\r\n```\r\n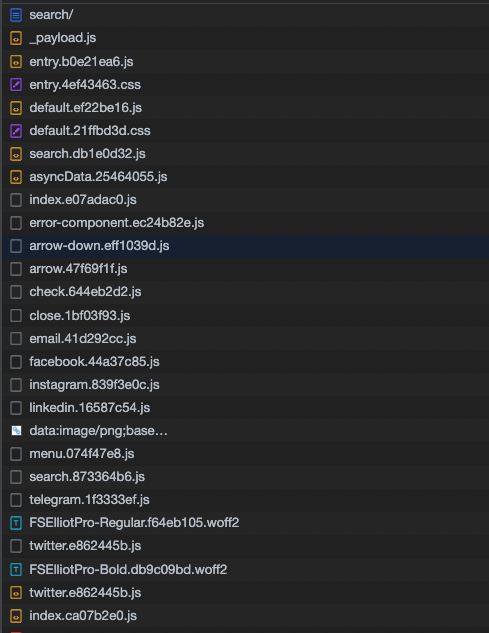\r\n\r\n## Code\r\n\r\n```\r\n\u003Ctemplate>\r\n \u003CIcon />\r\n\u003C/tempalte>\r\n\r\n\u003Cscript setup lang=\"ts\">\r\nconst props = defineProps\u003C{name: string}>()\r\n\r\nconst Icon = computed(() => defineAsyncComponent(() => import(`../icons/${props.name}.svg?component`)));\r\n\u003C/script>\r\n```\r\n\r\n\r\n\r\n",[2913,2916,2919],{"name":2914,"color":2915},"workaround available","11376d",{"name":2917,"color":2918},"❗ p4-important","D93F0B",{"name":2920,"color":2921},"performance","E84B77",18376,"Disable `prefetch` for dynamic imports","2025-03-16T23:31:44Z","https://github.com/nuxt/nuxt/issues/18376",0.7626332,{"description":2928,"labels":2929,"number":2930,"owner":2903,"repository":2904,"state":2931,"title":2932,"updated_at":2933,"url":2934,"score":2935},"There seems to be a bug that makes no sense to me. The client side `init()` in `loadIcon(name, timeout)` goes through successfully and populates the block level `dataStorage[\"\"]` with icons. \n\nNow comes the weird part: in the next line, there is a call `getIcon(name)` and as soon as I step into that function, `dataStorage` becomes \n```\n{ \n \"\": {\n prefix1: {\n icons: {} \n ...\n }\n}\n```\n\nWhen next icon gets processed, it is the same story, `_initialized` is `true`, `dataStorage[\"\"]` looks good and has all icons, but as soon as `getIcon(name)` is executed, `dataStorage` changes to \n\n```\n{\n \"\": {\n prefix1: ..., \n prefix2: ...\n }\n}\n```\n\nIt looks like there are two `dataStorage`s, one in scope of `loadIcon` and another one in scope of `getIcon`, but I have no clue how could it be prefilled with partial data...",[],267,"closed","Client bundle always falls back to Iconify API","2024-10-04T21:37:09Z","https://github.com/nuxt/icon/issues/267",0.72889304,{"labels":2937,"number":2944,"owner":2903,"repository":2903,"state":2931,"title":2945,"updated_at":2946,"url":2947,"score":2948},[2938,2941],{"name":2939,"color":2940},"enhancement","8DEF37",{"name":2942,"color":2943},"2.x","d4c5f9",8306,"建议将_NUXT_放入到localStorage中","2023-01-22T15:52:44Z","https://github.com/nuxt/nuxt/issues/8306",0.73380864,{"description":2950,"labels":2951,"number":2956,"owner":2903,"repository":2903,"state":2931,"title":2957,"updated_at":2958,"url":2959,"score":2960},"becasuse