even without any other code.\n\n### Version\n\nv3.0.0-alpha.5\n\n### Reproduction\n\n`\u003Ctemplate>\n \u003CUContainer>\n \u003CUForm :schema=\"schema\" :state=\"state\" class=\"space-y-4\" @submit=\"onSubmit\">\n \u003CUFormField label=\"Code\" name=\"Code\"\n >\u003CUInput v-model=\"state.code\" />\n \u003C/UFormField>\n \u003C/UForm>\n \u003C/UContainer>\n\u003C/template>\n\n\u003Cscript setup lang=\"ts\">\nimport { z } from 'zod';\nimport type { FormSubmitEvent } from '#ui/types';\n\nconst supabase = useSupabaseClient();\nconst toast = useToast();\n\n//Form\nconst schema = z.object({\n code: z.string().min(3),\n});\n\ntype Schema = z.output\u003Ctypeof schema>;\n\nconst state = reactive\u003CPartial\u003CSchema>>({\n code: undefined,\n});\n\nasync function onSubmit(event: FormSubmitEvent\u003CSchema>) {\n toast.add({\n title: 'Success',\n description: 'The form has been submitted.',\n color: 'success',\n });\n console.log(event.data);\n}\n\u003C/script>\n`\n\n### Description\n\nUInput causes an error\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2945,2946],{"name":2930,"color":2931},{"name":2933,"color":2934},2349,"UInput causes error","2024-10-10T14:15:25Z","https://github.com/nuxt/ui/issues/2349",0.70192796,{"description":2953,"labels":2954,"number":2960,"owner":2911,"repository":2936,"state":2937,"title":2961,"updated_at":2962,"url":2963,"score":2964},"### Environment\n\n- Operating System: Windows_NT\n- Node Version: v18.20.5\n- Nuxt Version: 3.14.1592\n- CLI Version: 3.15.0\n- Nitro Version: 2.10.4\n- Package Manager: npm@10.8.2\n- Builder: -\n- User Config: default\n- Runtime Modules: nuxt-vuefire@1.0.4, @nuxt/ui@3.0.0-alpha.9\n- Build Modules: -\n\n### Is this bug related to Nuxt or Vue?\n\nNuxt\n\n### Version\n\nv3.0.0-alpha.9\n\n### Reproduction\n\n\n\n\n\n```\n \u003CUTooltip :delay-duration=\"0\" :text=\"`R$ ${transactionCost.brl}`\">\n \u003CUIcon class=\"text-yellow-500 text-xs\" name=\"i-lucide-coins\" />\n \u003Cp class=\"text-neutral-200 text-xs\">\n {{ transactionCost.credits }}\n \u003C/p>\n\u003C/UTooltip>\n```\n\n``` \n\u003CNuxtLayout>\n \u003CUApp :toaster=\"{ position: 'top-right' }\">\n \u003CNuxtPage />\n \u003C/UApp>\n\u003C/NuxtLayout>\n```\n\n\n### Description\n\nThe `app.vue` is already using the `UApp`. When I try to add a `UTooltip` inside the modal, it throw an error.\n\n### Additional context\n\n_No response_\n\n### Logs\n\n```\nUncaught (in promise) Error: Injection `Symbol(TooltipProviderContext)` not found. Component must be used within `TooltipProvider`\n```",[2955,2956,2959],{"name":2930,"color":2931},{"name":2957,"color":2958},"needs reproduction","CB47CF",{"name":2933,"color":2934},2801,"[v3][UTooltip] `UTooltip` inside `UModal` causing error","2025-02-06T15:28:30Z","https://github.com/nuxt/ui/issues/2801",0.71966195,{"labels":2966,"number":2973,"owner":2911,"repository":2911,"state":2937,"title":2974,"updated_at":2975,"url":2976,"score":2977},[2967,2970,2971],{"name":2968,"color":2969},"3.x","29bc7f",{"name":2930,"color":2931},{"name":2957,"color":2972},"FBCA04",12686,"bug on server/api side.","2023-01-19T16:09:51Z","https://github.com/nuxt/nuxt/issues/12686",0.72362036,{"description":2979,"labels":2980,"number":2984,"owner":2911,"repository":2911,"state":2937,"title":2985,"updated_at":2986,"url":2987,"score":2988},"### Environment\n\n- Operating System: `Linux`\r\n- Node Version: `v18.16.0`\r\n- Nuxt Version: `3.4.3`\r\n- Nitro Version: `2.3.3`\r\n- Package Manager: `npm@9.5.1`\r\n- Builder: `vite`\r\n- User Config: `srcDir`, `modules`, `robots`, `vite`, `i18n`, `nitro`, `telemetry`, `algolia`, `app`, `auth`, `viewport`, `css`, `build`, `runtimeConfig`\r\n- Runtime Modules: `@nuxtjs/tailwindcss@6.6.7`, `genesis-design-system/nuxt`, `@nuxtjs/algolia@1.6.0`, `formidable@2.1.1`, `nuxt-viewport@2.0.4`, `@nuxtjs/partytown@1.3.0`, `@vueuse/nuxt@9.13.0`, `nuxt-schema-org@2.1.3`, `nuxt-icon@0.1.8`, `@pinia/nuxt@0.4.9`, `@nuxtjs/i18n@8.0.0-beta.11`, `@sidebase/nuxt-auth@0.4.4`, `@nuxtjs/robots@3.0.0`\r\n- Build Modules: `-`\r\n\n\n### Reproduction\n\nI have a newDesigns object that I have in the Node part, but lose in the VUE part.