vue method of using template ref (for setting a focus, here) throws: 'focus() is not a function'\nIn the above code snippet I included workaround (credits to Sonnet 3.5)\nTried it dozen of times and checked this place to see if it has not been solved earlier.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2929,2932,2935],{"name":2930,"color":2931},"bug","d73a4a",{"name":2933,"color":2934},"v3","49DCB8",{"name":2936,"color":2937},"triage","ffffff",3644,"ui","closed","\u003CUInput> template ref does not work","2025-03-21T13:14:18Z","https://github.com/nuxt/ui/issues/3644",0.70311195,{"description":2946,"labels":2947,"number":2950,"owner":2911,"repository":2939,"state":2940,"title":2951,"updated_at":2952,"url":2953,"score":2954},"### Environment\n\n-\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\nv3.16.0\n\n### Reproduction\n\n- \n\n### Description\n\nUpgraded all deps to the latest and bam Nuxt 3.16.0 is added. We get errors now as Nuxt UI 3 still ships with\n\n`'@unhead/vue': 1.11.20(vue@3.5.13(typescript@5.8.2))\n`\n\nAs of Nuxt 3.16.0 ships with @unhead2:\nhttps://nuxt.com/blog/v3-16#unhead-v2\n\nActual error:\n`[@nuxt/scripts 9:42:20 PM] ERROR Nuxt Scripts requires Unhead >= 2, you are using v1.11.20. Please run nuxi upgrade --clean to upgrade...`\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```shell-script\n\n```",[2948,2949],{"name":2930,"color":2931},{"name":2933,"color":2934},3513,"Nuxt 3.16.0 - ships with @unhead2","2025-03-10T08:49:36Z","https://github.com/nuxt/ui/issues/3513",0.70552146,{"description":2956,"labels":2957,"number":2960,"owner":2911,"repository":2961,"state":2940,"title":2962,"updated_at":2963,"url":2964,"score":2965},"(reported on discord) you can see by hard-reloading https://nuxt.com/modules?q=svg, for example.\r\n\r\nprobably similar to https://github.com/nuxt/nuxt.com/issues/1417",[2958],{"name":2930,"color":2959},"ff281a",1455,"nuxt.com","query parameters on module page result in hydration mismatch","2025-03-24T22:03:27Z","https://github.com/nuxt/nuxt.com/issues/1455",0.7128538,{"description":2967,"labels":2968,"number":2971,"owner":2911,"repository":2939,"state":2940,"title":2972,"updated_at":2973,"url":2974,"score":2975},"### Environment\n\n\n\nI get this error anytime I try and use \u003CUInput /> even without any other code.\n\n### Version\n\nv3.0.0-alpha.5\n\n### Reproduction\n\n`\u003Ctemplate>\n \u003CUContainer>\n \u003CUForm :schema=\"schema\" :state=\"state\" class=\"space-y-4\" @submit=\"onSubmit\">\n \u003CUFormField label=\"Code\" name=\"Code\"\n >\u003CUInput v-model=\"state.code\" />\n \u003C/UFormField>\n \u003C/UForm>\n \u003C/UContainer>\n\u003C/template>\n\n\u003Cscript setup lang=\"ts\">\nimport { z } from 'zod';\nimport type { FormSubmitEvent } from '#ui/types';\n\nconst supabase = useSupabaseClient();\nconst toast = useToast();\n\n//Form\nconst schema = z.object({\n code: z.string().min(3),\n});\n\ntype Schema = z.output\u003Ctypeof schema>;\n\nconst state = reactive\u003CPartial\u003CSchema>>({\n code: undefined,\n});\n\nasync