nuxt cannot use localStorage to save user token, so i used \"nuxtServerInit\" API ,started local server and simulation localstorage through \"req.session\",.yes, it succeed. however, this req.session only save the current server, when people connect to other servers, this record has disappeared. so i want to know that how to achieve the purpose of stateless login\r\n\r\nlocal server\r\n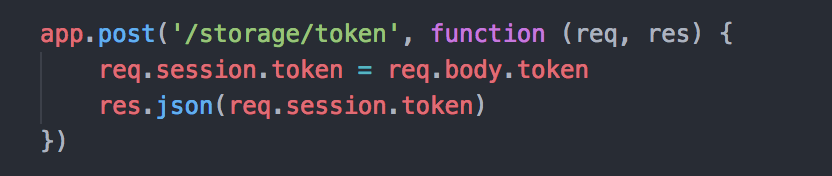\r\n\r\nnuxtServerInit\r\n\r\n\r\n\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1933\">#c1933\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2952,2955],{"name":2953,"color":2954},"question","cc317c",{"name":2942,"color":2943},2192,"how can i achieve the purpose of stateless login","2023-01-18T15:43:11Z","https://github.com/nuxt/nuxt/issues/2192",0.7394081,{"description":2962,"labels":2963,"number":2965,"owner":2903,"repository":2903,"state":2931,"title":2966,"updated_at":2967,"url":2968,"score":2969},"I have a survey with 5 different questions, the state of the questions is stored in a vuex store. These questions are used on different pages in the application. When I refresh the page the state is lost and gives an error.\r\n\r\nI have been looking at vuex-persistedstate, but it doesn't work together with nuxt as described in the issue below:\r\nhttps://github.com/robinvdvleuten/vuex-persistedstate/issues/16\r\n\r\nMy idea is to implement a local storage but unfortunately I did not succeed so far. I found this issue talking about local storage: https://github.com/nuxt/nuxt.js/issues/185 and it says we shouldn't use it. \r\n\r\nAre there any suggestions of keeping the vuex state on refresh without creating a session on server (no login). \n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c499\">#c499\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2964],{"name":2942,"color":2943},576,"Best impelentation localStorage","2023-01-18T15:39:31Z","https://github.com/nuxt/nuxt/issues/576",0.7422827,{"description":2971,"labels":2972,"number":2974,"owner":2903,"repository":2903,"state":2931,"title":2975,"updated_at":2976,"url":2977,"score":2978},"## Version\r\n\r\nv2.0.0\r\n\r\n## Github link\r\n\r\nhttps://github.com/StriveNuby/nuxt-admin\r\n\r\n## Purpose\r\n\r\nSwitching between each other can achieve data caching\r\n\r\n## Why is there no effect when using keep-alive?