\r\nIt seems that the payload is not correctly collecting the information to the VUE part (client)\r\n\r\nDebugger NODE Side\r\n\r\n\r\nFail in VUE\r\n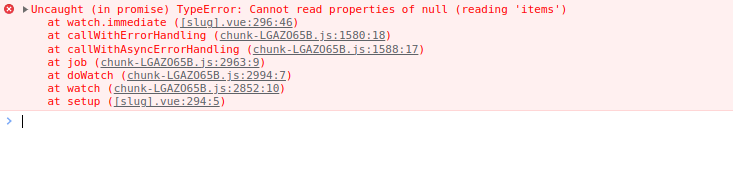\r\n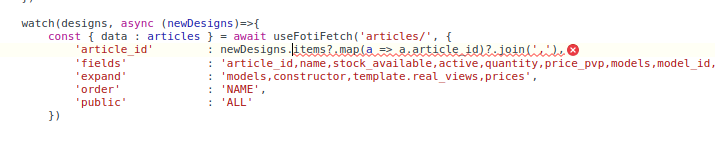\r\n\r\n\r\n\n\n### Describe the bug\n\nI have a newDesigns object that I have in the Node part, but lose in the VUE part.\r\nIt seems that the payload is not correctly collecting the information to the VUE part (client)\r\n\r\nDebugger NODE Side\r\n\r\n\r\nFail in VUE\r\n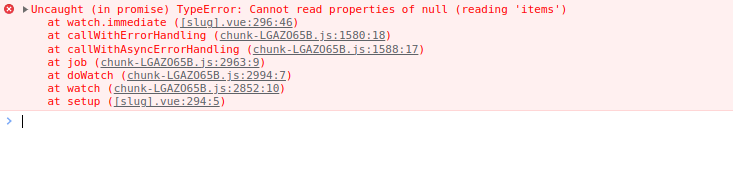\r\n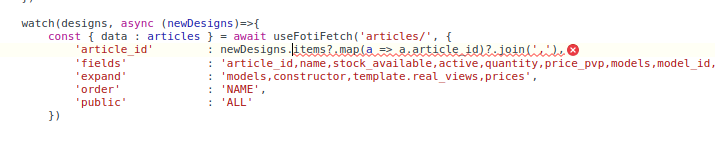\n\n### Additional context\n\n_No response_\n\n### Logs\n\n_No response_",[2981,2982,2983],{"name":2968,"color":2969},{"name":2908,"color":2909},{"name":2957,"color":2972},20630,"The values in the payload are lost in the VUE part","2023-05-09T09:37:19Z","https://github.com/nuxt/nuxt/issues/20630",0.73066723,{"description":2990,"labels":2991,"number":2992,"owner":2911,"repository":2993,"state":2937,"title":2994,"updated_at":2995,"url":2996,"score":2997},"After using `pnpm update` to upgrade vue to 3.4.3, the console throws a lot of `Hydration mismatch`. If I am using vue 3.3.13, no `Hydration mismatch` warnings will be thrown.\r\n\r\n\u003Cimg width=\"1402\" alt=\"SCR-20240103-qagg\" src=\"https://github.com/nuxt/nuxt.com/assets/133459587/188ac1c4-e19a-4570-a5d0-4863c92a7bda\">\r\n",[],1467,"nuxt.com","About vue@3.4.3 causing hydration mismatch","2024-05-03T00:34:36Z","https://github.com/nuxt/nuxt.com/issues/1467",0.7321222,{"description":2999,"labels":3000,"number":3005,"owner":2911,"repository":2936,"state":2937,"title":3006,"updated_at":3007,"url":3008,"score":3009},"### Environment\n\n- Operating System: `macos`\n- Node Version: `v18.16.0`\n- Nuxt Version: `3.16.0\"`\n- Vue: \"3.5.0\",\n- Nuxt/ui\": \"3.0.0\",\n- CLI Version: `3.8.4`\n- Package Manager: `pnpm`\n- IDE: VS Code\n\n\n### Version\n\nv3\n\n### Reproduction\n\nin any expample, even in your ui template npx nuxi init -t ui \u003Cmy-app>\n\n\n\n### Description\n\ntailwind IntelliSense works only in attribute class of elements, but not works in :ui={...}. so to write classes in ui i need learn them all, for example. \n\n\n\n\n\n\n\n\ni tried to help tailwind IntelliSense by addnig classregex to vscode setttings, but it did not help\n\n\n\njust for test purpose, i add some classregex for [CVA](https://cva.style/docs/getting-started/installation) for check if it will work. and it work for cva, not for :ui={..}\nscreen for cva\n\n\n### Additional context\n\nmake it work please! for :ui={..} and for app.config.ts\n\n### Logs\n\n```shell-script\n\n```",[3001,3002],{"name":2930,"color":2931},{"name":3003,"color":3004},"triage","ffffff",3570,"Tailwind CSS IntelliSense not works in :ui={} and in app.config.ts","2025-03-17T17:00:40Z","https://github.com/nuxt/ui/issues/3570",0.7336326,{"description":3011,"labels":3012,"number":3013,"owner":2911,"repository":2993,"state":2937,"title":3014,"updated_at":3015,"url":3016,"score":3017},"Could there be an issue with the website deployment?\n\n",[],1769,"Website documentation is outdated","2025-01-27T10:26:08Z","https://github.com/nuxt/nuxt.com/issues/1769",0.73441386,["Reactive",3019],{},["Set"],["ShallowReactive",3022],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$fixbCjDYiZeUMz8827HU2WVagA5jeqg_PnlvzrdN98jk":-1},"/nuxt/ui/3622"]