function onSubmit(event: FormSubmitEvent\u003CSchema>) {\n toast.add({\n title: 'Success',\n description: 'The form has been submitted.',\n color: 'success',\n });\n console.log(event.data);\n}\n\u003C/script>\n`\n\n### Description\n\nUInput causes an error\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2969,2970],{"name":2930,"color":2931},{"name":2933,"color":2934},2349,"UInput causes error","2024-10-10T14:15:25Z","https://github.com/nuxt/ui/issues/2349",0.71349055,{"description":2977,"labels":2978,"number":2982,"owner":2911,"repository":2983,"state":2940,"title":2984,"updated_at":2985,"url":2986,"score":2987},"### 🆒 Your use case\n\nUsing the Matomo script I would like client-side navigation (through `\u003CNuxtLink>`) to send page view events to Matomo.\n\n### 🆕 The solution you'd like\n\nPerhaps only if `trackPageView` is truthy the integration hooks into the vue router to send events.\n\n### 🔍 Alternatives you've considered\n\n_No response_\n\n### ℹ️ Additional info\n\n_No response_",[2979],{"name":2980,"color":2981},"enhancement","a2eeef",360,"scripts","Matomo: track client-side navigation page views","2025-01-29T12:02:02Z","https://github.com/nuxt/scripts/issues/360",0.7176177,{"description":2989,"labels":2990,"number":2991,"owner":2911,"repository":2911,"state":2940,"title":2992,"updated_at":2993,"url":2994,"score":2995},"The previous and next links at the bottom of documentation pages disappear when navigating to other pages. As far as I know, this only occurs when Nuxt links to a page without a trailing slash when the server 301s to one with a trailing slash.\r\n\r\n**Reproduction:**\r\n\r\n1. Visit https://v3.nuxtjs.org/docs/directory-structure/components/\r\n2. Use the sidebar or previous/next links to visit the `assets` or `composables` page\r\n\r\n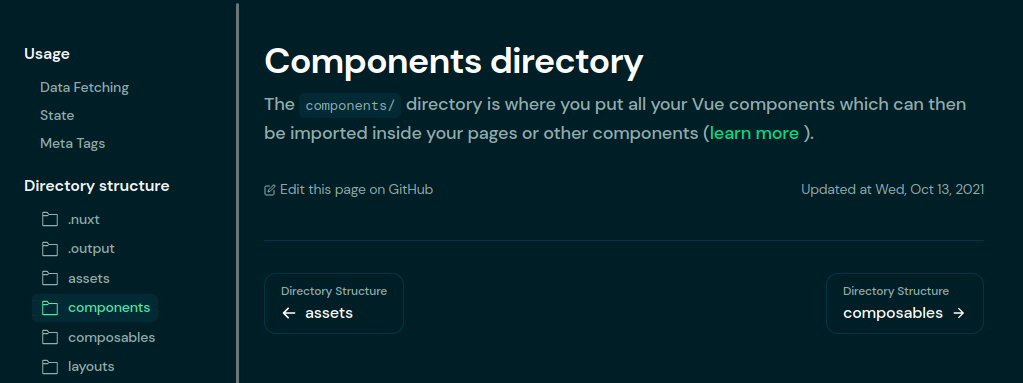\r\n\r\n3. Observe that the previous and next links are no longer present\r\n\r\n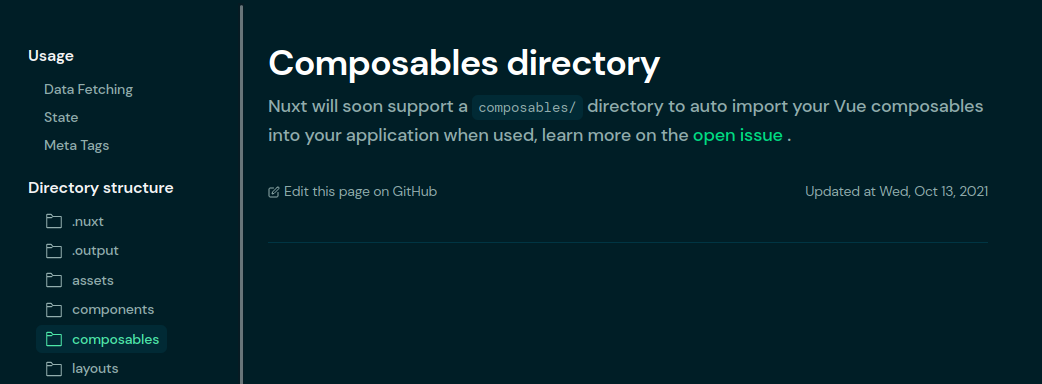\r\n\r\n**Behavior with a URL that 404s instead of 301s:**\r\n\r\n1. Visit https://v3.nuxtjs.org/docs/directory-structure/package/\r\n2. Use the sidebar or previous/next links to visit the `nuxt.config.ts` page\r\n3. Observe that the previous and next links are still present. Refresh the page manually to make them disappear",[],12019,"[Doc] PagePrevNext not rendering on page change in production","2023-01-19T15:54:17Z","https://github.com/nuxt/nuxt/issues/12019",0.7269626,{"labels":2997,"number":2998,"owner":2911,"repository":2911,"state":2940,"title":2992,"updated_at":2999,"url":3000,"score":2995},[],12119,"2023-01-19T15:59:14Z","https://github.com/nuxt/nuxt/issues/12119",{"description":2989,"labels":3002,"number":3003,"owner":2911,"repository":2911,"state":2940,"title":2992,"updated_at":3004,"url":3005,"score":2995},[],12213,"2023-01-19T16:00:22Z","https://github.com/nuxt/nuxt/issues/12213",["Reactive",3007],{},["Set"],["ShallowReactive",3010],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fmciOUKB0aM_OXxRucm0W28ez116JgclN369n_epr5d0":-1},"/nuxt/ui/3527"]