\r\n\r\n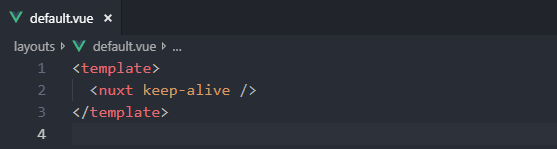\r\n\r\n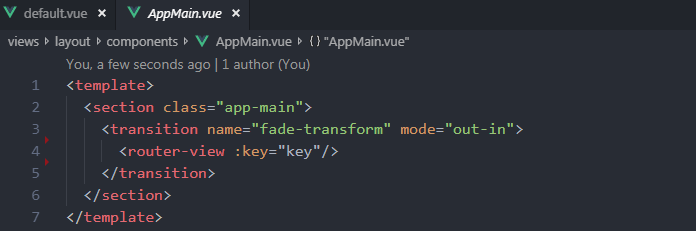\r\n\r\n### Screenshot in the project, Not implemented\r\n\r\n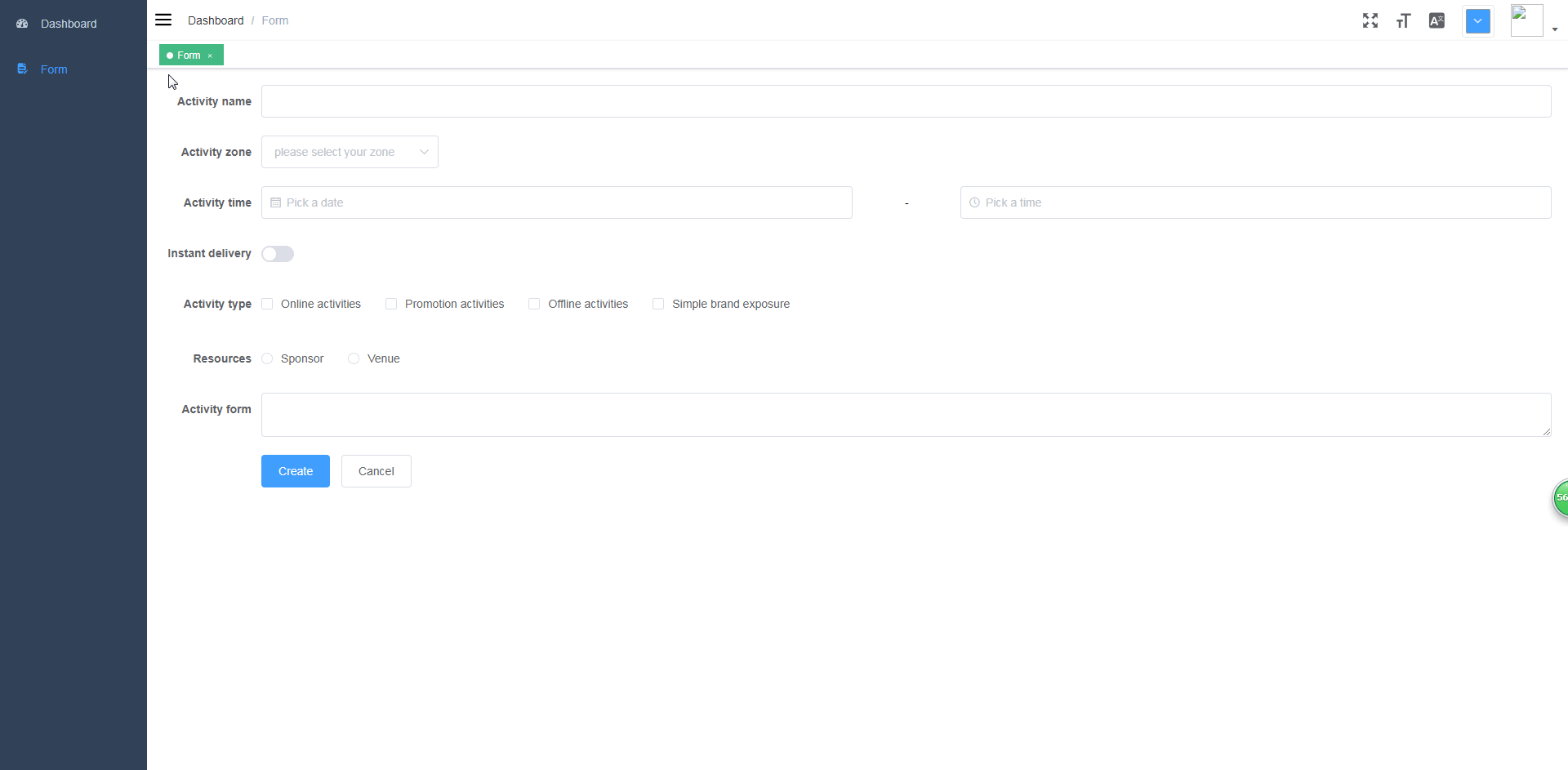\r\n\r\n\r\nOr does nuxtJs not support keep-alive? Is there any good idea?\r\n\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://cmty.app/nuxt\">Nuxt\u003C/a> community (\u003Ca href=\"https://cmty.app/nuxt/nuxt.js/issues/c7923\">#c7923\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2973],{"name":2942,"color":2943},4063,"Try to use keep-alive, can't make the desired effect, can you help?","2023-01-18T20:03:53Z","https://github.com/nuxt/nuxt/issues/4063",0.7427282,{"description":2980,"labels":2981,"number":2983,"owner":2903,"repository":2903,"state":2931,"title":2984,"updated_at":2985,"url":2986,"score":2987},"I am running some localStorage operations in store. Is there a way to dispatch this action similar to nuxtServerInit but on client-side instead? \n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1668\">#c1668\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2982],{"name":2942,"color":2943},1857,"client-side alternative to nuxtServerInit","2023-01-18T15:42:45Z","https://github.com/nuxt/nuxt/issues/1857",0.74317074,{"description":2989,"labels":2990,"number":2993,"owner":2903,"repository":2903,"state":2931,"title":2994,"updated_at":2995,"url":2996,"score":2997},"Is there any way to deal with localStorage, in order to set a property based on it for the entire application?\r\n\r\nLet's say I want to read a locally stored token to determine if a user has access to an API, and then set a boolean property on the layout, to be propagated to the relevant components, ie. navbar, login, logout, etc.\r\n\r\nsomething like this:\r\n\r\n```html\r\n\u003Ctemplate>\r\n \u003Cdiv>\r\n \u003Capp-nav :authed=\"authed\"/>\r\n \u003Cnuxt :authed=\"authed\"/>\r\n \u003C/div>\r\n\u003C/template>\r\n\r\n\u003Cscript>\r\n import AppNav from '~components/Nav.vue'\r\n\r\n export default {\r\n data: function () {\r\n return {\r\n authed: false\r\n }\r\n },\r\n\r\n components: {\r\n AppNav\r\n },\r\n\r\n created: () => {\r\n this.authed = localStorage.getItem('token') !== null\r\n }\r\n}\r\n\u003C/script>\r\n```\r\n\r\nThis example would generate an error ```localStorage is not defined```\r\n\r\nHow can I accomplish this?\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c157\">#c157\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[2991,2992],{"name":2953,"color":2954},{"name":2942,"color":2943},185,"read local storage on default layout?","2023-01-18T15:38:32Z","https://github.com/nuxt/nuxt/issues/185",0.7460859,{"description":2999,"labels":3000,"number":3002,"owner":2903,"repository":2903,"state":2931,"title":3003,"updated_at":3004,"url":3005,"score":3006},"Is this still completely impossible without using session or cookies?\r\n\r\nI'm working towards a quick generated site, with a very basic vuex store. I'd just like to persist some data across refreshes. I've tried hacking together a workaround by setting localstorage at the same time as setting the state, and then reading the state back from localstorage in a middleware, but obviously window not found is getting in the way.\r\n\r\nAny quick tips? If there are any it would be great to see the FAQ updated.\r\n\r\nNote - spa mode is not an option due to SEO concerns - which is the whole reason for using nuxt.\n\n\u003C!--cmty-->\u003C!--cmty_prevent_hook-->\n\u003Cdiv align=\"right\">\u003Csub>\u003Cem>This question is available on \u003Ca href=\"https://nuxtjs.cmty.io\">Nuxt.js\u003C/a> community (\u003Ca href=\"https://nuxtjs.cmty.io/nuxt/nuxt.js/issues/c1467\">#c1467\u003C/a>)\u003C/em>\u003C/sub>\u003C/div>",[3001],{"name":2942,"color":2943},1641,"Localstorage / persisting vuex","2023-01-18T15:42:07Z","https://github.com/nuxt/nuxt/issues/1641",0.7466988,["Reactive",3008],{},["Set"],["ShallowReactive",3011],{"$fTRc1wZytZ_XrK4EfJfei_Sz-An4H4Yy6syhVxH_PVJc":-1,"$f2FHP5BXTmtKZTp_B9c6oQmspyNe9FEfqIKqtyBfBkZE":-1},"/nuxt/icon/88"]